Python is a high-level, interpreted programming language known for its simplicity and readability. Created by Guido van Rossum and first released in 1991, Python’s clean syntax and versatile nature have made it a popular choice for beginners and professionals alike. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Python’s extensive standard library and active community contribute to its widespread use in web development, data science, automation, artificial intelligence, and more. Its cross-platform compatibility and ease of learning continue to drive its adoption across various industries.
What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. Created by Guido van Rossum and first released in 1991, Python has become one of the most popular programming languages in the world. Its design philosophy emphasizes code readability with the use of significant indentation, which makes it an ideal choice for both beginners and experienced developers.
Importance of Python in Modern Programming Python’s versatility has led to its widespread adoption in various domains, including web development, data science, machine learning, automation, and scientific computing. Its extensive standard library and the thriving community make it a go-to language for rapid development and prototyping. Python’s importance in modern programming cannot be overstated, as it continues to drive innovation across industries.
History of Python
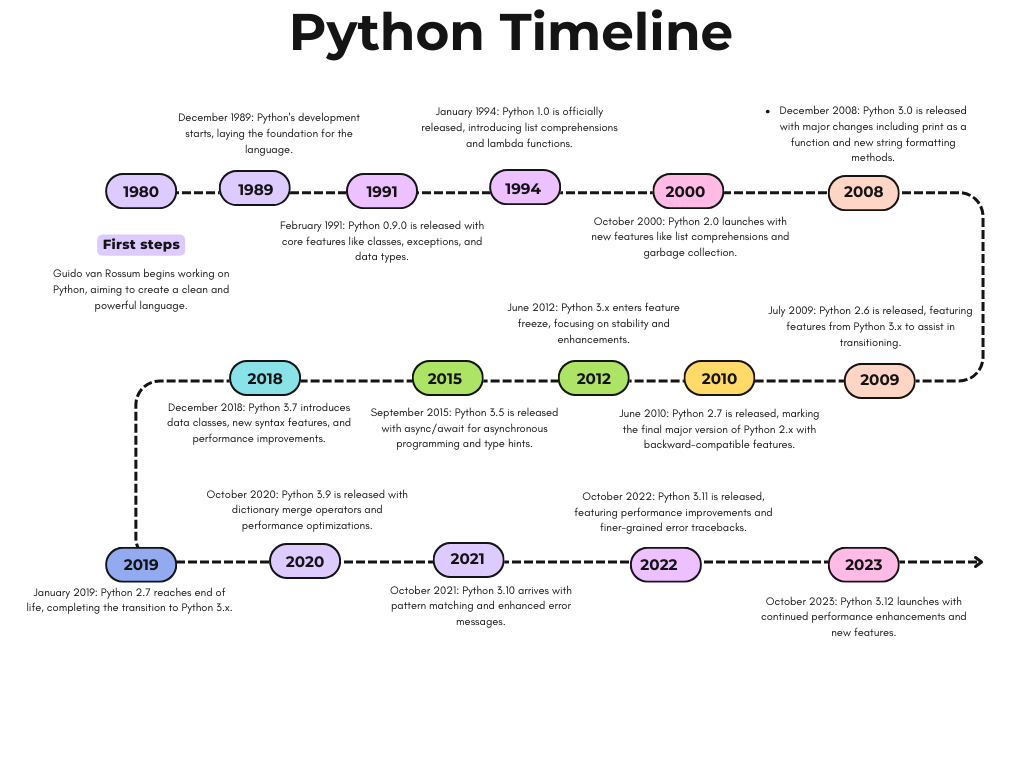
Origins and Development
Python’s story begins in the late 1980s, a period of significant technological advancement and experimentation in programming languages. The language was conceived as a successor to the ABC programming language, which was developed in the Netherlands at CWI (Centrum Wiskunde & Informatica). Guido van Rossum, a Dutch programmer, began working on Python in December 1989 during his Christmas holidays. His goal was to create a language that combined the best features of ABC with new functionalities and improvements.
Python was officially released to the public in February 1991 as version 0.9.0. This initial release included many features that are still present today, such as classes with inheritance, exception handling, functions, and the core data types like list, dictionary, and string. Python’s design was guided by a set of principles emphasizing simplicity, readability, and ease of learning, which have remained central to its evolution.
Python 1.0, the first official version, was released in January 1994. This version brought several enhancements, including new libraries and modules, and established Python as a practical language for various applications. The language quickly gained traction due to its clear syntax, dynamic typing, and extensibility.
Python 2.x Series
The introduction of Python 2.0 in October 2000 marked a significant milestone in the language’s development. Python 2.0 included many new features that improved functionality and usability, such as list comprehensions, garbage collection, and Unicode support. The release of Python 2.1 in April 2001 introduced iterators and a more powerful and flexible standard library, laying the foundation for future improvements.
The Python 2.x series continued to evolve with various updates and enhancements. Python 2.7, released in July 2010, was the final release in the Python 2.x series. It included improvements like the argparse
module for command-line parsing and various performance enhancements. Python 2.7 enjoyed a long period of support due to its widespread use, which allowed developers to transition to Python 3.x at their own pace.
Python 3.x Series
A pivotal moment in Python’s history was the release of Python 3.0 in December 2008. Python 3.0 was a major overhaul of the language, introducing several new features and making changes that were not backward compatible with Python 2.x. This decision aimed to address design flaws and inconsistencies in Python 2.x and to clean up the language by removing deprecated features.
Key improvements in Python 3.0 included:
- Enhanced Unicode Support: Python 3.x introduced a more consistent handling of Unicode, which made it easier to work with international text and encoding.
- Print Function: The
print
statement was replaced with theprint()
function, making it more consistent with other functions. - Division Operator: Integer division was changed to return a float by default, with the
//
operator introduced for integer division. - Iterators and Generators: Python 3.x improved the iterator protocol and introduced the
yield from
syntax to simplify generator composition. - Consistent Syntax: Python 3.x aimed to provide a more consistent and logical syntax, addressing many of the irregularities found in Python 2.x.
Despite these improvements, the transition from Python 2 to Python 3 was challenging for many projects due to the lack of backward compatibility. The Python community faced a split, with some developers continuing to use Python 2.x while others embraced Python 3.x.
To facilitate the transition, Python 2.7 was supported for a long time, receiving updates and fixes until its end-of-life in January 2020. The official discontinuation of Python 2.7 marked the end of support for Python 2.x and encouraged the community to adopt Python 3.x.
Current Status and Modern Developments
As of now, Python 3.x is the standard version in use, with ongoing development and regular releases that introduce new features and improvements. Python 3.9, released in October 2020, introduced features like dictionary merge operators and new string methods. Python 3.10, released in October 2021, brought structural pattern matching and more precise error messages.
Python 3.11, released in October 2022, focused on performance improvements and included features like exception groups and enhanced debugging support. The language continues to evolve, with Python 3.12 and beyond expected to bring further enhancements in performance, syntax, and functionality.
Python’s development is guided by the Python Enhancement Proposal (PEP) process, which allows community members to propose and discuss changes to the language. The Python Software Foundation (PSF) oversees the language’s development, ensuring that it remains relevant and robust.
Today, Python is widely used in various fields, including web development, data science, machine learning, automation, and more. Its growth and success are a testament to its strong community, clear design principles, and adaptability to modern programming needs.
Python’s Core Features
Python has gained immense popularity among developers and data scientists due to its core features that simplify programming and enhance productivity. Below, we delve into some of these core features, including modern additions that further bolster Python’s capabilities.
Simplicity and Readability
Python’s syntax is designed with simplicity and readability in mind, making it accessible to both novice and experienced developers. This design philosophy is evident in several key aspects:
- Indentation-Based Syntax: Python uses indentation to define code blocks, replacing the need for curly braces or explicit block delimiters. This approach promotes clean and readable code, as it visually represents the structure of the code. It reduces syntactical noise and makes the code easier to follow and maintain.
- Concise Syntax: Python’s syntax allows developers to express complex ideas in fewer lines of code. For instance, list comprehensions and generator expressions provide a concise way to create and manipulate lists. This brevity not only speeds up development but also reduces the likelihood of errors and improves code readability.
- Readability Emphasis: Python follows the principle of “There should be one—and preferably only one—obvious way to do it,” which encourages writing code that is clear and straightforward. This philosophy is reflected in Python’s design decisions and its emphasis on code clarity and simplicity.
Extensive Standard Library
Python’s extensive standard library is one of its most notable features, providing a wide range of modules and packages that facilitate various programming tasks:
- Rich Module Collection: The standard library includes modules for file I/O, system calls, networking, and internet protocols, among others. This rich collection reduces the need for external libraries, as many common tasks can be accomplished using built-in modules.
- Web Development: Python’s standard library includes modules like
http.server
andcgi
for basic web server functionalities. For more advanced web development, frameworks such as Django and Flask are available, but the standard library’s offerings provide foundational tools for building web applications. - Data Manipulation: Modules like
csv
,json
, andsqlite3
are part of the standard library, enabling developers to handle data formats and interact with databases efficiently. These modules facilitate tasks such as parsing CSV files, working with JSON data, and executing SQL queries. - Modern Enhancements: Recent Python versions have introduced new modules and enhancements to the standard library. For example, the
dataclasses
module, introduced in Python 3.7, provides a decorator and functions for creating data classes, which simplify the definition of classes used primarily for storing data.
Cross-Platform Compatibility
Python’s cross-platform compatibility is a significant advantage, allowing code to run on various operating systems with minimal modifications:
- Multi-OS Support: Python is designed to be platform-independent, meaning that code written on one operating system (e.g., Windows) can typically run on others (e.g., macOS, Linux) without changes. This feature is particularly beneficial for developing applications that need to operate across different environments.
- Virtual Environments: To further support cross-platform development, Python offers tools like
venv
andvirtualenv
for creating isolated environments. These tools ensure that dependencies are consistent and isolated from the system’s global Python installation, reducing conflicts and improving portability. - Platform-Specific Modules: While Python aims for cross-platform compatibility, it also includes modules that provide platform-specific functionalities. For example, the
platform
module allows developers to access information about the operating system and hardware, enabling them to write code that adapts to the specific platform if necessary.
Modern Features
Python continues to evolve, incorporating modern features that enhance its functionality and usability:
- Asynchronous Programming: Python introduced
asyncio
and theasync
/await
syntax in Python 3.5 and later, providing support for asynchronous programming. This feature allows developers to write concurrent code more easily and efficiently, improving performance for I/O-bound and high-level structured network code. - Type Hinting: Type hinting, introduced in Python 3.5 with PEP 484, allows developers to specify the types of variables and function return values. This feature enhances code readability and helps tools like linters and IDEs catch potential errors before runtime.
- Pattern Matching: Python 3.10 introduced structural pattern matching, allowing developers to match patterns in data structures more intuitively. This feature simplifies complex conditional logic and enhances code clarity.
- F-Strings: Python 3.6 introduced f-strings (formatted string literals), which provide a concise and efficient way to embed expressions inside string literals. F-strings improve readability and performance compared to older string formatting methods.
Python’s core features—simplicity and readability, extensive standard library, and cross-platform compatibility—coupled with modern enhancements, make it a powerful and versatile language for a wide range of applications. Whether you’re developing web applications, performing data analysis, or working with machine learning, Python’s robust capabilities and evolving features ensure it remains a top choice for developers around the world.
Python Syntax and Structure
Variables
In Python, variables are dynamically typed and do not require explicit declaration before use. Memory allocation occurs automatically when a value is assigned to a variable. This dynamic typing allows for more flexible coding practices, as variables can be reassigned to different data types as needed.

Data Types
Python supports a variety of built-in data types, each with specific characteristics and use cases:
- Integers: Whole numbers without fractional parts. Python supports arbitrarily large integers, which means they are only limited by the available memory.
- Floats: Floating-point numbers represent real numbers and include a decimal point. Python uses double-precision floating-point numbers.
- Strings: Strings are sequences of characters enclosed in single quotes (
'
), double quotes ("
), or triple quotes ('''
or"""
). Strings are immutable, meaning they cannot be changed after creation. - Lists: Lists are ordered collections that can hold items of different types. Lists are mutable, allowing elements to be modified, added, or removed.
- Tuples: Tuples are similar to lists but are immutable. Once created, the contents of a tuple cannot be altered.
- Dictionaries: Dictionaries are unordered collections of key-value pairs. Keys must be unique and immutable, while values can be of any type.
Functions
Function Definition
Functions in Python are defined using the def
keyword. Functions are first-class objects, meaning they can be assigned to variables, passed as arguments, and returned from other functions. This flexibility supports higher-order programming and functional programming techniques.

Function Calls
Functions are called by using their name followed by parentheses. Any required arguments are placed within the parentheses.

Higher-Order Functions
Higher-order functions can take other functions as arguments or return them. This feature is useful for implementing functional programming patterns, such as decorators and callbacks.

Built-In Functions
Python includes a rich set of built-in functions that perform common tasks, such as len()
for determining the length of an object, max()
for finding the maximum value, and sorted()
for sorting iterable objects.
Control Flow
Conditionals
Control flow in Python is managed using if
, elif
, and else
statements. These statements allow branching logic based on conditions. The use of indentation is crucial, as it defines the scope of these control structures.
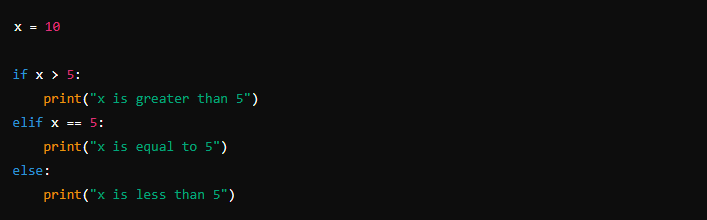
Loops
Python provides two primary loop constructs for iteration:
- For Loops: Used to iterate over sequences such as lists, tuples, or strings. The
for
loop can also iterate over ranges of numbers using therange()
function. - While Loops: Continue executing as long as a specified condition is true. This loop is useful for cases where the number of iterations is not known in advance.
Exceptions
Exception handling in Python is performed using try
, except
, else
, and finally
blocks. This mechanism allows for graceful error handling and resource management.
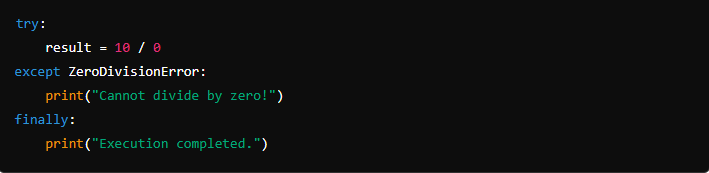
Object-Oriented Programming (OOP)
Classes and Objects
Python supports object-oriented programming, which is centered around the concepts of classes and objects. Classes define the blueprint for objects, encapsulating data and behavior. Objects are instances of classes.
Creating Objects
Objects are instantiated by calling the class name followed by parentheses. Initialization parameters are passed to the class constructor.
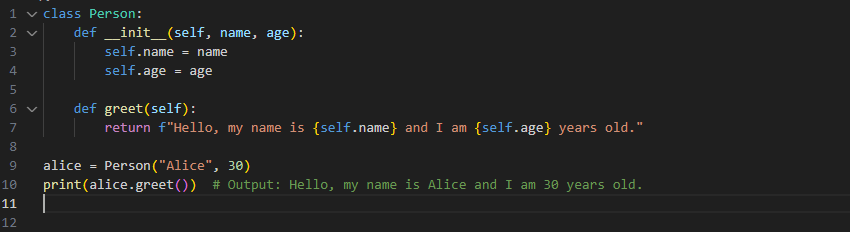
Python for Web Development
Python is widely used in web development, thanks to its powerful frameworks that cater to different needs and preferences. Here’s an overview of some prominent Python web frameworks:
1. Django
Django is a high-level framework that encourages rapid development and clean, pragmatic design. It comes with a wealth of built-in features, including an ORM, authentication, and an admin panel. This framework is known for its “batteries-included” philosophy, providing a comprehensive set of tools and libraries out of the box.
2. Flask
Flask is a micro-framework that provides the essential tools needed to build a web application while giving developers more control over their projects. It’s lightweight and flexible, making it ideal for small to medium-sized applications and for those who prefer to add components as needed.
3. FastAPI
FastAPI is a modern, high-performance framework for building APIs with Python 3.6+ based on standard Python type hints. It’s designed to create robust and scalable APIs quickly, with support for asynchronous programming. FastAPI emphasizes speed, ease of use, and automatic generation of interactive API documentation.
4. Falcon
Falcon is a minimalist web framework designed for building high-performance APIs. It focuses on being lightweight and highly efficient, making it suitable for large-scale applications that need to handle a lot of requests with minimal overhead. Falcon is often chosen for its speed and ability to handle complex workloads.
5. CherryPy
CherryPy is one of the oldest Python web frameworks, known for its simplicity and ease of use. It provides a simple interface for building web applications with built-in tools for handling HTTP requests, sessions, and more. CherryPy is suitable for both small and large-scale applications and can be used to create production-ready applications quickly.
Advantages of Python for Web Development
Python’s simplicity and readability make it an excellent choice for web development. Its diverse range of frameworks, like Django, Flask, FastAPI, Falcon, and CherryPy, allow developers to choose the right tool for their specific needs, from rapid development to high-performance applications. Additionally, Python’s support for asynchronous operations and extensive libraries make it well-suited for developing robust and scalable web applications.
Python for Data Science
Python has emerged as a leading language in the field of data science, thanks to its powerful and flexible libraries. These libraries provide essential tools for data manipulation, numerical computations, and data visualization, making Python an indispensable tool for data professionals.
1. Pandas
Pandas is a fundamental library for data analysis in Python. It introduces two primary data structures: Series and DataFrames. Series is a one-dimensional array-like structure, while DataFrames are two-dimensional, tabular data structures similar to spreadsheets or SQL tables. Pandas excels in handling and manipulating data through its powerful indexing, alignment, and reshaping capabilities. It supports various file formats, including CSV, Excel, and SQL databases, making data ingestion and preprocessing straightforward. Key features include data cleaning operations like filling missing values, merging datasets, and group-by functionalities, which are essential for preparing data for analysis.
2. NumPy
NumPy, short for Numerical Python, provides support for high-performance operations on large, multi-dimensional arrays and matrices. Its core data structure, the ndarray, allows for efficient storage and manipulation of numerical data. NumPy includes a comprehensive set of mathematical functions to perform operations such as linear algebra, statistical computations, and Fourier transforms. It is the backbone of many other scientific computing libraries and is crucial for performing numerical operations that require speed and efficiency.
3. Matplotlib
Matplotlib is a plotting library that allows for the creation of a wide variety of static, animated, and interactive visualizations. It offers comprehensive control over plot aesthetics, including colors, styles, and layouts, enabling the creation of publication-quality charts. Matplotlib’s integration with other libraries like Pandas and NumPy makes it easy to visualize data directly from DataFrames and arrays. Common visualizations include line plots, bar charts, histograms, scatter plots, and heatmaps, which help in exploring and presenting data trends and patterns effectively.
Use Cases in Data Analysis
Python’s data science libraries are applied across numerous fields to derive insights from complex datasets. In finance, these tools are used for algorithmic trading, risk management, and portfolio optimization. In healthcare, they assist in analyzing patient data, predicting disease outbreaks, and personalizing treatment plans. Marketing professionals use Python to analyze consumer behavior, optimize advertising campaigns, and forecast sales trends. The ease of use, combined with Python’s extensive ecosystem, enables data scientists to perform robust analyses, build predictive models, and generate meaningful visualizations that support data-driven decision-making.
Python in Machine Learning
Machine learning (ML) is a branch of artificial intelligence that focuses on developing algorithms and models that allow computers to learn from and make decisions based on data. Unlike traditional programming where explicit instructions are given to the computer, machine learning models learn patterns and insights from data, making predictions or decisions without being explicitly programmed for specific tasks. This capability to adapt and improve over time makes ML highly valuable in a variety of applications, from recommendation systems to autonomous vehicles.
Python has emerged as the dominant language for machine learning due to its simplicity, readability, and the robust ecosystem of libraries that support various aspects of ML. Its straightforward syntax makes it accessible to both beginners and experienced practitioners, while its extensive libraries provide powerful tools for developing and deploying machine learning models.
Key Libraries (TensorFlow, Scikit-learn)
Python’s machine learning ecosystem is supported by a range of libraries, each offering unique capabilities and advantages. Two of the most prominent libraries are TensorFlow and Scikit-learn.
1. TensorFlow
TensorFlow is an open-source library developed by Google, designed for high-performance numerical computation and deep learning. It provides a comprehensive, flexible ecosystem for developing and deploying machine learning models, particularly those involving deep neural networks. TensorFlow’s core component is its data flow graph, which allows users to build complex models by defining a graph of computations. This graph-based approach facilitates optimization and scalability, making TensorFlow suitable for both research and production environments.
One of TensorFlow’s key features is its support for GPU acceleration, which significantly speeds up training and inference processes. Additionally, TensorFlow offers a high-level API called Keras, which simplifies the process of building and training neural networks with its user-friendly interface. TensorFlow is widely used in applications such as image and speech recognition, natural language processing, and reinforcement learning.
2. Scikit-learn
Scikit-learn is a versatile library for classical machine learning algorithms and data analysis. It provides simple and efficient tools for data mining and data analysis, built on top of NumPy, SciPy, and Matplotlib. Scikit-learn is known for its ease of use and comprehensive documentation, making it a popular choice for beginners and experienced practitioners alike.
The library includes a wide range of algorithms for classification, regression, clustering, and dimensionality reduction. It also offers utilities for model selection, preprocessing, and evaluation, such as cross-validation and grid search. Scikit-learn is particularly valued for its ability to integrate seamlessly with other Python libraries and for its straightforward API, which allows users to apply machine learning techniques with minimal code.
Other Notable Libraries
In addition to TensorFlow and Scikit-learn, there are other notable libraries that contribute to Python’s dominance in machine learning:
- Keras: Originally developed as a high-level API for TensorFlow, Keras now supports multiple backends and is known for its simplicity and ease of use in building and training neural networks.
- PyTorch: Developed by Facebook’s AI Research lab, PyTorch is a dynamic computational graph library that is widely used for deep learning research and applications. It offers flexible and intuitive tools for model building and training.
- XGBoost: An optimized gradient boosting library designed for performance and efficiency, XGBoost is often used in competitive machine learning and data science challenges.
Python’s extensive machine learning libraries, combined with its simplicity and flexibility, make it a powerful tool for developing innovative and effective machine learning models. Whether you are working on deep learning projects, classical machine learning tasks, or data analysis, Python provides the tools and resources needed to tackle complex challenges and drive advancements in the field.
Python for Automation
Python’s robust capabilities and user-friendly syntax make it a prime choice for automation across various domains. From simple scripting to complex system integrations, Python’s flexibility and extensive library ecosystem facilitate a wide range of automation tasks.
Scripting and Task Automation
File Management
Python’s built-in libraries, such as os
and shutil
, provide comprehensive tools for managing files and directories. These libraries enable developers to perform operations like renaming files, copying or moving files, and traversing directories. For example, the os
module can be used to list files in a directory, while shutil
can handle file copying and deletion.
Web Scraping
Web scraping involves extracting data from websites. Python’s BeautifulSoup
and requests
libraries are commonly used for this purpose. requests
handles HTTP requests, while BeautifulSoup
parses HTML and XML documents to extract the necessary data.
Data Entry and Processing
Python’s ability to handle data processing tasks is facilitated by libraries like pandas
, which is essential for automating data entry, cleaning, and transformation tasks. Automation scripts can read from various data sources (CSV, Excel, databases) and process the data as needed.
API Interactions
Python’s requests
library is also instrumental in automating interactions with web APIs. Developers can use it to send HTTP requests, handle responses, and integrate with various web services.
Controlling Hardware
For more advanced automation tasks, such as controlling hardware or interfacing with external devices, Python offers libraries like pyserial
for serial communication and RPi.GPIO
for Raspberry Pi GPIO pin control. These libraries enable automation scripts to interact with physical components.
Popular Automation Libraries
Selenium
Selenium is a powerful tool for automating web browsers. It can simulate user interactions, such as clicking buttons, filling out forms, and navigating websites. Selenium supports multiple browsers and is widely used for automated testing of web applications.
PyAutoGUI
PyAutoGUI provides functions for controlling the mouse and keyboard programmatically. This library can automate GUI interactions, such as clicking buttons and typing text, making it useful for automating repetitive tasks in desktop applications.
Other Notable Libraries
schedule
: A lightweight library for scheduling tasks to run at specific intervals. It is useful for setting up recurring automation tasks.paramiko
: A library for SSH protocol implementation, which allows for secure connections to remote servers and execution of commands remotely.pytesseract
: A Python wrapper for Google’s Tesseract-OCR Engine, which is used for optical character recognition to extract text from images.
Python’s capabilities for automation are vast and continue to expand with its ever-growing ecosystem. The combination of easy-to-read syntax, powerful libraries, and versatile features makes Python an ideal language for automating a wide array of tasks, from simple file operations to complex system integrations.
Python in Scientific Computing
Libraries (SciPy, SymPy) Python is widely used in scientific computing, thanks to libraries like SciPy and SymPy. SciPy is a library used for scientific and technical computing, providing modules for optimization, integration, interpolation, and more. SymPy is a symbolic mathematics library that allows for algebraic manipulation, calculus, and equation solving.
Applications in Research and Development Python’s scientific libraries are used in various research fields, including physics, chemistry, biology, and engineering. Researchers use Python to simulate complex systems, analyze experimental data, and develop new algorithms. Python’s open-source nature and extensive ecosystem make it a popular choice in the scientific community.
Python for Game Development
Libraries and Frameworks (Pygame, Panda3D) Python is also used in game development, particularly for prototyping and educational purposes. Pygame is a popular library that simplifies the creation of 2D games, while Panda3D is a framework for developing 3D games. These tools allow developers to create interactive games with minimal code.
Examples of Games Developed with Python Several games have been developed using Python, particularly indie and educational games. For example, the game “Frets on Fire” was developed using Python and Pygame. Python’s simplicity and readability make it an excellent choice for game development, especially for beginners.
Python for GUI Development
Libraries (Tkinter, PyQt) Python provides several libraries for creating graphical user interfaces (GUIs), with Tkinter and PyQt being the most popular. Tkinter is included with Python’s standard library and provides a simple way to create GUIs, while PyQt offers more advanced features and is used in professional applications.
Advantages and Limitations Python’s GUI libraries allow developers to create cross-platform applications with native-looking interfaces. However, Python’s performance limitations can be a drawback for more complex applications that require high responsiveness.
Python for Networking
Python’s versatility extends into networking, where it is employed to create, manage, and interact with networked applications and systems. Its broad array of libraries and frameworks provide tools for both low-level and high-level networking tasks, making Python a powerful choice for network programming.
Libraries for Networking
Socket Library
The socket
module is part of Python’s standard library and provides a fundamental interface for network communication. It enables developers to create network connections using the TCP/IP protocol and handle data transmission between systems. Key functionalities of the socket
library include:
- Creating a Socket: A socket is an endpoint for communication. The
socket
module allows you to create sockets that use various communication protocols, such as TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). - Binding and Listening: For server-side applications, you can bind a socket to a specific address and port, and then listen for incoming connections. This is typically used for developing web servers and other network services.
- Sending and Receiving Data: Once a connection is established, data can be sent and received through the socket. The
send
andrecv
methods facilitate data transfer between the client and server.
Twisted Framework
Twisted is an asynchronous networking framework that provides a higher-level interface for building networked applications. It is designed for scalability and supports various protocols and services. Twisted is particularly useful for developing applications that require handling multiple simultaneous connections efficiently.
- Asynchronous Networking: Twisted uses an event-driven architecture, allowing it to manage multiple connections without blocking. This is crucial for building scalable network servers and clients.
- Protocol Implementation: Twisted supports a wide range of network protocols, including HTTP, FTP, and IMAP. Developers can implement custom protocols or use built-in ones for various networking tasks.
Applications in Network Programming
Developing Web Servers
Python’s libraries and frameworks, including socket
and Twisted, are used to build web servers that handle HTTP requests. The socket
module provides the basic building blocks for creating HTTP servers, while frameworks like Twisted offer more advanced features and support for concurrent connections.
Creating Chat Applications
Building real-time chat applications is a common use case for Python networking. Using socket
for basic implementations or Twisted for more complex applications, developers can create chat systems that handle multiple users and provide functionalities like message broadcasting and user management.
Implementing Protocols
Python’s networking libraries allow developers to implement custom network protocols or work with existing ones. For instance, Twisted’s protocol framework supports the creation of custom protocols for specific applications, while the socket
module can be used to develop simpler implementations.
Network Testing and Automation
Python is often used for network testing and automation tasks, such as network monitoring, performance testing, and automated configuration. Libraries like scapy
and paramiko
(for SSH) complement Python’s networking capabilities, enabling developers to create tools that test and automate network interactions.
Network Security
Python is also used for network security tasks, including vulnerability scanning, penetration testing, and network traffic analysis. Libraries like nmap
(for network scanning) and cryptography
(for secure communications) are often utilized in security-related applications.
Python’s networking capabilities are extensive and diverse, supporting a wide range of applications from simple network interactions to complex, scalable network services. Its ease of use, combined with powerful libraries and frameworks, makes it a preferred choice for network programming and automation.
Python for Embedded Systems
MicroPython and CircuitPython MicroPython and CircuitPython are lightweight versions of Python designed for microcontrollers. They allow developers to write Python code that runs on embedded systems, such as the ESP8266 and Raspberry Pi.
Applications in IoT Python is increasingly being used in the Internet of Things (IoT) for developing applications that run on embedded devices. Its simplicity and extensive library support make it an ideal choice for IoT development, particularly for prototyping.
Python in Academia and Education
Python as a Teaching Tool Python is widely used as a teaching tool in schools and universities due to its simplicity and readability. It is often the first programming language taught to students, as it allows them to focus on learning programming concepts without getting bogged down by complex syntax.
Python’s Role in Research In academia, Python is used in research for data analysis, simulations, and developing new algorithms. Its extensive library support and open-source nature make it a preferred choice for researchers across various disciplines.
Community and Ecosystem
Python Package Index (PyPI) The Python Package Index (PyPI) is the official repository for third-party Python libraries. It hosts thousands of packages that extend Python’s functionality in areas like web development, data science, and machine learning.
Community Support and Documentation Python’s large and active community provides extensive support and documentation, making it easy for developers to find solutions to problems and stay updated with the latest developments. The community also contributes to the continuous improvement of Python through open-source projects.
Challenges and Limitations of Python
Python, celebrated for its readability, ease of use, and versatility, is one of the most popular programming languages today. However, despite its many advantages, Python has several challenges and limitations that developers must consider. Understanding these limitations is crucial for effectively leveraging Python in various contexts and making informed decisions about its use.
1. Performance and Speed
Interpreted Language: Python is an interpreted language, meaning that code is executed line-by-line at runtime. This interpretation process can lead to slower execution times compared to compiled languages like C or C++. The dynamic nature of Python also contributes to this overhead, as type checking and other operations are performed at runtime.
Global Interpreter Lock (GIL): The Global Interpreter Lock (GIL) is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes simultaneously. While this simplifies memory management and avoids issues with concurrent access, it also limits Python’s performance in multi-threaded applications. This can be a significant drawback for CPU-bound tasks that require parallel execution.
Workarounds: To mitigate these performance issues, developers often use optimization techniques or alternative implementations of Python. For example, the PyPy
interpreter is designed to improve execution speed through Just-In-Time (JIT) compilation. Additionally, using extensions written in C or C++ (such as Cython
or Numba
) can help accelerate performance-critical sections of Python code.
2. Memory Consumption
High Memory Usage: Python’s memory management, while convenient, can lead to higher memory consumption compared to lower-level languages. The language’s dynamic typing, automatic memory management, and garbage collection contribute to this overhead. This can be particularly problematic in memory-constrained environments or for applications with large datasets.
Garbage Collection: Python uses a garbage collector to manage memory, which automatically reclaims memory that is no longer in use. While this simplifies memory management, it can introduce unpredictability in memory usage and performance, as the garbage collector runs at arbitrary times and can cause performance hiccups.
Strategies: To address memory issues, developers can use profiling tools to identify memory hotspots and optimize data structures. Libraries like memory_profiler
and objgraph
can help track and manage memory usage more effectively.
3. Concurrency and Parallelism
Concurrency Limitations: Python’s GIL and interpreted nature pose challenges for concurrent programming. While Python supports concurrency through threading and asynchronous programming (using asyncio
), it struggles with parallelism due to the GIL. This means that multi-threaded Python programs may not fully utilize multi-core processors for CPU-bound tasks.
Asynchronous Programming: Asynchronous programming in Python, while powerful, introduces complexity. The asyncio
library allows for non-blocking I/O operations, but developers must understand the nuances of async/await syntax and manage event loops. This can create a learning curve and potential pitfalls in program design.
Parallel Processing: For parallel processing, developers often use multiprocessing or external libraries like concurrent.futures
. The multiprocessing module creates separate memory spaces for processes, bypassing the GIL but incurring overhead due to inter-process communication.
4. Mobile and Web Development
Limited Mobile Support: Python is not commonly used for mobile app development. While there are frameworks like Kivy and BeeWare that allow Python to be used for mobile apps, these are not as mature or widely adopted as native development tools for iOS (Swift/Objective-C) and Android (Java/Kotlin). This can lead to limited performance and fewer resources for mobile development.
Web Development Challenges: In web development, Python frameworks like Django and Flask are widely used, but they face stiff competition from other languages and frameworks, such as JavaScript with Node.js. Python’s web frameworks can be less performant compared to solutions optimized for real-time and high-traffic web applications.
5. Dynamic Typing and Error Handling
Dynamic Typing: Python’s dynamic typing allows for rapid development and flexibility but can also lead to runtime errors that are only detected when the code is executed. This can result in bugs that are difficult to trace and fix, especially in large codebases.
Lack of Type Checking: While Python supports type hints (introduced in Python 3.5), the language does not enforce type checking at runtime. This means that type errors are only caught during development or through optional static analysis tools like mypy
. Developers need to be diligent about writing tests and using type hints to mitigate these issues.
Error Handling: Python’s error handling model relies on exceptions, which can be caught and handled using try
/except
blocks. While this provides flexibility, it can also lead to verbose and potentially error-prone code if not used carefully.
6. Dependency Management
Package Management: Python’s ecosystem includes a vast number of third-party libraries and packages, which can be both an advantage and a challenge. Managing dependencies and ensuring compatibility between packages can be complex, especially in larger projects.
Virtual Environments: Python developers often use virtual environments to isolate dependencies for different projects. Tools like venv
and virtualenv
help manage these environments, but maintaining them and ensuring consistency across development, testing, and production environments can still be challenging.
Package Conflicts: Dependency conflicts, where different packages require incompatible versions of the same dependency, can cause issues. Tools like pip
and pipenv
aim to address this by managing and resolving dependencies, but conflicts can still arise.
7. Community and Ecosystem
Fragmented Ecosystem: While Python’s large and active community is a strength, it can also lead to fragmentation. Multiple libraries and frameworks often offer similar functionalities, and choosing the right one for a specific task can be challenging. Additionally, some libraries may become outdated or unsupported over time.
Documentation Quality: The quality and completeness of documentation can vary between Python libraries and frameworks. While many libraries are well-documented, others may have incomplete or outdated documentation, making it harder for developers to use and troubleshoot them effectively.
8. Integration with Other Languages
Interoperability Issues: Python often needs to interface with other programming languages, such as C/C++, Java, or .NET. While tools and libraries exist for integration (e.g., ctypes
, SWIG
, Jython
), these can introduce complexity and performance overhead.
Performance Considerations: Interfacing Python with lower-level languages for performance reasons can be challenging. Bridging between Python and languages like C/C++ often involves writing additional code and managing data conversions, which can impact performance and maintainability.
Expert Insights or Case Studies
Python, a versatile and powerful language, has garnered significant attention from experts and professionals across various industries. Its applications range from web development to data science, machine learning, automation, and beyond. This article delves into expert insights and case studies showcasing Python’s impact and practical applications in diverse fields.
1. Expert Insights into Python’s Evolution and Adoption
Guido van Rossum’s Vision
Guido van Rossum, Python’s creator, envisioned a language that prioritized simplicity and readability. He designed Python to be intuitive and easy to learn, with a focus on clear and concise syntax. This vision has driven Python’s evolution, making it a go-to language for both beginners and experienced developers.
Key Points:
- Readability and Simplicity: Python’s syntax emphasizes readability, which lowers the learning curve for new programmers and improves maintainability for existing code.
- Versatility: Python’s design allows it to be applied in various domains, from web development to scientific computing.
- Community and Growth: The Python community has played a crucial role in its success, contributing to a rich ecosystem of libraries and frameworks.
Python in Industry
1. Data Science and Analytics:
Expert Opinion: Dr. Wes McKinney, the creator of the Pandas library, highlights Python’s role in transforming data science. His work emphasizes Python’s ability to handle and analyze large datasets efficiently.
Key Insights:
- Pandas: Revolutionized data manipulation with its DataFrame structure, enabling complex data analyses with ease.
- Scikit-learn: Provides robust tools for machine learning, making it accessible for data scientists to build and deploy models.
2. Machine Learning and AI:
Expert Opinion: Andrew Ng, a prominent figure in AI and machine learning, has recognized Python’s importance in the field. Python’s libraries such as TensorFlow and PyTorch are pivotal in developing sophisticated machine learning models.
Key Insights:
- TensorFlow and PyTorch: Facilitate deep learning and neural network construction, with extensive support for building scalable AI models.
- Jupyter Notebooks: Enable interactive development and visualization, making experimentation with machine learning models more accessible.
3. Web Development:
Expert Opinion: Armin Ronacher, the creator of Flask, underscores Python’s impact on web development. His work highlights Python’s ability to support both simple and complex web applications.
Key Insights:
- Django: Offers a full-stack framework with built-in features like authentication, ORM, and an admin panel.
- Flask: Provides flexibility and control, allowing developers to build lightweight and modular web applications.
2. Case Studies Showcasing Python’s Impact
Case Study 1: Instagram – Scaling with Django
Background: Instagram, a leading social media platform, initially built its backend using Django, a Python web framework. The company faced rapid growth and needed a scalable solution to handle a large volume of user interactions.
Challenges:
- Scalability: Managing and scaling infrastructure to handle millions of users and their data.
- Performance: Ensuring that the application remains responsive and efficient under high traffic.
Solution: Instagram leveraged Django’s robust features, such as its ORM and admin interface, to build and scale their application. Django’s modular architecture allowed Instagram to add new features quickly and efficiently.
Results:
- Scalability: Django’s design allowed Instagram to handle a massive user base while maintaining performance.
- Development Speed: Django’s built-in tools accelerated the development process, enabling rapid deployment of new features.
Lessons Learned:
- Framework Choice: Django’s comprehensive features and scalability made it a suitable choice for Instagram’s needs.
- Modularity: The modular nature of Django facilitated ongoing development and integration of new features.
Case Study 2: Spotify – Leveraging Python for Data Processing
Background: Spotify, a leading music streaming service, uses Python extensively for data processing and analysis. The company processes vast amounts of data related to user listening habits, recommendations, and playlists.
Challenges:
- Data Volume: Handling and analyzing large datasets efficiently.
- Real-Time Processing: Providing real-time recommendations and analytics to enhance user experience.
Solution: Spotify employs Python alongside other technologies to manage and analyze data. Python libraries like Pandas and NumPy are used for data manipulation, while Apache Spark is used for distributed data processing.
Results:
- Efficiency: Python’s libraries enabled efficient data manipulation and analysis.
- Scalability: Integration with Apache Spark facilitated handling large datasets and real-time processing.
Lessons Learned:
- Library Utilization: Python’s ecosystem of libraries supports complex data processing tasks effectively.
- Integration: Combining Python with other technologies can address performance and scalability needs.
Case Study 3: NASA – Python in Space Missions
Background: NASA uses Python for various tasks, including data analysis, simulation, and automation in space missions. Python’s simplicity and extensive libraries make it suitable for scientific computing and mission-critical applications.
Challenges:
- Complexity: Managing and analyzing complex scientific data from space missions.
- Reliability: Ensuring the accuracy and reliability of mission-critical software.
Solution: NASA employs Python’s scientific libraries like SciPy and NumPy for data analysis and simulations. Python scripts are also used for automating repetitive tasks and processing data from instruments.
Results:
- Accuracy: Python’s libraries provided reliable tools for scientific analysis and simulation.
- Efficiency: Automation scripts streamlined processes and improved operational efficiency.
Lessons Learned:
- Scientific Libraries: Python’s scientific libraries offer powerful tools for complex calculations and data analysis.
- Automation: Python’s scripting capabilities enhance efficiency and accuracy in mission operations.
Case Study 4: Dropbox – Python for Backend Infrastructure
Background: Dropbox, a cloud storage and file-sharing service, uses Python extensively for backend development. The company needed a reliable and scalable solution to handle its growing user base and file storage requirements.
Challenges:
- Scalability: Managing a large-scale file storage system with millions of users.
- Performance: Ensuring that file operations are fast and reliable.
Solution: Dropbox utilized Python for its backend infrastructure, leveraging its ease of use and rapid development capabilities. The company also used Python’s C extension capabilities to optimize performance-critical components.
Results:
- Scalability: Python’s flexibility and ease of integration with other technologies supported Dropbox’s growth.
- Performance: Optimizations and extensions improved the performance of critical backend components.
Lessons Learned:
- Integration: Python’s ability to integrate with lower-level languages enhances performance.
- Development Speed: Python’s simplicity accelerated development and deployment processes.
Future of Python
Python, a language known for its simplicity and versatility, has had a profound impact on the tech industry since its inception. As we look toward the future, several emerging trends and evolving roles are set to shape Python’s journey, ensuring its continued relevance and importance in the tech landscape. Here’s a detailed exploration of these aspects:
Emerging Trends in Python
1. Expansion of Libraries and Frameworks
One of Python’s greatest strengths is its rich ecosystem of libraries and frameworks. As new technologies emerge and the needs of developers evolve, Python continues to expand its repertoire. For instance, in the field of data science, libraries like Pandas and NumPy have been foundational, but newer libraries like Polars and Vaex are emerging to handle big data with better performance. In machine learning, frameworks like TensorFlow and PyTorch have set benchmarks, but new tools are constantly being developed to simplify workflows and improve efficiency.
The rise of specialized libraries, such as Dask for parallel computing and Hugging Face’s Transformers for natural language processing, showcases Python’s adaptability. These advancements reflect the language’s capacity to integrate cutting-edge technologies and address specific needs, reinforcing its position as a go-to language for developers.
2. Advances in Machine Learning and AI
Python’s role in machine learning and artificial intelligence (AI) is poised for continued growth. The language’s readability and extensive library support have made it the preferred choice for many AI practitioners. The future will likely see Python playing a central role in advancements in AI, from developing more sophisticated algorithms to deploying AI models in production.
In particular, Python’s involvement in generative models, reinforcement learning, and neural network architecture innovations will continue to expand. The language’s ecosystem is also evolving to support new paradigms, such as federated learning and edge AI, which require specialized tools and frameworks.
3. Growth in Automation and Scripting
Automation remains a significant area where Python excels. Its simplicity makes it an ideal choice for scripting and automating repetitive tasks, from system administration to data processing. As businesses increasingly seek efficiency and automation solutions, Python’s role in this domain will grow. New libraries and tools that streamline automation processes will likely emerge, further solidifying Python’s position in this space.
Moreover, Python’s integration with tools like Ansible and Terraform for infrastructure automation is set to expand, reflecting the growing demand for DevOps and cloud-native solutions. The language’s ability to simplify complex workflows will continue to be a driving force in its adoption for automation tasks.
4. Innovations in Web Development
Python’s web development frameworks, such as Django and Flask, have been popular choices for building robust web applications. Looking ahead, the web development landscape is expected to evolve with trends like serverless architecture, microservices, and progressive web apps (PWAs). Python is well-positioned to adapt to these changes, with new frameworks and updates to existing ones likely to address these emerging needs.
Frameworks like FastAPI, which focuses on performance and ease of use, are already gaining traction. The evolution of web standards and technologies will drive further innovations in Python’s web development ecosystem, ensuring it remains a relevant choice for building modern web applications.
Python’s Evolving Role in the Tech Industry
1. Python in Machine Learning and AI
Python’s influence in machine learning and AI is set to grow exponentially. The language’s simplicity allows researchers and practitioners to focus on solving complex problems rather than dealing with intricate syntax. As AI becomes more embedded in various applications, from autonomous vehicles to healthcare diagnostics, Python will continue to be a central player.
The development of new libraries and frameworks tailored for specific AI applications will enhance Python’s capabilities. Additionally, the language’s role in facilitating collaboration through tools like Jupyter Notebooks and interactive environments will support continued innovation and experimentation in AI research.
2. Python and the Internet of Things (IoT)
The Internet of Things (IoT) represents another area where Python is expected to expand its role. Python’s versatility and ease of integration make it a suitable choice for developing IoT applications. With the growing number of connected devices and the need for data processing at the edge, Python’s use in IoT is likely to increase.
Frameworks like MicroPython and CircuitPython are already making it easier to develop applications for microcontrollers and embedded systems. As IoT technology evolves, Python’s ability to handle data from diverse sources and facilitate communication between devices will be crucial.
3. Python in Academia and Research
Python’s role in academia and research is also evolving. Its use in teaching programming and data analysis has become widespread due to its simplicity and readability. Educational institutions and research organizations increasingly adopt Python for teaching computer science, data science, and related fields.
Moreover, Python’s extensive libraries for scientific computing, such as SciPy and SymPy, support research across various domains. The language’s adaptability to new research methodologies and its active community contribute to its continued relevance in academic and research settings.
4. Python and Emerging Technologies
As new technologies emerge, Python’s adaptability ensures it remains relevant. The language’s ability to integrate with other languages and technologies, such as C++ and JavaScript, enhances its utility in diverse tech environments. Python’s role in blockchain technology, quantum computing, and augmented reality is expected to grow as these fields develop.
Python’s extensive community and ecosystem support innovation, making it a key player in exploring and implementing new technologies. Its versatility ensures that Python will continue to be a valuable asset in the tech industry’s ever-evolving landscape.
The future of Python is bright, with emerging trends and evolving roles ensuring its continued relevance and importance. From expanding libraries and frameworks to its growing impact in machine learning, automation, and IoT, Python’s adaptability and simplicity make it a language that will remain at the forefront of technological advancements. As new technologies and challenges arise, Python’s robust ecosystem and active community will drive its evolution, making it a vital tool for developers and researchers alike.
In summary, Python’s journey is far from over. Its ability to embrace new trends, adapt to evolving needs, and support diverse applications will continue to shape its role in the tech industry for years to come.
FAQs
Common Questions about Python
- What is Python primarily used for? Python is used for web development, data science, machine learning, automation, and more.
- Is Python good for beginners? Yes, Python’s simple syntax and readability make it an excellent choice for beginners.
- Can Python be used for mobile app development? While Python can be used for mobile development with frameworks like Kivy, it is not commonly used in this domain due to performance limitations.
Conclusion
Recap of Key Points Python is a versatile programming language with a wide range of applications, from web development and data science to machine learning and automation. Its simplicity, readability, and extensive library support make it a popular choice among developers.
Final Thoughts or Call-to-Action Whether you are a beginner looking to learn programming or an experienced developer exploring new domains, Python offers the tools and resources to help you succeed. Start exploring Python today and see how it can transform your projects.
External Authoritative Sources to Cite:
- Official Python documentation (docs.python.org)
- “Automate the Boring Stuff with Python” by Al Sweigart