Django, a powerful Python web framework, has gained immense popularity for its simplicity, scalability, and efficiency. This guide aims to provide a comprehensive overview of Django, covering everything from its history and setup to advanced features and real-world applications. Whether you are a software engineer, web developer, or tech enthusiast, this article will equip you with the knowledge to leverage Django effectively in your projects.
1. Introduction
Overview of Django
Brief History and What It Is
Django was created in 2005 by Adrian Holovaty and Simon Willison while they were working at the Lawrence Journal-World newspaper. The framework was designed to facilitate the rapid development of web applications by providing a high-level abstraction for common web development tasks. Named after the jazz guitarist Django Reinhardt, the framework aims to make complex web development tasks simpler and more efficient.
Key Features:
- Batteries-Included Philosophy: Django comes with a wide array of built-in features such as an ORM, an admin interface, and authentication systems, allowing developers to focus on building their applications rather than reinventing the wheel.
- MTV Architecture: Django follows the Model-Template-View (MTV) architecture, which separates data, user interface, and logic, leading to cleaner and more maintainable code.
Why It’s Popular
Django’s popularity can be attributed to several factors:
- Rapid Development: Django’s built-in features and convention-over-configuration approach significantly speed up the development process.
- Scalability: Used by major websites like Instagram and Pinterest, Django can handle large-scale applications efficiently.
- Security: Django includes built-in protection against common security threats such as SQL injection, cross-site scripting, and cross-site request forgery.
Who Should Use Django?
Target Audience
- Software Engineers: Django is ideal for software engineers who need a robust framework for building scalable web applications. Its extensive documentation and large community make it accessible even to those new to web development.
- Web Developers: For web developers, Django offers a comprehensive toolset that simplifies the development process. Its focus on DRY (Don’t Repeat Yourself) principles helps in writing clean and maintainable code.
- Tech Companies: Tech companies benefit from Django’s scalability and versatility, making it a popular choice for building both startup MVPs and large-scale enterprise applications.
Use Cases
- Enterprise Applications: Django’s features such as the admin interface and ORM make it suitable for building complex enterprise applications.
- Startups: Startups leverage Django for rapid development and prototyping due to its out-of-the-box features and ease of deployment.
Why Learn Django?
Benefits
- Efficient Development: Django’s “batteries-included” approach means that many common tasks are already handled, allowing developers to focus on writing unique application code. For example, the Django ORM handles database interactions, reducing the need for custom SQL queries.
- Career Opportunities: Proficiency in Django opens up various career opportunities, including roles like Backend Developer, Full-Stack Developer, and Django Specialist. The demand for Django skills in job markets is high due to its widespread use.
- Community and Ecosystem: Django has a vibrant community and a rich ecosystem of third-party packages, which can enhance its functionality and provide solutions to common problems.
Real-World Applications
- Case Studies: Websites like Instagram, Spotify, and The Washington Post use Django to handle high traffic and complex requirements. These case studies demonstrate Django’s capability to power successful, large-scale applications.
2. Getting Started with Django
Setting Up Your Development Environment
Installing Python
Before installing Django, you need Python installed on your system. Python can be downloaded from Python’s official website. Follow the installation instructions for your operating system:
- Windows: Download the installer and follow the prompts. Ensure that you check the option to add Python to your PATH.
- macOS: Python is pre-installed, but you can use Homebrew to install the latest version (
brew install python
). - Linux: Use your package manager to install Python (
sudo apt-get install python3
for Debian-based distributions).
Installing Django via pip
Django can be installed using pip, Python’s package manager. Open your terminal or command prompt and run:
pip install django
This command downloads and installs the latest version of Django.
Setting up a Virtual Environment
Virtual environments allow you to manage dependencies for different projects separately. To create a virtual environment:
- Create a Virtual Environment: Run
python -m venv myenv
in your project directory. - Activate the Virtual Environment:
- Windows:
myenv\Scripts\activate
- macOS/Linux:
source myenv/bin/activate
- Windows:
- Install Django within the Virtual Environment: With the virtual environment activated, run
pip install django
.
Choosing an IDE or Code Editor
Popular IDEs and editors for Django development include:
- PyCharm: A powerful IDE with Django-specific features like code completion and debugging.
- VS Code: A lightweight, customizable editor with extensions for Django development.
- Sublime Text: Known for its speed and simplicity, with Django-specific plugins available.
Creating Your First Django Project
Project Structure Overview
When you create a Django project, the basic structure includes:
- manage.py: A command-line utility for administrative tasks.
- project_name/: The project directory containing settings and configuration.
- project_name/settings.py: Configuration settings for your project.
- project_name/urls.py: URL declarations for the project.
- project_name/wsgi.py: WSGI configuration for deploying the project.
Running the Development Server
To start the development server, navigate to your project directory and run:
To start the development server, navigate to your project directory and run:
python manage.py runserver
This command starts a server on http://127.0.0.1:8000/
, where you can view your project in a web browser.
Basic Settings Configuration
In settings.py
, you configure essential settings:
- DATABASES: Define your database settings (default is SQLite).
- INSTALLED_APPS: List the applications included in your project.
- ALLOWED_HOSTS: Specify the hosts/domain names that are valid for this site.
Understanding Django’s MVT Architecture
Models, Views, and Templates Explained
- Models: Define the data structure of your application. Models are Python classes that subclass
django.db.models.Model
. For example:from django.db import models class Author(models.Model): name = models.CharField(max_length=100)
- Views: Handle user requests and return responses. Views can be function-based or class-based. Example of a function-based view:
from django.http import HttpResponse def index(request): return HttpResponse("Hello, world!")
- Templates: Define the HTML structure of your application. Templates use Django’s template language to dynamically generate HTML. Example:
<!DOCTYPE html> <html> <body> <h1>Hello, {{ name }}!</h1> </body> </html>
How MVT Differs from MVC
- Model-View-Controller (MVC): In MVC, the controller handles user input and updates the model and view.
- Model-Template-View (MVT): In Django’s MVT, the view handles the user input and updates the model and template, while the template renders the data to the user.
3. Django Models
Introduction to Django Models
What Are Models in Django?
Models in Django represent the data structure of your application and handle database interactions. Each model class corresponds to a table in the database.
Defining Models in models.py
To define a model, create a class in models.py
that subclasses django.db.models.Model
. For example:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=100)
published_date = models.DateField()
Field Types and Options
Common Field Types
- CharField: Used for small to medium-sized text fields. Example:
title = models.CharField(max_length=200)
- IntegerField: Used for integer values. Example:
pages = models.IntegerField()
- DateField: Used for date values. Example:
published_date = models.DateField()
Field Options
- null: Determines if the field can store NULL values in the database.
- blank: Determines if the field is required in forms.
- default: Provides a default value for the field.
Relationships Between Models
Types of Relationships
- One-to-One: One model instance is related to one instance of another model. Example:
class Profile(models.Model): user = models.OneToOneField(User, on_delete=models.CASCADE)
- Many-to-One: Many instances of one model are related to one instance of another model. Example:
class Comment(models.Model): post = models.ForeignKey(Post, on_delete=models.CASCADE)
- Many-to-Many: Many instances of one model are related to many instances of another model. Example:
class Student(models.Model): courses = models.ManyToManyField(Course)
Use Cases
- One-to-One: Useful for extending user profiles with additional information.
- Many-to-One: Suitable for models like comments associated with a single post.
- Many-to-Many: Ideal for models like students enrolled in multiple courses.
Working with the Django ORM
QuerySets and Filters
- QuerySets: Allow you to retrieve and manipulate data from the database. Example:
books = Book.objects.all()
- Filters: Used to narrow down query results. Example:
books = Book.objects.filter(author='J.K. Rowling')
CRUD Operations
- Create: Add new objects to the database. Example:
Book.objects.create(title='New Book', author='Author Name')
- Read: Retrieve data from the database. Example:
book = Book.objects.get(pk=1)
- Update: Modify existing objects. Example:
book.title = 'Updated Title' book.save()
- Delete: Remove objects from the database. Example:
book.delete()
Model Migrations
Understanding Migrations
Migrations are a way to propagate changes you make to your models into your database schema. They are created based on changes to your models.
Applying Migrations in Django
- Creating Migrations: Run
python manage.py makemigrations
to generate migration files. - Applying Migrations: Run
python manage.py migrate
to apply these migrations to your database.
Django Views and URL Routing are foundational elements that shape how your application handles user requests and delivers responses. This article explores Django Views, URL Routing, and Templates in detail, providing a comprehensive guide to their implementation and best practices.
1. Introduction to Django Views
In Django, Views are the heart of your application, processing requests and returning responses. They act as a bridge between the models (which handle data) and the templates (which render the data).
Function-Based Views vs. Class-Based Views
Function-Based Views (FBVs)
Function-Based Views are simple Python functions that receive a web request and return a web response. They are straightforward to implement and are ideal for simple views.
Example:
from django.http import HttpResponse
def home(request):
return HttpResponse("Welcome to the Django Home Page!")
Advantages:
- Simplicity: Easy to understand and implement.
- Explicitness: Clear structure of request handling.
Disadvantages:
- Scalability: Can become unwieldy for complex views.
Class-Based Views (CBVs)
Class-Based Views offer a more organized and reusable approach. They use Python classes to handle requests, allowing for inheritance and mixins, which can lead to cleaner and more maintainable code.
Example:
from django.http import HttpResponse
from django.views import View
class HomeView(View):
def get(self, request):
return HttpResponse("Welcome to the Django Home Page!")
Advantages:
- Reusability: Easier to reuse and extend functionality.
- Organization: Better suited for complex views with multiple HTTP methods.
Disadvantages:
- Complexity: Can be harder to grasp initially.
2. Routing in Django
Routing in Django involves mapping URLs to views. This process is essential for directing user requests to the appropriate views.
urls.py and URL Patterns
The urls.py
file in each Django app contains URL patterns that map URLs to views. The URL patterns are defined using Django’s path()
or re_path()
functions.
Example:
from django.urls import path
from .views import HomeView, AboutView
urlpatterns = [
path('', HomeView.as_view(), name='home'),
path('about/', AboutView.as_view(), name='about'),
]
Components:
- Path Function: Maps a URL pattern to a view.
- Name Parameter: Assigns a name to the URL pattern, useful for reverse URL resolution.
Including URLs from Different Apps
To keep URL configurations modular, Django allows you to include URL patterns from different apps.
Example:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('app1.urls')),
path('blog/', include('blog.urls')),
]
In this setup, the main URL configuration includes URLs from app1
and blog
, facilitating modular organization.
3. Working with Templates
Django Templates are used to render dynamic HTML content. They provide a way to separate the presentation logic from the business logic of your application.
Template Structure and Syntax
Templates in Django are plain text files with a special syntax for embedding dynamic content. They use a combination of HTML and Django Template Language (DTL).
Example:
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ header }}</h1>
<p>{{ content }}</p>
</body>
</html>
In this example, {{ title }}
, {{ header }}
, and {{ content }}
are placeholders for dynamic content provided by the view.
Template Inheritance
Template inheritance allows you to create a base template with common layout elements and extend it in other templates.
Base Template Example:
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}My Site{% endblock %}</title>
</head>
<body>
<header>{% block header %}Header{% endblock %}</header>
<main>{% block content %}Content{% endblock %}</main>
<footer>Footer</footer>
</body>
</html>
Child Template Example:
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block header %}Welcome to My Site{% endblock %}
{% block content %}
<p>This is the home page.</p>
{% endblock %}
Django Template Tags and Filters
Built-in Template Tags
Django provides several built-in template tags to control logic and render dynamic content.
- {% if %}: Conditional statements.
- {% for %}: Looping through items.
Example:
{% if user.is_authenticated %}
<p>Hello, {{ user.username }}!</p>
{% else %}
<p>Please log in.</p>
{% endif %}
Custom Template Tags
You can also create custom template tags and filters to extend Django’s templating capabilities.
Creating a Custom Template Tag:
from django import template
register = template.Library()
@register.simple_tag
def my_custom_tag(arg1, arg2):
return f"Result: {arg1} and {arg2}"
Using the Custom Tag in a Template:
{% load my_custom_tags %}
<p>{% my_custom_tag "Hello" "World" %}</p>
Handling Forms in Django
Forms in Django are used to collect and validate user input. Django provides a form system that simplifies form handling, validation, and processing.
Working with forms.py
Create forms by defining classes in forms.py
that inherit from forms.Form
or forms.ModelForm
.
Example:
from django import forms
from .models import Book
class BookForm(forms.ModelForm):
class Meta:
model = Book
fields = ['title', 'author', 'published_date']
Validating and Processing Form Data
Forms include built-in validation methods and allow for custom validation logic.
Example of Custom Validation:
from django.core.exceptions import ValidationError
def clean_title(self):
title = self.cleaned_data.get('title')
if 'badword' in title:
raise ValidationError('Invalid title')
return title
Processing Form Data in a View:
from django.shortcuts import render, redirect
from .forms import BookForm
def add_book(request):
if request.method == 'POST':
form = BookForm(request.POST)
if form.is_valid():
form.save()
return redirect('book_list')
else:
form = BookForm()
return render(request, 'add_book.html', {'form': form})
4. Understanding Django Templates
Django Templates provide a powerful mechanism for rendering dynamic HTML. They are designed to keep presentation logic separate from business logic, making your application more maintainable.
Template Structure and Syntax
Django Templates use a combination of HTML and template language to generate dynamic content.
Example Template:
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ header }}</h1>
<p>{{ content }}</p>
</body>
</html>
In this template, {{ title }}
, {{ header }}
, and {{ content }}
are placeholders for dynamic data provided by the view.
Template Inheritance
Template inheritance is a powerful feature that allows you to define a base template with common elements and create child templates that extend it.
Base Template:
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}My Site{% endblock %}</title>
</head>
<body>
<header>{% block header %}Header{% endblock %}</header>
<main>{% block content %}Content{% endblock %}</main>
<footer>Footer</footer>
</body>
</html>
Child Template:
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block header %}Welcome to My Site{% endblock %}
{% block content %}
<p>This is the home page.</p>
{% endblock %}
Django Template Tags and Filters
Built-in Template Tags
Django provides several built-in template tags for controlling the flow of your template.
- {% if %}: For conditional statements.
- {% for %}: For looping through items.
- {% include %}: For including other templates.
Example:
{% if user.is_authenticated %}
<p>Hello, {{ user.username }}!</p>
{% else %}
<p>Please log in.</p>
{% endif %}
Custom Template Tags and Filters
Creating a Custom Template Tag (continued)
from django import template
register = template.Library()
@register.simple_tag
def my_custom_tag(arg1, arg2):
return f"Result: {arg1} and {arg2}"
Using the Custom Tag in a Template:
{% load my_custom_tags %}
<p>{% my_custom_tag "Hello" "World" %}</p>
Custom Template Filters
Custom template filters modify the presentation of data in templates. They are defined using the @register.filter
decorator.
Creating a Custom Filter:
from django import template
register = template.Library()
@register.filter
def reverse_string(value):
return value[::-1]
Using the Custom Filter in a Template:
<p>{{ some_text|reverse_string }}</p>
In this example, reverse_string
is a custom filter that reverses a given string.
5. Working with Static Files
Static files (CSS, JavaScript, images) are crucial for adding styling and interactivity to your Django application. Django provides a framework for managing these files efficiently.
Managing Static Files
Django uses the {% static %}
template tag to refer to static files. This tag ensures that the correct URL is generated for the static files, considering your project’s settings.
Setting Up Static Files in Django:
- Configure Static Settings in
settings.py
:
STATIC_URL = '/static/'
STATICFILES_DIRS = [os.path.join(BASE_DIR, "static")]
STATIC_ROOT = os.path.join(BASE_DIR, "staticfiles")
- Organize Static Files in the Project Directory:Create a
static
directory at the root of your project and within each app if needed. - Use
{% static %}
Tag in Templates:
Example HTML Template:
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
<link rel="stylesheet" type="text/css" href="{% static 'styles/main.css' %}">
</head>
<body>
<h1>{{ header }}</h1>
<p>{{ content }}</p>
<script src="{% static 'scripts/main.js' %}"></script>
</body>
</html>
In this example, {% static 'styles/main.css' %}
and {% static 'scripts/main.js' %}
dynamically generate the correct URLs for the static files.
Using the {% static %}
Tag
The {% static %}
tag is used to refer to static files in templates. It automatically handles the URL generation, making sure it works correctly in both development and production environments.
Example Usage:
<img src="{% static 'images/logo.png' %}" alt="Site Logo">
This tag ensures that the URL for the image is correctly resolved based on your static files configuration.
6. Best Practices for Django Views and Templates
Optimizing Views
- Use Class-Based Views for Reusability: Class-Based Views can make your code more modular and reusable, especially for views that require multiple HTTP methods.
- Minimize Business Logic in Views: Keep views focused on handling requests and responses. Move complex logic to models or services.
- Implement Pagination for Large Datasets: Use Django’s pagination tools to handle large datasets efficiently.
Efficient URL Routing
- Use Namespaces for URL Patterns: Namespaces help in organizing and managing URLs, especially in large projects with multiple apps.
- Avoid Hardcoding URLs: Use the
{% url %}
template tag orreverse()
function to generate URLs dynamically.
Example:
<a href="{% url 'about' %}">About Us</a>
Managing Templates
- Leverage Template Inheritance: Use base templates to maintain a consistent layout and reduce duplication across your templates.
- Keep Templates DRY (Don’t Repeat Yourself): Avoid repeating code. Use template inheritance and includes to reuse common elements
Django’s views and URL routing mechanisms, combined with its powerful templating system, provide a robust framework for web development. Understanding and effectively using these features is crucial for building efficient and maintainable Django applications.
Key Takeaways:
- Views: Serve as the core logic handlers in Django, with options for function-based and class-based implementations.
- URL Routing: Maps URLs to views, enabling modular and scalable URL management.
- Templates: Separate presentation logic from business logic, facilitating a clean and maintainable codebase.
- Static Files: Manage and serve CSS, JavaScript, and images, enhancing the visual and interactive aspects of your site.
By mastering these elements, you can harness the full potential of Django, creating dynamic and efficient web applications that adhere to best practices and industry standards.
Django Admin Interface
Overview of Django Admin
The Django Admin Interface is a powerful feature that provides an automatic, web-based interface for managing your application’s data. It is built on top of Django’s models and provides a way for administrators to perform CRUD (Create, Read, Update, Delete) operations directly from the web browser without needing to write custom forms or views.
Key Features:
- Automatic Interface Generation: Django automatically generates a user-friendly interface based on your models.
- Customizable: You can extend and customize the admin interface to fit specific needs.
- Security: Provides robust built-in security features to manage user access and permissions.
Enabling and Accessing the Admin Interface
To use the Django Admin Interface, you need to perform the following steps:
- Ensure Django Admin is Installed: Django Admin is included in the default Django installation. Make sure it is listed in your
INSTALLED_APPS
setting insettings.py
:INSTALLED_APPS = [ # Other installed apps 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', ]
- Run Migrations: Django Admin requires some initial database tables. Run the migrations to set these up:
python manage.py migrate
- Create a Superuser: To access the admin interface, you need a superuser account. Create one using:
python manage.py createsuperuser
Follow the prompts to set up the superuser account. - Accessing the Admin Interface: Start the development server and access the admin interface at
/admin
:python manage.py runserver
Navigate tohttp://127.0.0.1:8000/admin
and log in with the superuser credentials.
Customizing the Django Admin
Django provides various ways to customize the admin interface to better suit your needs. Customization can involve modifying the display of models, adding search and filter capabilities, or creating custom actions.
Customizing Models in the Admin
To customize how a model is displayed in the admin interface, you need to create a class that inherits from admin.ModelAdmin
and register it with the admin site.
Example:
from django.contrib import admin
from .models import Book
class BookAdmin(admin.ModelAdmin):
list_display = ('title', 'author', 'published_date')
search_fields = ('title', 'author__name')
list_filter = ('published_date', 'author')
admin.site.register(Book, BookAdmin)
In this example:
list_display
specifies the fields to be displayed in the list view.search_fields
enables search functionality on specified fields.list_filter
adds filter options in the sidebar based on specified fields.
Adding Filters and Search Capabilities
Filters and search capabilities can be added to make data management easier.
Adding Filters:
class BookAdmin(admin.ModelAdmin):
list_filter = ('author', 'published_date')
Adding Search Functionality:
class BookAdmin(admin.ModelAdmin):
search_fields = ('title', 'author__name')
Creating Custom Admin Actions
Custom admin actions allow you to define custom operations that can be performed on selected items in the admin interface.
Example:
def mark_as_published(modeladmin, request, queryset):
queryset.update(status='published')
mark_as_published.short_description = "Mark selected books as published"
class BookAdmin(admin.ModelAdmin):
actions = [mark_as_published]
In this example, the custom action mark_as_published
allows marking multiple selected books as published.
Admin Security Best Practices
Securing the Admin Panel:
- Use Strong Passwords: Ensure that admin users have strong passwords to prevent unauthorized access.
- Enable HTTPS: Use HTTPS to secure data transmitted between the client and server. Configure your web server to enforce HTTPS.
- Limit Access: Restrict access to the admin interface to specific IP addresses if possible, using web server configuration.
Managing User Permissions:
Django offers a robust and flexible permission system, enabling you to control what users can and cannot do within the admin interface.
Default Permissions:
By default, Django provides four basic permissions—add, change, delete, and view—for each model. These are automatically created when you generate a model.
Custom Permissions:
You can define custom permissions directly within your model’s Meta
class. This allows you to add more specific permissions as needed. For example:
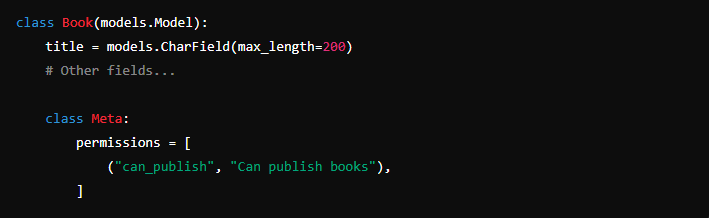
Assigning Permissions:
Permissions can be assigned to individual users or groups through the Django admin interface. Navigate to the “Users” and “Groups” sections, where you can manage and assign permissions as required.
Django Authentication and Authorization
Django provides a powerful and comprehensive authentication and authorization framework out of the box, allowing developers to manage users, authenticate them, and control their permissions seamlessly. This framework is flexible enough to accommodate custom user models, third-party authentication services, and complex permission structures.
Built-in Authentication System
Django’s built-in authentication system is designed to handle the most common use cases for user management, including registration, login, password management, and user permissions. It provides:
- User models: The foundation of the authentication system, representing the users of your application.
- Authentication backends: Components that define how Django will authenticate a user.
- Session management: Django’s session framework, which stores user-specific data between requests.
User Model, Login, and Registration
User Model
Django comes with a default User
model that includes essential fields such as username
, password
, email
, first_name
, and last_name
. This model is highly customizable, allowing developers to extend it or replace it with a completely custom user model.
Example Usage:

The create_user
method automatically handles password hashing, making it easy to securely create new users.
Login
Django provides built-in views to manage user authentication processes, such as logging in. The LoginView
can be utilized to authenticate users by configuring it in your urls.py
file.
Example:
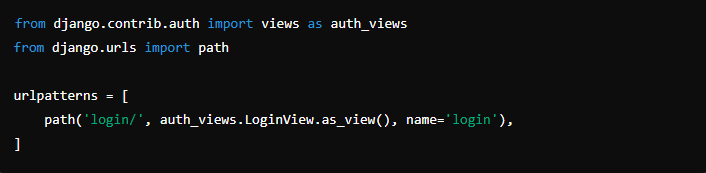
Django expects a login template at templates/registration/login.html
by default, which can be customized according to the design and functionality requirements of your application.
Registration
For user registration, Django offers the UserCreationForm
, which simplifies the process of creating new users. This form handles common tasks such as validation and saving the user to the database.
Example Form and View:
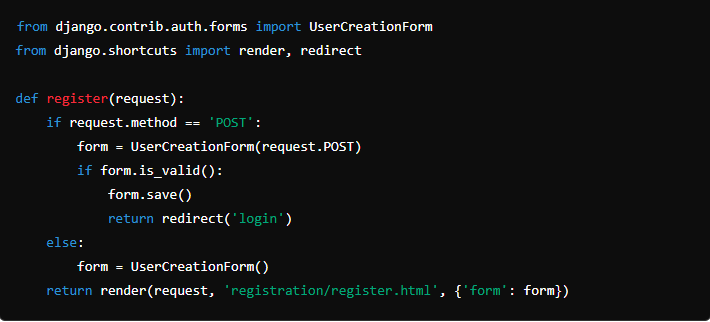
This view handles both GET and POST requests, rendering the registration form and processing the form data when submitted.
Managing Permissions and Groups
Permissions and groups in Django allow fine-grained control over what users can do in your application. By assigning specific permissions to users or groups, you can manage access to various parts of your application.
Creating and Assigning Permissions
Django automatically creates four basic permissions for each model: add
, change
, delete
, and view
. However, you can define custom permissions that suit your application’s specific needs.
Creating Custom Permissions:
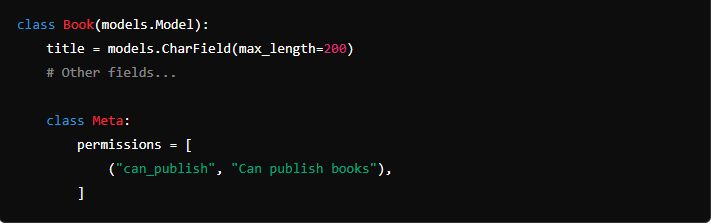
This example adds a custom permission, can_publish
, which can be used to control which users are allowed to publish books.
Assigning Permissions to Users or Groups: Permissions can be assigned through the Django admin interface or programmatically using the Django shell.
Example:
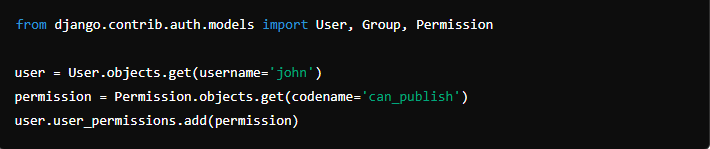
This code snippet assigns the can_publish
permission to a user named john
.
Managing Groups
Groups in Django serve as a convenient way to bundle multiple permissions together. Users can be added to groups, inheriting all the permissions associated with that group. This is particularly useful for managing roles such as Editor
, Moderator
, or Admin
.
Creating a Group and Assigning Permissions:
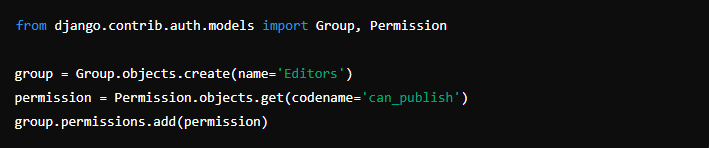
In this example, a group named Editors
is created, and the can_publish
permission is assigned to it. Any user added to the Editors
group will inherit this permission.
Custom User Models
While Django’s default User
model is sufficient for many applications, there are cases where you might need to extend or completely replace it to accommodate additional fields or custom authentication behavior.
Extending the Default User Model
To add custom fields to the default User
model, you can subclass AbstractUser
, which includes all the fields and methods of the default User
model, allowing you to extend it.
Example Custom User Model:
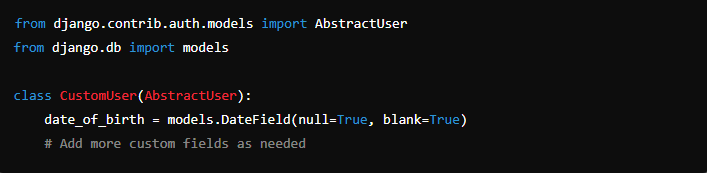
Updating Settings:

By setting AUTH_USER_MODEL
, Django will use your custom user model throughout the application.
Creating a Custom User Model
For more complex requirements, you can create a custom user model from scratch by subclassing AbstractBaseUser
and PermissionsMixin
. This gives you full control over the user model’s behavior, including the fields used for authentication.
Example:
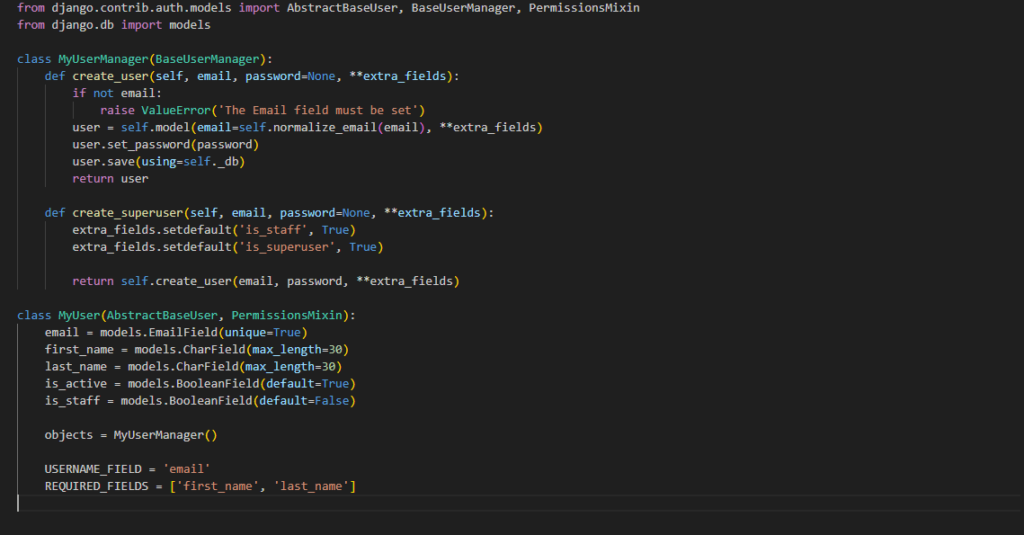
This custom user model uses an email address as the primary identifier for authentication instead of a username.
Integrating Social Authentication
Django provides support for social authentication via third-party packages, with django-allauth
being one of the most popular options. django-allauth
allows users to authenticate using social accounts like Google, Facebook, or GitHub.
Using Django Allauth
Installation: To get started with django-allauth
, install the package using pip:
Configuration: After installation, add allauth
to your INSTALLED_APPS
and configure authentication backends in your settings.py
file:
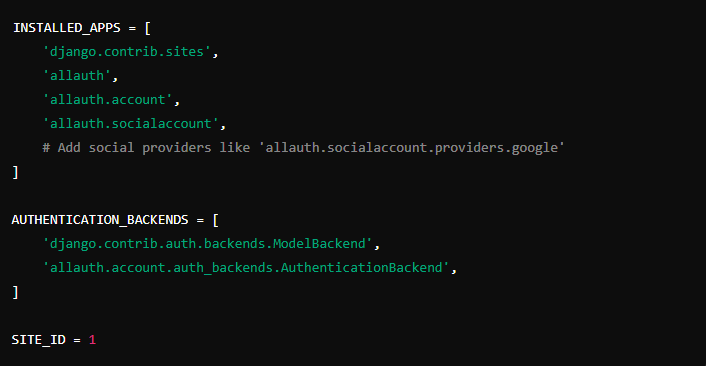
URLs and Views: Include allauth
URLs in your urls.py
file to handle authentication-related routes:
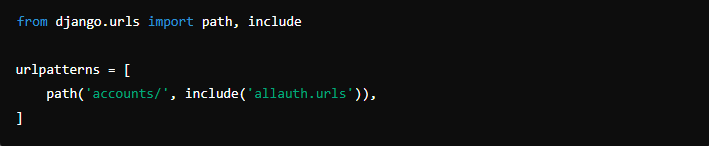
Templates: django-allauth
comes with default templates for login, registration, and password management. You can override these templates to match the look and feel of your application.
OAuth and Social Login Integration
To integrate OAuth and social login, you need to configure social authentication providers within the Django admin interface under the “Social Applications” section. This involves:
- Creating a social application: Navigate to the Django admin, and add a new social application for each provider (e.g., Google, Facebook). Enter the client ID and secret provided by the social provider.
- Configuring OAuth redirect URLs: Set the appropriate OAuth redirect URLs in the provider’s developer console (e.g., Google Developer Console). This URL is typically something like
https://yourdomain.com/accounts/google/login/callback/
.
Example URL Configuration:
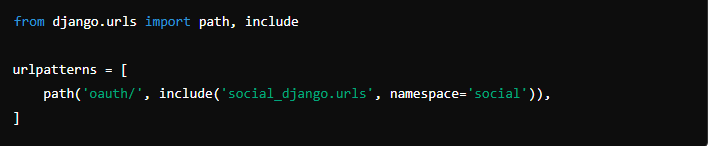
Customizing Social Login Flow
django-allauth
is highly customizable, allowing you to tweak the social login flow to meet your specific requirements. For instance, you can:
- Customize user data handling: Define signals to process user data after authentication.
- Override default views: Replace the default views provided by
django-allauth
with custom views to handle specific business logic. - Add additional scopes: Modify the scopes requested during social authentication to access more user data.
By leveraging django-allauth
, you can provide a seamless social login experience for your users, enhancing the convenience and security of your authentication system.
Django’s authentication and authorization framework is a robust solution that provides everything needed for managing users, permissions, and security in modern web applications. From the simplicity of its default user model to the flexibility of custom authentication systems and social logins, Django ensures that developers have the tools to build secure and scalable applications. Whether you’re working on a small project or a large enterprise application, Django’s authentication and authorization features will meet your needs and help you maintain the integrity and security of your application.
Django REST Framework (DRF)
Introduction to Django REST Framework
What is DRF and Why Use It?
Django REST Framework (DRF) is a powerful, flexible toolkit that simplifies the process of building Web APIs within Django applications. As a third-party library, DRF enhances Django’s capabilities, making it an ideal choice for developers looking to efficiently create and manage RESTful APIs. Whether you’re building simple APIs for a small project or complex ones for a large enterprise application, DRF provides the tools and features necessary to streamline API development.
Key Features of DRF
- Serialization: DRF’s serialization mechanism allows for the conversion of complex data types, like Django models, into easily transferable formats such as JSON or XML. It also supports deserialization, enabling the reconstruction of these complex types from the data received via the API.
- Authentication and Permissions: DRF includes robust, built-in support for various authentication methods (e.g., token-based, session-based) and permissions, allowing fine-grained control over who can access specific API endpoints.
- ViewSets and Routers: These abstractions simplify the creation of standard CRUD (Create, Retrieve, Update, Delete) operations, enabling automatic URL routing and reducing boilerplate code.
- Browsable API: One of DRF’s most user-friendly features is the browsable API, which provides an interactive, web-based interface for exploring and testing APIs directly from the browser.
Benefits of Using DRF
- Ease of Use: DRF is designed to be intuitive, allowing developers to quickly set up and manage APIs without needing to write extensive boilerplate code.
- Extensibility: DRF’s modular architecture makes it easy to extend and customize, catering to the specific needs of your project.
- Integration: Seamlessly integrates with Django’s ORM, authentication system, and other built-in features, making it an excellent choice for projects already using Django.
- Documentation: Automatically generates interactive API documentation, making it easier for developers to understand and work with the API.
Setting Up a REST API with Django
Installing and Configuring DRF
To get started with DRF, you’ll first need to install the package via pip: using pip install djangorestframework
Next, add rest_framework
to the INSTALLED_APPS
list in your Django project’s settings.py
file:

Optionally, you can configure global settings for DRF in settings.py
to customize default permissions and authentication methods:
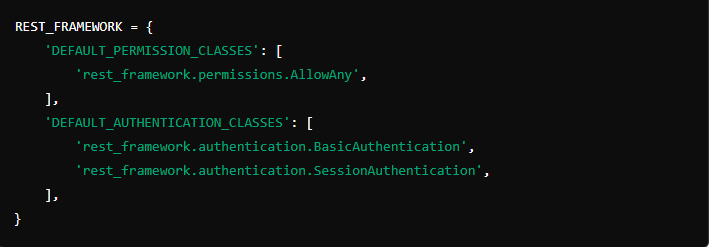
Serializers in DRF
Writing Serializers and Serializer Fields
Serializers in DRF serve a similar purpose to Django forms, facilitating the conversion of complex data types—like Django models—into simpler, JSON-serializable types. They also handle deserialization, enabling you to validate and save incoming data.
Creating a Serializer: Below is an example of how to define a serializer for a Django model:
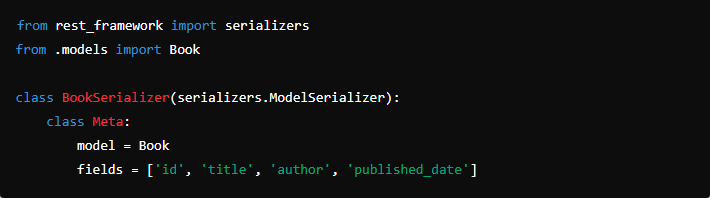
Key Concepts
- ModelSerializer: Automatically generates a serializer based on a specified Django model, reducing the need for manual field definitions.
- fields: Specifies which model fields should be included in the serialized output, providing control over the data exposed by the API.
Serializer Fields: DRF offers a variety of field types tailored to different data formats:
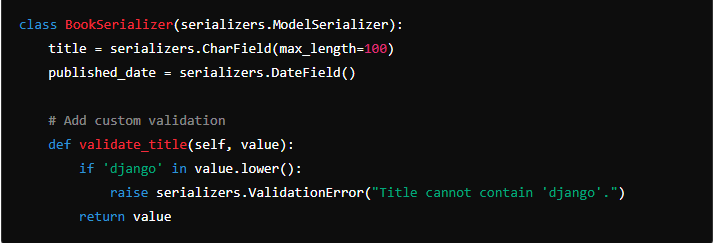
Custom Fields: If your API requires custom data handling, you can define custom serializer fields:
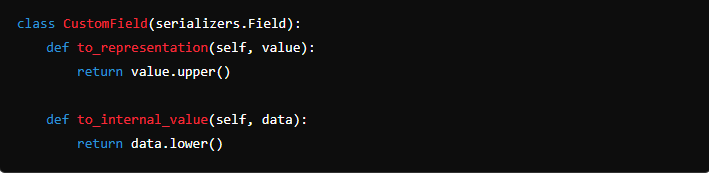
ViewSets and Routers
Simplifying Views with ViewSets
ViewSets in DRF provide a high-level abstraction that simplifies the implementation of CRUD operations. When paired with routers, they can automatically generate URL patterns, significantly reducing the amount of code you need to write.
Creating a ViewSet:
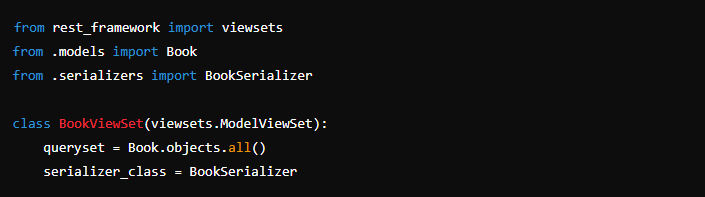
Key Concepts
- ModelViewSet: A ViewSet that provides default implementations for standard CRUD operations, allowing you to focus on customizing your API’s behavior.
- queryset: Defines the data that the view will operate on, typically all objects of a given model.
- serializer_class: Indicates the serializer class that will handle data serialization and deserialization for the view.
Using Routers
Routers automatically generate URL patterns for your ViewSets, adhering to DRF’s philosophy of reducing redundancy.
Creating a Router:
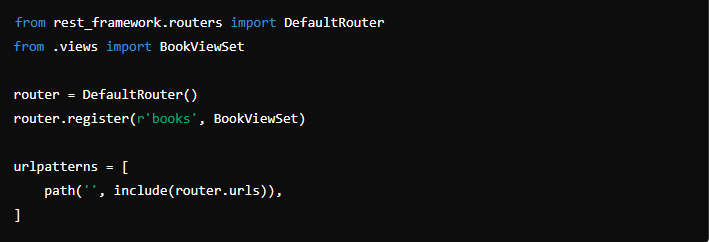
Benefits
- Automatic URL Routing: Routers handle URL mapping, saving you from writing and maintaining verbose URL configuration code.
- DRY Principle: By reducing repetition, routers help keep your codebase concise and maintainable.
Authentication and Permissions in DRF
Token-Based Authentication
Token-based authentication allows users to authenticate via tokens instead of traditional sessions, making it ideal for stateless applications.
Installation: pip install djangorestframework-simplejwt
Configuration: Add token authentication to your settings.py
:
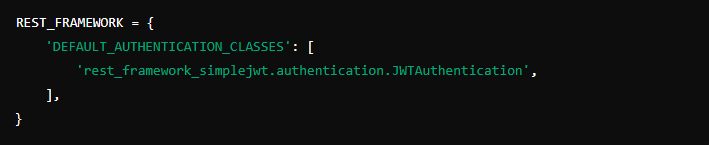
Generating Tokens: To enable token generation and refreshing, you can create corresponding views:
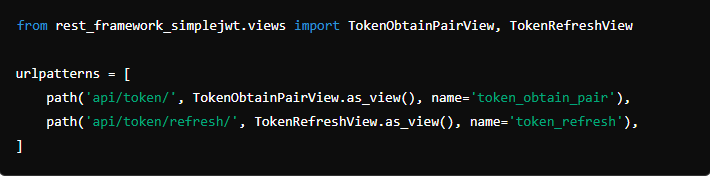
Custom Permission Classes
DRF enables the creation of custom permission classes, giving you granular control over access to your API.
Creating a Custom Permission:
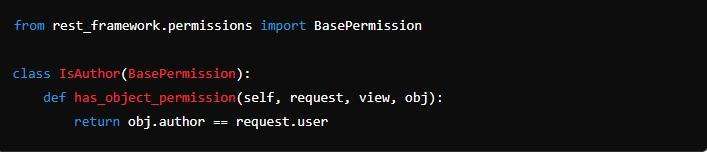
Usage:

Benefits
- Fine-Grained Control: Custom permissions allow you to enforce complex access rules, tailored to your application’s needs.
Advanced DRF Features
Pagination, Filtering, and Ordering
DRF includes built-in support for handling large datasets through pagination, filtering, and ordering, enhancing the performance and usability of your API.
Pagination: Set up pagination in settings.py
:

Usage in ViewSets:

Filtering: Implement filtering using DjangoFilterBackend
:
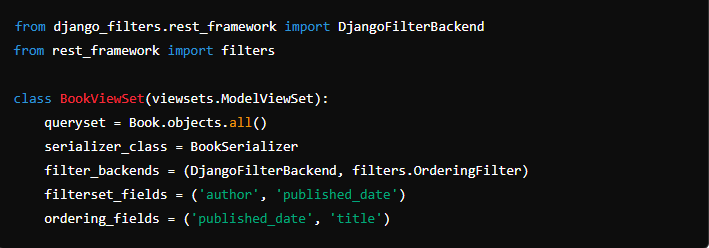
Ordering: Define ordering within the viewset:

Working with Nested Serializers
Nested serializers in DRF allow you to represent model relationships more comprehensively.
Defining Nested Serializers:
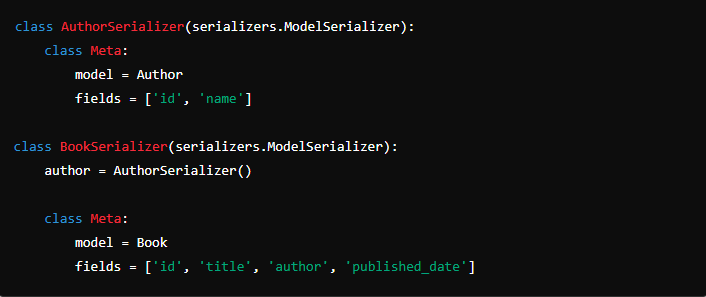
Benefits
- Rich Representations: Nested serializers enable detailed and expressive representations of related objects, improving the clarity and utility of your API responses.
Django Middleware
Understanding Middleware in Django
What is Middleware?
Middleware in Django is a powerful system that allows developers to interact with and modify the request and response processes globally. It acts as a layer between the Django application and the incoming requests, enabling you to customize the behavior of your application.
How Middleware Operates:
Middleware components are executed in a sequential order for every request and response. These components can:
- Intercept and modify requests before they reach the view.
- Alter responses before they are sent back to the client.
- Handle exceptions raised within views.
Middleware Lifecycle Phases:
- Request Phase: Middleware processes the request before it is handed over to the view. This is where tasks such as request validation, authentication, and logging typically occur.
- View Phase: The request is passed to the appropriate view function. Middleware can also influence how the view operates or modify the response that the view generates.
- Response Phase: After the view has processed the request, middleware comes into play again to alter or augment the response before it is returned to the client.
Utilizing Built-in Middleware
Django provides a variety of built-in middleware classes to handle common functionalities within an application.
Examples of Built-in Middleware:
django.middleware.security.SecurityMiddleware
: Enhances security by adding security-related HTTP headers.django.middleware.csrf.CsrfViewMiddleware
: Protects against Cross-Site Request Forgery (CSRF) attacks.django.middleware.common.CommonMiddleware
: Offers common utilities such as URL rewriting and handling of ETags.
Configuring Middleware in settings.py
:
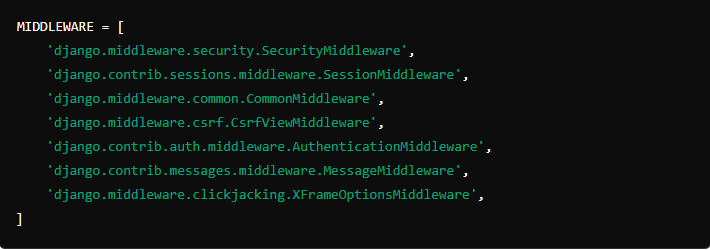
Customizing Middleware
Middleware can be customized to perform specific tasks that are unique to your application.
Example: Logging Middleware
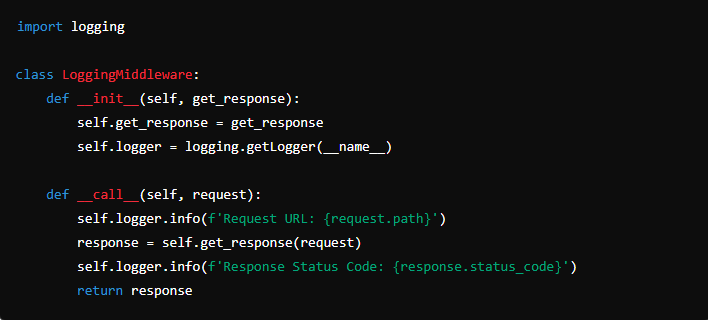
Creating Custom Middleware
Writing and Implementing Custom Middleware
To create custom middleware, define a middleware class with methods to process requests and responses.
Example: User-Agent Logging Middleware
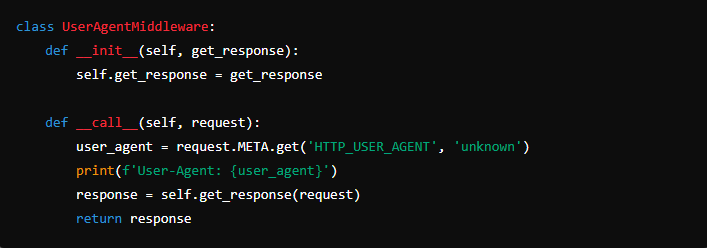
Incorporating Custom Middleware into Settings:
To activate your custom middleware, add it to the MIDDLEWARE
list in settings.py
:
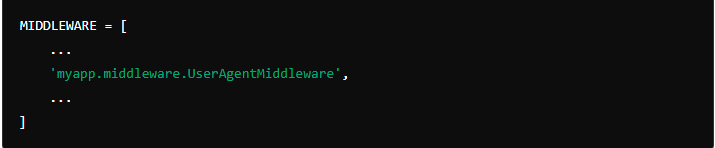
Testing and Debugging Middleware
- Testing: Use Django’s test client to simulate requests and verify that your middleware behaves as expected.
- Debugging: Incorporate logging or print statements within your middleware to trace its execution flow and identify issues.
Django Signals
Introduction to Django Signals
Django signals are a powerful feature within the Django framework that allows different parts of an application to communicate with each other without being tightly coupled. They enable you to trigger custom logic when certain actions occur elsewhere in the application, making your code more modular and easier to maintain.
What Are Signals and Why Use Them?
Signals in Django are a messaging system that lets decoupled components get notified when specific events happen within the application. They are particularly useful for scenarios where you need to perform actions based on changes or events in other parts of your application, such as sending notifications, updating related data, or executing custom business logic.
How Signals Work
Django signals work by connecting a sender (the component that emits the signal) with a receiver (the function that gets executed when the signal is sent). The process is managed by the signal dispatcher, which handles the connection between signals and their respective receivers. This architecture allows for a clean separation of concerns, where the components that send signals don’t need to know about the components that handle them.
Key Components:
- Signal: An instance of the
django.db.models.signals.Signal
class that defines the type of message being sent. - Sender: The model or component responsible for sending the signal.
- Receiver: A function that is triggered when the signal is emitted and is responsible for handling the signal’s logic.
Common Use Cases for Django Signals
Django signals are commonly used in various scenarios, such as:
- Model Signals: Reacting to changes in model instances, like when an object is saved or deleted.
- Custom Signals: Creating your own signals to handle specific events in your application, such as user registration or payment processing.
Sending and Receiving Signals
Model Signals
Django provides several built-in signals that correspond to the lifecycle events of a model. These include:
- pre_save: Triggered just before a model’s
save
method is called. - post_save: Triggered immediately after a model’s
save
method is called. - pre_delete: Triggered just before a model’s
delete
method is called. - post_delete: Triggered immediately after a model’s
delete
method is called.
Example: Sending a Signal When a Model is Saved
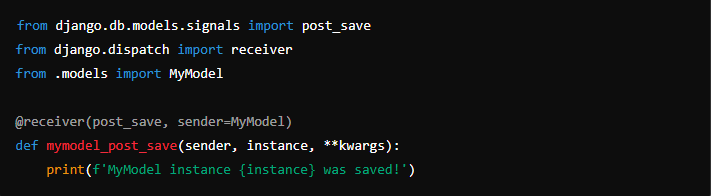
In this example, the mymodel_post_save
function is connected to the post_save
signal of the MyModel
model, allowing it to execute whenever an instance of MyModel
is saved.
Custom Signals
In addition to built-in signals, Django allows you to create custom signals for specific events in your application. This is useful for scenarios where you need to trigger actions that are not covered by Django’s built-in signals.
Example: Creating and Emitting a Custom Signal
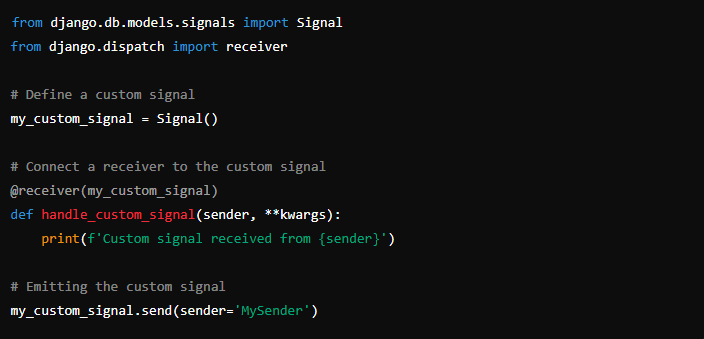
In this example, a custom signal my_custom_signal
is created and connected to the handle_custom_signal
receiver. The signal is then emitted using the send
method, which triggers the receiver function.
Creating Custom Signals
Defining and Connecting Custom Signals
Custom signals are defined using the Signal
class from django.db.models.signals
. Once defined, these signals can be connected to receiver functions that perform specific actions when the signal is triggered.
Example: Defining and Connecting a Custom Signal
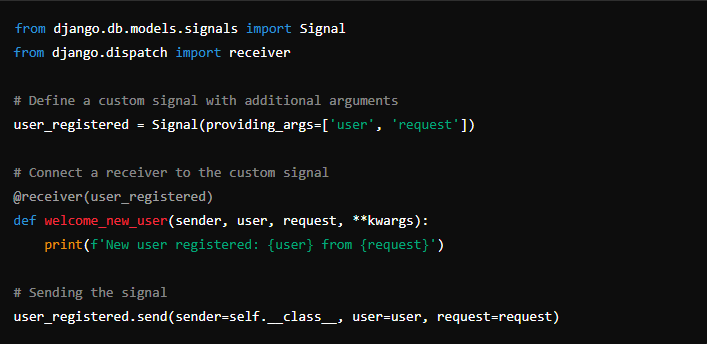
In this example, a user_registered
signal is defined and connected to the welcome_new_user
receiver. The signal is then sent with relevant data, such as the user
and request
, triggering the receiver function to execute.
Django signals are a flexible and powerful feature that can help you build more modular and maintainable applications. By understanding how to use both built-in and custom signals, you can efficiently manage inter-component communication and handle complex event-driven logic within your Django projects
Testing in Django
Introduction to Django Testing
Testing is an essential practice in Django development, ensuring that your application functions correctly and remains stable as you introduce new features or make changes. By writing comprehensive tests, you can identify bugs early, confirm that modifications don’t disrupt existing functionality, and document your application’s expected behavior.
Why Testing Is Important
Testing is crucial for maintaining the integrity of your application. It helps you:
- Identify Bugs Early: Catch issues before they reach production.
- Ensure Code Reliability: Verify that new code doesn’t break existing features.
- Provide Documentation: Serve as a living guide for how your application should behave under various conditions.
Types of Testing in Django
- Unit Testing: Focuses on testing individual components, such as models, views, and forms, in isolation from the rest of the application.
- Integration Testing: Ensures that different parts of the application work together as expected, testing the interaction between components.
- Functional Testing: Simulates the end-user experience to verify that the application behaves as expected from the user’s perspective.
Writing Unit Tests in Django
Django provides a built-in testing framework that extends Python’s unittest
module, allowing you to write and run tests easily. This framework includes a test client that can simulate HTTP requests and check the responses, making it ideal for testing views and the behavior of your web application.
Writing Unit Tests for Models
Unit tests for models verify that your data models behave as expected. For example, you might test that a model instance can be created and that its attributes are set correctly.
Example: Model Test Case
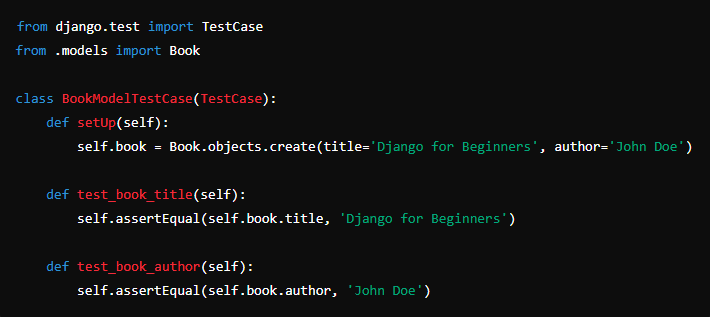
In this example, tests are written to ensure that the Book
model correctly stores and retrieves the title and author attributes.
Testing Views
View tests verify that your view functions or class-based views handle HTTP requests and responses correctly, ensuring that they return the expected status codes and content.
Example: View Test Case
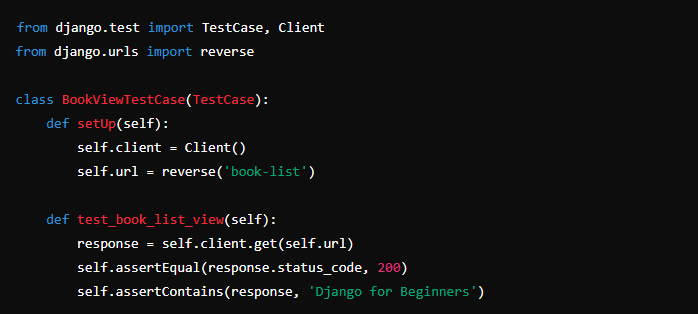
This test case checks that the book-list
view returns a 200 status code and that the response contains the expected content.
Testing Forms
Form tests ensure that your forms validate data correctly and behave as expected, preventing invalid data from being processed.
Example: Form Test Case
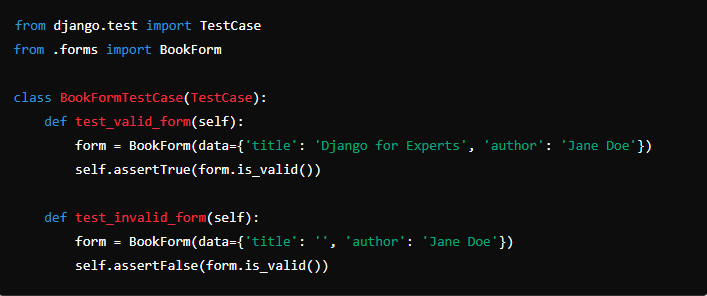
These tests check that a form validates input correctly, rejecting forms with missing required fields.
Integration Testing in Django
Integration tests ensure that the various components of your application work together as intended. These tests typically simulate user interactions, verifying that the entire workflow functions correctly from start to finish.
Example: Integration Test Case
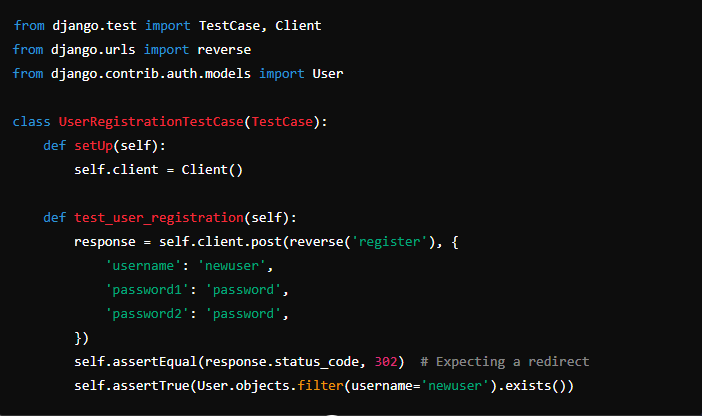
This integration test simulates a user registration process, checking that a new user can be successfully created and that the application redirects as expected.
Using Mocks and Fixtures
Mocks and fixtures are useful tools for setting up test data and simulating behavior in your tests.
Using Mocks
Mocks allow you to replace parts of your system during testing to isolate and test specific components without relying on the actual implementation.
Example: Using the unittest.mock
Library
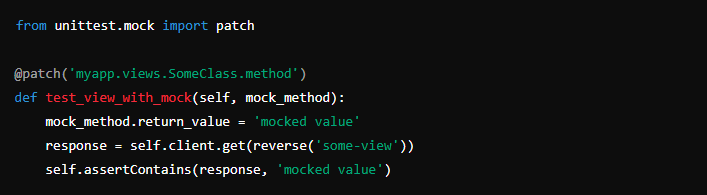
In this example, the method SomeClass.method
is mocked to return a specific value during the test, allowing you to focus on testing the view logic.
Using Fixtures
Fixtures provide predefined data that can be loaded into the database during testing, ensuring that your tests run with consistent data.
Example: Using Fixtures:
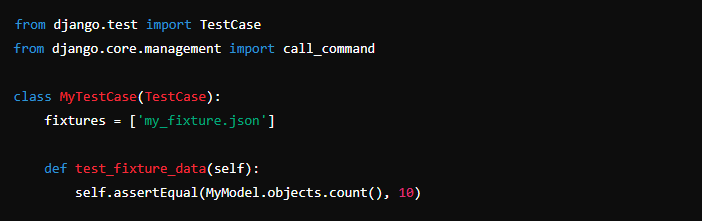
This test case loads data from a fixture file before running the tests, allowing you to verify that the data was loaded correctly and behaves as expected.
Testing in Django is a vital practice that helps ensure the robustness and reliability of your application. By leveraging Django’s built-in testing framework, along with tools like mocks and fixtures, you can write comprehensive tests that cover everything from individual components to entire workflows, giving you confidence that your application will perform correctly in production.
Deploying Django Applications
Preparing for Deployment
Environment-Specific Settings
- Introduction: Discuss the need for different configurations for development, staging, and production environments.
- Settings Management: Explain how to manage environment-specific settings using Django’s
settings.py
, environment variables, and.env
files. - Django-Environ: Introduce
django-environ
for managing environment variables and settings.
Security Best Practices
- Secret Keys: Emphasize the importance of keeping secret keys secure and not hardcoding them into source code. Discuss using environment variables for secret keys.
- Allowed Hosts: Explain the
ALLOWED_HOSTS
setting and its role in preventing HTTP Host header attacks. Provide guidance on configuringALLOWED_HOSTS
appropriately. - Other Security Considerations: Briefly touch on other security practices such as HTTPS, CSRF protection, and secure cookie settings.
Deployment on Various Platforms
Preparing for Deployment
Deploying a Django application requires careful preparation, especially when it comes to configuring environment-specific settings and ensuring security best practices are followed.
Environment-Specific Settings
Deploying an application to different environments—such as development, staging, and production—necessitates distinct configurations tailored to each environment’s specific needs.
- Introduction: Different environments often require unique settings to address their distinct requirements. For example, development may need detailed logging and debug tools, while production should prioritize security and performance.
- Settings Management: Managing these environment-specific settings can be done using Django’s
settings.py
file, where you can conditionally import settings based on the environment. Environment variables are also crucial, as they allow you to dynamically adjust settings without altering the source code. Tools like.env
files can store these variables, making them easy to manage and deploy. - Django-Environ: To streamline the management of environment variables, you can use the
django-environ
package. It helps to load environment variables from a.env
file and use them in your Django settings, making your configuration more flexible and secure.
Security Best Practices
Ensuring that your Django application is secure is a top priority during deployment. Here are some key considerations:
- Secret Keys: The secret key is a critical part of your Django project’s security, as it is used for cryptographic signing. Never hardcode the secret key into your source code. Instead, use environment variables to store and retrieve it securely.
- Allowed Hosts: The
ALLOWED_HOSTS
setting in Django is designed to prevent HTTP Host header attacks by specifying a list of host/domain names that the Django site can serve. Make sure to configure this setting correctly, listing only the domains that should serve your application. - Other Security Considerations: Beyond secret keys and allowed hosts, you should also enforce HTTPS for secure communication, enable CSRF protection to prevent cross-site request forgery, and configure secure cookie settings to enhance security.
Deployment on Various Platforms
Django can be deployed on several cloud platforms, each offering unique features and advantages. Below are some of the most popular options:
Deploying on AWS
Amazon Web Services (AWS) provides a robust infrastructure for deploying Django applications, with various services that can be utilized for a seamless deployment experience.
- Overview: AWS services like EC2 (Elastic Compute Cloud), RDS (Relational Database Service), and S3 (Simple Storage Service) are commonly used in Django deployments. EC2 handles the application server, RDS manages the database, and S3 is used for storing static and media files.
- Setup: Setting up a Django application on AWS involves launching an EC2 instance for your application, configuring RDS for your database, and setting up S3 for static and media file storage. AWS’s scalability and flexibility make it an excellent choice for handling varying traffic loads.
- Elastic Beanstalk: AWS Elastic Beanstalk simplifies the deployment process by managing the infrastructure for you. It’s an excellent option for developers who want to focus on code without worrying about the underlying infrastructure. Elastic Beanstalk handles the scaling, monitoring, and load balancing for your Django application.
Deploying on Heroku
Heroku is known for its ease of use, making it a popular choice for developers who want to deploy Django applications quickly.
- Overview: Heroku offers a streamlined deployment process, allowing you to deploy applications using Git. It’s ideal for small to medium-sized projects where simplicity and speed are key.
- Setup: Deploying a Django application on Heroku involves installing the Heroku CLI, setting up a PostgreSQL database add-on, and deploying your application via Git. Heroku takes care of the underlying infrastructure, making it a great option for developers who want to avoid manual server management.
Deploying on DigitalOcean
DigitalOcean is a cost-effective platform that provides flexible deployment options for Django applications.
- Overview: DigitalOcean offers Droplets (virtual private servers) that can be used to host Django applications. It’s an excellent choice for developers who want more control over their server environment.
- Setup: Setting up a Django application on DigitalOcean involves creating and configuring a Droplet, setting up your database, and managing static and media files. DigitalOcean also offers managed databases and storage options to simplify this process.
Setting Up a Production Server with Gunicorn and Nginx
For production deployments, it’s crucial to use a robust server setup that can handle incoming traffic efficiently.
- Gunicorn: Gunicorn (Green Unicorn) is a Python WSGI HTTP server that serves Django applications. To set it up, install Gunicorn and configure it to run your Django application. Gunicorn is designed to handle multiple requests simultaneously, making it ideal for production environments.
- Nginx: Nginx acts as a reverse proxy server, directing client requests to Gunicorn and serving static files. It also functions as a load balancer, distributing incoming requests across multiple Gunicorn processes. Configuring Nginx with Gunicorn involves setting up the appropriate server blocks and optimizing settings for security and performance.
- Configuration: Sample configuration files for Gunicorn and Nginx can help you get started. It’s important to fine-tune these configurations to balance performance and security, ensuring that your application can handle production traffic smoothly.
Continuous Integration and Deployment (CI/CD)
Implementing Continuous Integration and Deployment (CI/CD) pipelines is essential for automating the testing, building, and deployment processes in Django projects.
- Setting Up CI/CD Pipelines with Django: CI/CD automates the integration of code changes from multiple contributors, running tests, and deploying to production. This approach reduces manual intervention, speeds up the deployment process, and ensures code quality.
- Tools: Popular CI/CD tools like GitHub Actions, GitLab CI, and Jenkins can be integrated with Django projects. These tools automate various stages of development, from testing to deployment, making it easier to manage large projects.
- Pipeline Setup: Setting up a CI/CD pipeline involves configuring automated tests, defining build steps, and automating the deployment process. This setup ensures that every code change is tested and deployed consistently.
Monitoring and Logging
After deployment, it’s crucial to monitor your application’s performance and log any errors for quick diagnosis and resolution.
Setting Up Monitoring Tools
- Sentry: Sentry is a popular tool for error monitoring and performance tracking. It integrates seamlessly with Django to capture exceptions and performance metrics, helping you keep your application running smoothly.
- Prometheus: Prometheus is another powerful monitoring tool that can track application metrics and set up alerts. It’s particularly useful for monitoring the health and performance of your Django application.
Logging and Error Reporting
- Logging Configuration: Django’s logging framework can be configured to capture and manage logs, providing insights into application behavior and potential issues. Logs can be routed to various outputs, including files and external logging services.
- Error Reporting: Setting up error reporting tools and integrating them with external services can give you better visibility into your application’s health, enabling you to quickly identify and resolve issues.
Performance Optimization in Django
Optimizing performance is crucial for ensuring that your Django application runs efficiently and can handle varying loads effectively. This involves improving database performance, optimizing caching, managing static files, and implementing scalable solutions.
Database Optimization
Query Optimization and Indexing
- Overview: Efficient database queries are essential for high-performance applications. Unoptimized queries can lead to slow response times and increased load on the database.
- Indexing: Indexes are vital for speeding up query execution by allowing the database to quickly locate the necessary data. Properly creating and managing indexes on frequently queried fields can drastically improve performance.
- Database Profiling: Tools such as Django Debug Toolbar and database-specific profiling tools can help you analyze and optimize database queries. Profiling allows you to identify slow queries and optimize them for better performance.
Caching Strategies
Redis
- Overview: Redis is an in-memory data structure store that can be used for caching to enhance performance. It offers fast access to frequently used data, reducing the load on your database.
- Setup: Integrating Redis with Django involves configuring the cache backend and setting up Redis as the cache store. This can significantly improve the response time of your application.
Memcached
- Overview: Memcached is another caching solution that stores data in memory to reduce database load. It is useful for caching frequent queries and session data.
- Setup: To use Memcached with Django, you need to configure the cache backend in your settings and install the appropriate client libraries. Memcached is known for its simplicity and speed.
Cache Configuration
- Setup: Configuring caching mechanisms in Django involves setting up cache backends and defining cache settings in
settings.py
. Properly configured caching can greatly improve application performance by reducing redundant database queries and speeding up response times.
Optimizing Templates and Static Files
Compressing and Minifying Assets
- Static Files: Managing and optimizing static files (like CSS and JavaScript) is crucial for reducing load times and improving user experience. Techniques such as minification and concatenation help streamline asset delivery.
- Compression: Tools like Django Compressor, along with third-party services, can compress and minify static files to decrease their size and improve loading times. This reduces the amount of data transferred and speeds up page rendering.
Template Optimization
- Template Rendering: Efficient template rendering is key to reducing response times. Avoiding complex logic in templates and using template inheritance can improve performance.
- Template Caching: Implementing template caching allows Django to cache the output of rendered templates, reducing the time spent generating pages. This can be configured in your Django settings to cache whole views or parts of views.
Asynchronous Tasks and Background Processing
Using Celery for Background Tasks
- Introduction: Celery is a powerful tool for handling asynchronous tasks and background processing in Django. It allows you to offload time-consuming tasks from the request/response cycle, improving overall application performance.
- Setup: Setting up Celery involves installing the Celery package, configuring it with Django, and defining tasks that can be run asynchronously. Using Celery with a message broker like RabbitMQ or Redis allows for efficient task management.
Implementing WebSockets and Real-Time Features
WebSockets
- Overview: WebSockets provide a way to establish a persistent, real-time connection between the client and server. This is useful for applications that require live updates, such as chat applications or live notifications.
- Setup: Implementing WebSockets in Django typically involves using Django Channels, which extends Django to handle WebSockets and other real-time communication protocols.
Django Channels
- Overview: Django Channels is an extension that adds support for WebSockets and other asynchronous protocols. It integrates with Django’s existing architecture to provide real-time features and background task processing.
- Setup: Setting up Django Channels involves installing the package, configuring routing for WebSockets, and integrating it with Django’s ASGI interface.
Scaling Django Applications
Horizontal vs. Vertical Scaling
- Horizontal Scaling: This involves adding more instances of your application to handle increased load. Horizontal scaling distributes traffic across multiple servers, improving fault tolerance and handling higher traffic volumes.
- Vertical Scaling: Vertical scaling involves increasing the resources (CPU, RAM) of a single server. While it can improve performance, it has limitations and can be more costly compared to horizontal scaling.
Load Balancing and Handling High Traffic
- High Traffic Handling: To manage high traffic, it’s essential to implement strategies such as horizontal scaling, efficient caching, and load balancing. Ensuring that your application can handle peak loads without performance degradation is crucial.
- Load Balancers: Load balancers distribute incoming traffic across multiple servers to ensure that no single server is overwhelmed. They improve application availability and reliability by balancing the load and providing redundancy.
By applying these performance optimization techniques, you can ensure that your Django application runs efficiently, scales effectively, and provides a seamless experience for your users.
Security in Django
Securing web applications is crucial to protect user data and maintain the integrity of your system. Django offers robust tools and practices to safeguard your applications against common vulnerabilities. This section covers essential security practices, built-in features, HTTPS usage, and compliance with data protection regulations.
Common Security Practices
- Protecting Against SQL Injection SQL injection is a serious vulnerability that allows attackers to execute arbitrary SQL commands, potentially exposing or altering sensitive data. Django mitigates SQL injection risks through its ORM system:
- Use Django’s ORM: Django’s ORM automatically escapes parameters to prevent SQL injection. By utilizing ORM methods such as
.filter()
,.get()
, and.exclude()
, you can avoid raw SQL queries that might be prone to injection attacks. - Parameterized Queries: When raw SQL queries are necessary, use Django’s
connection.cursor()
to execute parameterized queries. This approach ensures that inputs are safely escaped, preventing injection.
- Use Django’s ORM: Django’s ORM automatically escapes parameters to prevent SQL injection. By utilizing ORM methods such as
- Protecting Against Cross-Site Scripting (XSS) XSS attacks involve injecting malicious scripts into web pages viewed by users, potentially compromising their data or interacting with the site on their behalf. Django provides several mechanisms to guard against XSS:
- Automatic HTML Escaping: By default, Django templates escape variables to prevent XSS. Ensure you use Django’s template language for outputting user data.
- Sanitize User Input: When you need to render user-provided HTML, use Django’s
mark_safe
carefully. Ensure that input is sanitized to avoid XSS. - Use Django’s Bleach Library: For more controlled HTML sanitization, integrate the
bleach
library, which provides fine-grained control over allowed HTML tags and attributes.
- Protecting Against Cross-Site Request Forgery (CSRF) CSRF attacks trick users into performing unwanted actions on a site where they are authenticated. Django includes built-in mechanisms to prevent CSRF attacks:
- CSRF Token Middleware: Django’s middleware automatically adds a CSRF token to forms. Include
{% csrf_token %}
in all forms to enable CSRF protection. - CSRF Exemptions: For APIs or non-form POST requests, you can use the
@csrf_exempt
decorator if necessary. However, be cautious with this approach to avoid reducing security.
- CSRF Token Middleware: Django’s middleware automatically adds a CSRF token to forms. Include
Django’s Built-in Security Features
- CSRF ProtectionDjango’s CSRF protection is a cornerstone of its security model:
- CSRF Middleware: Django includes
django.middleware.csrf.CsrfViewMiddleware
, which adds CSRF protection to your views. - Setting CSRF Cookies: Ensure that
CSRF_COOKIE_SECURE
is set toTrue
if you are using HTTPS to ensure the CSRF token is only sent over secure connections.
- CSRF Middleware: Django includes
- Secure Cookies Cookies are crucial for session management and other critical functions. Securing cookies helps protect against various attacks:
- Secure Cookies: Set
SESSION_COOKIE_SECURE
andCSRF_COOKIE_SECURE
toTrue
to ensure cookies are only transmitted over HTTPS. - HTTPOnly Cookies: Use
SESSION_COOKIE_HTTPONLY
to prevent JavaScript from accessing session cookies, which helps protect against XSS attacks. - SameSite Cookies: Configure
SESSION_COOKIE_SAMESITE
toStrict
orLax
to protect against CSRF by controlling how cookies are sent with cross-site requests.
- Secure Cookies: Set
- Other Security Features
- Content Security Policy (CSP): Implement CSP to restrict the sources from which scripts, styles, and other resources can be loaded, mitigating risks from unauthorized content.
- HTTP Security Headers: Configure HTTP headers such as
X-Frame-Options
andX-XSS-Protection
to enhance security.
Using HTTPS and SSL
- Enforcing HTTPS in DjangoHTTPS encrypts data transmitted between the server and clients. To enforce HTTPS:
- Redirect HTTP to HTTPS: Use
SECURE_SSL_REDIRECT
to automatically redirect HTTP requests to HTTPS. - HSTS: Implement HTTP Strict Transport Security (HSTS) to enforce HTTPS. Set
SECURE_HSTS_SECONDS
to a reasonable value, like one year. - Secure Cookies with HTTPS: Ensure cookies are only sent over HTTPS by setting
SESSION_COOKIE_SECURE
andCSRF_COOKIE_SECURE
toTrue
.
- Redirect HTTP to HTTPS: Use
Handling User Data and Privacy
- GDPR Compliance and Best Practices for Data ProtectionThe General Data Protection Regulation (GDPR) sets strict rules for handling personal data. Adhere to these best practices for compliance:
- Data Minimization: Collect only the data you need and process it only for necessary purposes.
- User Consent: Obtain explicit consent from users before collecting their data. Implement features that allow users to update or delete their data as needed.
- Data Encryption: Encrypt sensitive data both in transit and at rest to protect against unauthorized access.
- Data Subject Access Requests (DSARs): Implement processes to handle requests from users who wish to access, correct, or delete their data.
- Privacy Policy: Maintain a clear privacy policy that informs users about data collection, processing, and storage practices.
By incorporating these practices into your Django application, you can build a more secure and privacy-conscious web application. Regularly update your security measures to address new vulnerabilities and keep your application safe.
n today’s digital landscape, securing web applications is more crucial than ever. Django provides a comprehensive suite of tools and best practices to help developers protect their applications from a wide range of vulnerabilities. By leveraging Django’s built-in security features, such as its ORM for preventing SQL injection, automatic HTML escaping to guard against XSS, and robust CSRF protection, you can create a secure and resilient application environment.
Implementing additional security practices, such as enforcing HTTPS, using secure cookies, and adhering to data protection regulations like GDPR, further strengthens your application’s defenses. It’s essential to stay informed about emerging threats and continuously update your security strategies to address new challenges.
As you build and deploy Django applications, remember that security is an ongoing process. Regularly review and test your security measures, educate your team about best practices, and incorporate feedback from security audits. By doing so, you not only protect your users but also enhance the trust and reliability of your application.
Ultimately, a secure application is not just about implementing technical controls but also about fostering a culture of security awareness and vigilance. Embrace these practices, stay proactive, and ensure that security remains a top priority throughout your development lifecycle.
By following the guidance outlined in this article, you are well-equipped to safeguard your Django applications and deliver a secure, reliable experience for your users.