FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It’s designed to be easy to use and to offer high productivity while providing the best of both worlds: the simplicity of Python and the power of modern web standards.
What is FastAPI?
FastAPI is a web framework designed to simplify the development of APIs while ensuring performance is not compromised. It is built on top of Starlette for the web parts and Pydantic for the data parts, making it both lightweight and fast. FastAPI allows developers to leverage Python’s type annotations to automatically generate interactive API documentation and ensure robust data validation.
Some key characteristics of FastAPI include:
- Asynchronous Programming: Built with support for asynchronous programming, it leverages Python’s async and await capabilities, enabling you to build high-performance applications.
- Automatic Data Validation: Using Pydantic, FastAPI automatically validates request data and ensures that it adheres to the specified types.
- Interactive API Documentation: FastAPI generates automatic, interactive API documentation using OpenAPI (formerly Swagger) and ReDoc.
- Dependency Injection: It simplifies the management of dependencies, allowing developers to write cleaner and more modular code.
History and Background
FastAPI was created by Sebastián Ramírez in 2018. The framework emerged from the need for a high-performance web framework that combines the speed of asynchronous code execution with the ease of use and readability of Python. Ramírez’s vision was to create a framework that was not only fast and efficient but also developer-friendly, allowing for rapid development without sacrificing performance.
The development of FastAPI was heavily influenced by existing tools and frameworks:
- Starlette: FastAPI is built on Starlette, a lightweight ASGI framework that provides many of the underlying features such as routing, middleware, and sessions.
- Pydantic: FastAPI uses Pydantic for data validation and settings management. Pydantic’s use of Python type annotations allows FastAPI to automatically validate incoming request data, reducing the likelihood of errors.
FastAPI gained rapid popularity in the Python community due to its modern approach, leveraging the latest Python features, and its impressive performance benchmarks. It’s widely used in production environments at companies such as Microsoft, Netflix, and Uber.
Why FastAPI?
The growing popularity of FastAPI can be attributed to several factors that make it an appealing choice for developers:
- Performance and Speed:
- High Throughput: FastAPI is one of the fastest Python web frameworks available. It’s comparable in performance to frameworks like Node.js and Go, thanks to its support for asynchronous programming and efficient underlying architecture.
- Async and Sync Support: FastAPI supports both synchronous and asynchronous request handling, allowing developers to write high-performance APIs without dealing with complex threading or multiprocessing issues.
- Ease of Use:
- Minimal Boilerplate: FastAPI reduces boilerplate code by automating many common tasks, such as request validation, serialization, and documentation generation.
- Pythonic Design: FastAPI’s design follows Python’s philosophy of simplicity and readability. It uses Python’s type hints extensively, making the code more understandable and maintainable.
- Intuitive Development: With built-in support for dependency injection and validation, FastAPI encourages writing clean and modular code, which is easier to test and maintain.
- Modern Pythonic Features:
- Type Hints: FastAPI leverages Python’s type hinting system to perform runtime type checking and validation. This leads to fewer runtime errors and more predictable behavior.
- Automatic Documentation: FastAPI generates interactive API documentation with OpenAPI and ReDoc, making it easier for developers to explore and test their APIs.
- Data Validation: Pydantic models ensure that the data sent to your API meets the required schema, catching errors early in the development process.
Comparison with Other Frameworks
FastAPI’s approach and feature set distinguish it from other popular Python web frameworks, including Django, Flask, and Falcon.
- Django:
- Overview: Django is a full-stack web framework that includes everything you need to build a web application, from ORM to templating to authentication.
- Strengths: Django’s “batteries-included” approach provides a comprehensive set of tools for building complex web applications. It’s well-suited for projects where developers need a lot of built-in functionality.
- Comparison: FastAPI, in contrast, is focused specifically on building APIs. While Django is great for full-fledged web applications, FastAPI excels in performance and simplicity, especially for API-centric projects. Django is synchronous by nature, which can be a limitation for highly concurrent workloads.
- Flask:
- Overview: Flask is a micro-framework that provides the bare minimum needed to build web applications. It’s known for its simplicity and flexibility.
- Strengths: Flask’s lightweight nature makes it easy to extend and customize. It’s a popular choice for small to medium-sized projects or when you need to build a simple API quickly.
- Comparison: FastAPI offers many of the same benefits as Flask, such as simplicity and flexibility, but with additional features like automatic validation and async support. FastAPI’s performance outpaces Flask, especially in high-concurrency scenarios. Flask’s ecosystem is more mature, but FastAPI is catching up rapidly in terms of community support and available extensions.
- Falcon:
- Overview: Falcon is a high-performance web framework designed specifically for building fast APIs. It’s known for its minimalism and speed.
- Strengths: Falcon is lightweight and extremely fast, making it a good choice for building microservices or highly performant APIs.
- Comparison: FastAPI and Falcon share a focus on performance, but FastAPI’s ease of use and additional features, like automatic documentation and dependency injection, give it an edge in developer productivity. Falcon might still be the choice for extremely minimalistic setups where every bit of performance counts, but FastAPI’s balance of speed and usability makes it more attractive for most use cases.
FastAPI not only a strong contender in performance-driven applications but also a more developer-friendly option, particularly for projects that demand both efficiency and ease of use.
2. Setting Up FastAPI
Setting up FastAPI is straightforward, but understanding the underlying structure and how to properly configure your development environment can significantly enhance your productivity. In this section, we’ll walk through the installation process, explore the basic project structure, and create a simple “Hello World” application. We’ll also discuss how to run your FastAPI server efficiently using Uvicorn, a lightning-fast ASGI server.
Installation
FastAPI requires Python 3.7 or higher, as it heavily relies on type hints and asynchronous capabilities that are fully supported only in these versions. Before starting, ensure that Python is installed on your machine and that it’s updated to at least version 3.7.
Step 1: Setting Up a Virtual Environment
It’s a good practice to create a virtual environment for your FastAPI project. This helps in managing dependencies and avoids conflicts between different Python projects on your machine.
To create a virtual environment, run:

Activate the virtual environment:
- On macOS/Linux:
- source env/bin/activate
- On Windows:
- .\env\Scripts\activate
Once the environment is activated, you’ll see the (env)
prefix in your terminal.
Step 2: Installing FastAPI and Uvicorn
FastAPI doesn’t come with its own server, so you’ll need to install an ASGI server to run your application. Uvicorn is the most commonly used server with FastAPI due to its high performance.
Install FastAPI and Uvicorn using pip:

This command installs the latest versions of FastAPI and Uvicorn. You can specify versions if needed, but generally, it’s advisable to work with the latest stable releases to take advantage of new features and performance improvements.
Basic Project Structure
Organizing your FastAPI project correctly from the beginning can save you a lot of refactoring later. Here’s a basic structure that’s scalable and follows best practices:
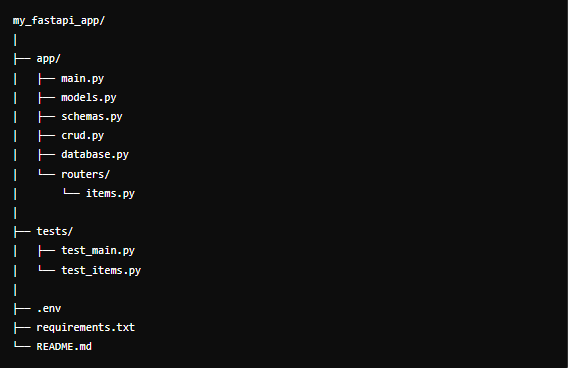
- app/main.py: This is the entry point of your application where you’ll define the FastAPI instance and include your routers.
- app/models.py: Contains the SQLAlchemy models (if using a relational database) or any other models representing your data structure.
- app/schemas.py: Holds the Pydantic models used for data validation and serialization.
- app/crud.py: This module typically contains the functions to interact with the database, abstracting the CRUD operations (Create, Read, Update, Delete).
- app/database.py: Manages the database connection and session, often configured with SQLAlchemy.
- app/routers/: This directory contains different route modules, helping you to keep your routes organized by functionality.
- tests/: Holds your test files. Using separate files for different functionalities helps maintain a clean test suite.
- .env: A file to store environment variables like database URLs, secret keys, etc.
- requirements.txt: Lists the dependencies of your project, which can be installed with pip.
- README.md: Contains documentation for your project.
This structure ensures that your application is modular, making it easier to scale and maintain as the project grows.
Hello World Example
Now, let’s dive into coding a simple “Hello World” application using FastAPI. This example will introduce you to the basics of FastAPI, including creating an instance of FastAPI, defining routes, and returning responses.
Create a file named main.py
inside the app/
directory:
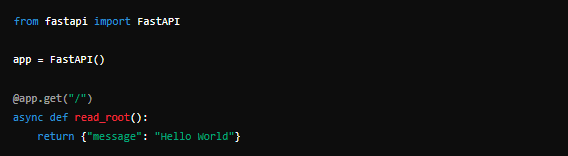
Here’s a breakdown of what’s happening in the code:
- from fastapi import FastAPI: Imports the FastAPI class.
- app = FastAPI(): Creates an instance of the FastAPI class. This instance will be the main point of interaction for defining your routes.
- @app.get(“/”): This is a decorator that maps the function
read_root
to the path/
with a GET request. FastAPI uses these decorators to link routes to functions. - async def read_root(): Defines an asynchronous function that handles the GET request at the root URL (
/
). The function returns a dictionary, which FastAPI automatically converts to JSON.
When this function is called, FastAPI will return the JSON object {"message": "Hello World"}
as the response.
Running a FastAPI Server
To run your FastAPI application, you need to use an ASGI server like Uvicorn. Let’s run the server and see the “Hello World” application in action.
Navigate to the root of your project and run the following command:

Here’s what the command does:
- app.main: This tells Uvicorn to find the
app
object in themain.py
file inside theapp
directory. - –reload: This option enables the auto-reload feature, which automatically restarts the server whenever you make changes to your code. This is very useful during development.
Once the server starts, you should see output similar to this:

Visit http://127.0.0.1:8000
in your browser, and you should see the “Hello World” message in JSON format.
Interactive API Documentation
One of the coolest features of FastAPI is its automatic documentation. While the server is running, you can access the interactive API documentation at:
- Swagger UI:
http://127.0.0.1:8000/docs
- ReDoc:
http://127.0.0.1:8000/redoc
These documentation interfaces allow you to explore and test your API endpoints interactively, making it easier to develop and debug your application.
Using Uvicorn as an ASGI Server
Uvicorn is an ASGI (Asynchronous Server Gateway Interface) server that is optimized for handling asynchronous Python web applications, including FastAPI. It’s lightweight, fast, and capable of handling a large number of concurrent connections, making it an excellent choice for production deployments.
Why Uvicorn?
- Performance: Uvicorn is highly optimized and uses modern Python features like async/await, which makes it suitable for high-performance web applications.
- ASGI Support: Unlike WSGI (used by frameworks like Flask and Django), ASGI is designed for asynchronous frameworks. This allows you to handle WebSockets, background tasks, and long-lived connections efficiently.
- Scalability: Uvicorn’s ability to handle asynchronous I/O makes it scalable for applications that require handling many simultaneous requests.
Running Uvicorn in Production
In a production environment, you typically want to run Uvicorn with more robust settings. Here’s an example of how to run Uvicorn with multiple workers:

Explanation:
- –host 0.0.0.0: This option binds the server to all available IP addresses, making it accessible over the network.
- –port 8000: Specifies the port number. You can change this if needed.
- –workers 4: Runs 4 worker processes. Increasing the number of workers can improve performance by handling more requests in parallel.
Using Gunicorn with Uvicorn
For better process management in production, you can use Gunicorn in combination with Uvicorn:

Gunicorn provides better process management, monitoring, and signal handling, while Uvicorn remains responsible for handling the ASGI protocol.
Configuring Environment Variables
For a production-ready application, managing environment variables is crucial. FastAPI doesn’t handle environment variables directly, but you can use Python’s built-in os
module or external packages like python-dotenv
to manage them.
Create a .env
file in your project root:

In your FastAPI application, you can load these variables using the os
module:
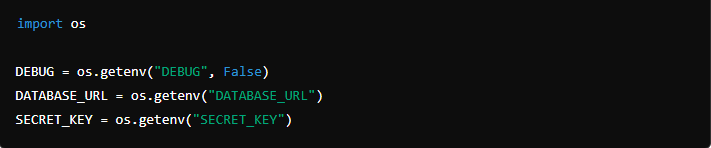
Then, load the environment variables in your main.py
:
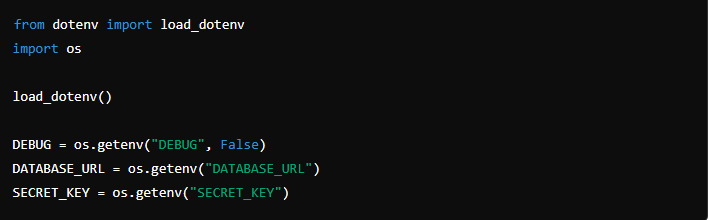
Customizing Uvicorn with Configuration Files
For larger projects, you might prefer to manage Uvicorn’s configuration with a configuration file rather than command-line arguments. Create a file named uvicorn_config.py
:

Run Uvicorn with the configuration file:

Setting up FastAPI is simple yet powerful, allowing you to create high-performance web applications quickly. By following best practices in project structure, understanding the basics of running a FastAPI server with Uvicorn, and effectively managing your environment variables, you can build scalable and maintainable applications. The “Hello World” example we covered is just the beginning; FastAPI’s capabilities extend far beyond simple use cases, making it a versatile choice for a wide range of web applications.
3. Core Concepts
Understanding FastAPI’s core concepts is crucial for building robust and scalable web applications. These concepts form the foundation of FastAPI’s functionality, enabling you to handle HTTP requests, validate data, manage dependencies, and execute background tasks efficiently. In this section, we’ll explore key concepts such as path operations, request and response models, Pydantic for data validation, dependency injection, and background tasks.
Path Operations
In FastAPI, path operations are the core of how you define the API endpoints and handle different HTTP methods. Each path operation corresponds to a specific route in your application and is tied to a function that handles the request.
GET, POST, PUT, DELETE
FastAPI supports all the standard HTTP methods, including GET, POST, PUT, DELETE, and more. These methods are crucial for building RESTful APIs, where each method represents a different action on a resource.
- GET: Retrieves data from the server. This is typically used for fetching resources.
- POST: Submits new data to the server. This is used for creating new resources.
- PUT: Updates an existing resource. It usually requires the full payload of the resource to be updated.
- DELETE: Removes a resource from the server.
Here’s how you can define these operations in FastAPI:
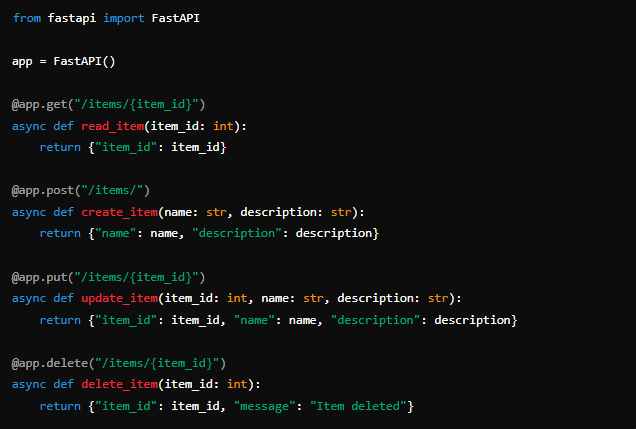
Path Parameters
Path parameters are dynamic parts of a URL, allowing you to capture values specified in the path. These parameters are defined by placing them in curly braces {}
within the path.
For example:

In this example, when you navigate to /users/5
, FastAPI captures the value 5
and passes it as the user_id
parameter to the get_user
function.
Type Annotations in Path Parameters
FastAPI uses Python’s type hints to validate path parameters. By specifying a type (e.g., int
, str
), FastAPI automatically validates and converts the parameter before it’s passed to the function.

Here, item_id
is expected to be an integer. If a user tries to access the URL /items/foo
, FastAPI will return a 422 Unprocessable Entity error because foo
is not a valid integer.
Path Parameter Validation
You can also add more complex validation using Path
from FastAPI, which allows you to set constraints on the parameters.

In this example, the item_id
must be a positive integer, and FastAPI will automatically enforce this constraint.
Query Parameters
Query parameters are optional and are used to filter or modify the request. They are appended to the URL after a ?
, and each parameter is separated by an &
. In FastAPI, query parameters are defined as function arguments that are not part of the path.
For example:

Here, skip
and limit
are query parameters. You can access them like this: /items/?skip=5&limit=20
.
Optional Query Parameters
Query parameters can be optional, with default values set in the function signature. If the user doesn’t provide a value, FastAPI uses the default.

In this example, if the user doesn’t specify skip
or limit
, the default values of 0
and 10
will be used.
Query Parameter Validation
Like path parameters, you can use the Query
function from FastAPI to add validation constraints to query parameters.
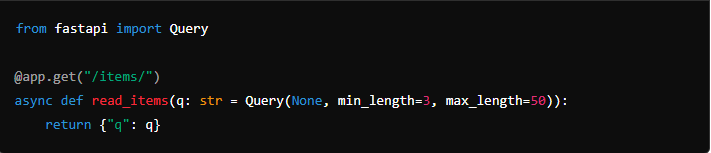
Here, q
is a query parameter that must be between 3 and 50 characters long. If the input doesn’t meet these criteria, FastAPI will return a 422 error.
Request and Response Models
FastAPI leverages Pydantic models to validate and serialize data. These models are used in both request and response bodies, ensuring data consistency and validation.
Pydantic Models
Pydantic is a powerful data validation and parsing library used by FastAPI. It allows you to define data models with type annotations, and FastAPI will automatically validate the incoming data against these models.
Here’s an example of a Pydantic model:
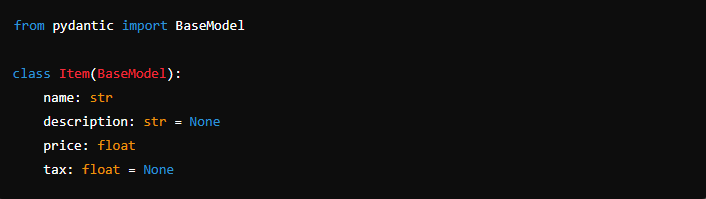
This Item
model can be used to validate incoming JSON data in a POST request:

When a client sends a POST request to /items/
with a JSON body, FastAPI will validate the data against the Item
model. If the data is valid, it will be passed to the create_item
function as an item
object.
Request Body
The request body in FastAPI is defined using Pydantic models. When you expect data in the request body, you use a Pydantic model as a parameter in your endpoint function.
For example:

The request body must include the fields defined in the Item
model. If any field is missing or doesn’t match the expected type, FastAPI will return a 422 error.
Response Model
You can also specify the response model, ensuring that the data returned by your API is structured and validated according to the model. This helps maintain consistency in the API’s responses.

In this example, FastAPI will validate the returned data against the Item
model before sending it back to the client. If the returned data doesn’t match the model, FastAPI will raise an error.
Response Model Aliasing
Sometimes, you might want to expose a different field name in the response than what’s used internally. Pydantic allows you to define alias names for fields.
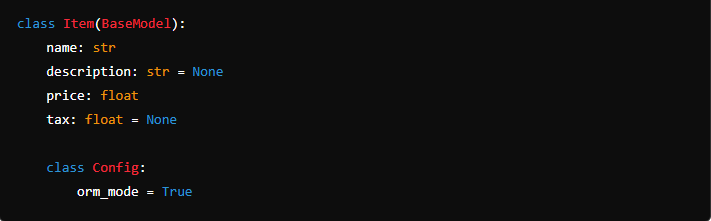
With orm_mode
set to True
, Pydantic models can accept SQLAlchemy objects, making it easier to integrate with databases.
Data Validation
Data validation is one of the core features of FastAPI, thanks to its deep integration with Pydantic. FastAPI automatically validates incoming data based on the types and constraints you define in your Pydantic models.
Field Types and Constraints
Pydantic supports a wide range of field types, including strings, integers, floats, lists, and more. You can also apply constraints such as minimum and maximum lengths, regular expressions, and more.
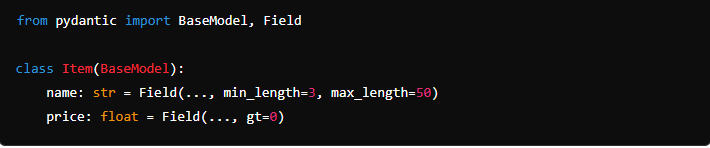
In this example, name
must be between 3 and 50 characters long, and price
must be greater than zero.
Custom Validators
Pydantic also allows you to create custom validators for more complex validation logic. You can define a custom method in your model and use the @validator
decorator to apply it to a field.
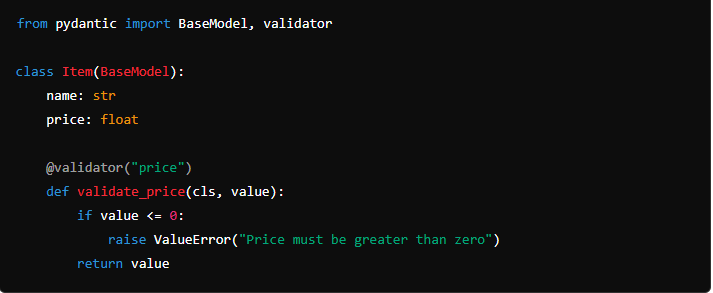
This custom validator ensures that the price
field is always greater than zero.
Nested Models
Pydantic models can be nested, allowing you to define complex data structures. This is particularly useful when your API deals with JSON objects that have nested objects.
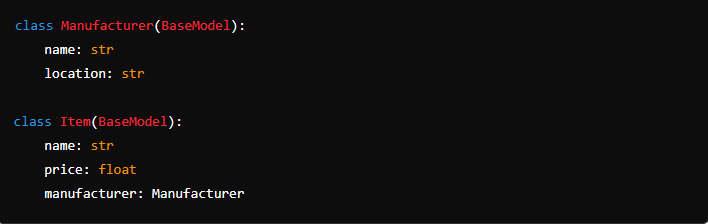
In this example, the Item
model includes a nested Manufacturer
model, which validates the nested object structure.
Dependency Injection
Dependency injection is a powerful feature in FastAPI that allows you to inject dependencies into your endpoints in a clean and maintainable way. Dependencies can be anything from database connections to authentication mechanisms.
Defining Dependencies
In FastAPI, you define dependencies using Python functions and the Depends
function. The Depends
function tells FastAPI to call the dependency function and pass its return value to your endpoint function.
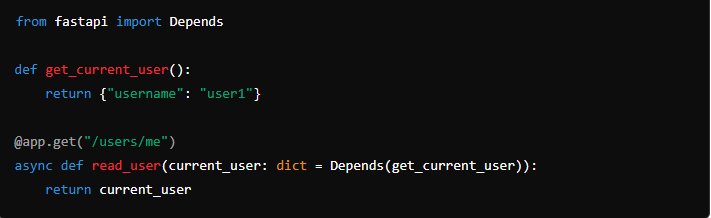
Here, get_current_user
is a dependency that is injected into the read_user
endpoint. The return value of get_current_user
is passed as the current_user
parameter to read_user
.
Handling Dependencies with Classes
Dependencies can also be classes, which is useful for more complex scenarios like database connections. The class is instantiated once, and the instance is reused across requests.
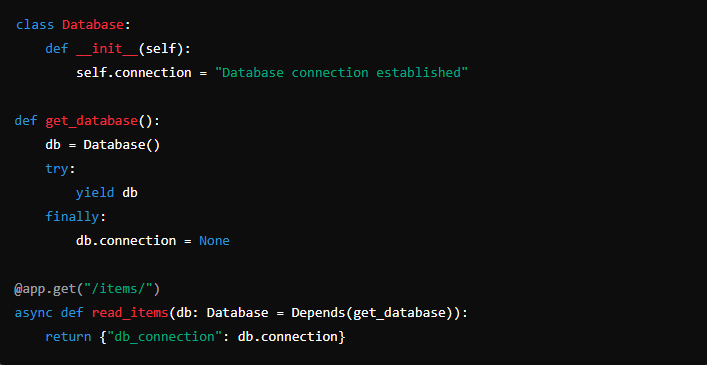
In this example, get_database
is a dependency that creates a Database
instance, which is then used in the read_items
endpoint.
Dependency Injection in Sub-Applications
FastAPI allows you to apply dependencies to entire sub-applications, making it easier to manage dependencies across multiple endpoints.
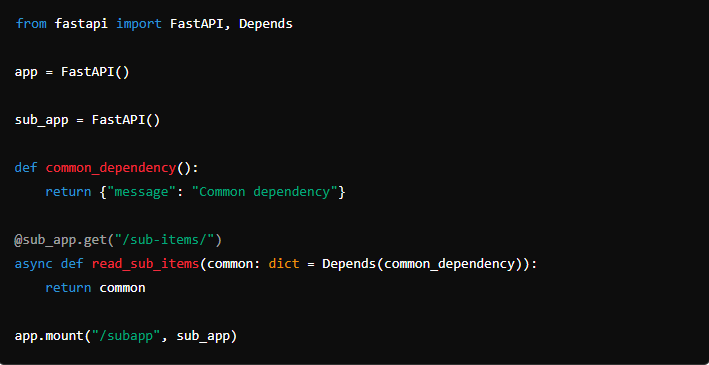
In this example, the common_dependency
is applied to all endpoints within the sub_app
, simplifying dependency management.
Background Tasks
Background tasks in FastAPI allow you to perform operations asynchronously without blocking the main thread. This is useful for tasks like sending emails, processing files, or any other long-running operation that doesn’t need to be completed before returning a response to the client.
Using Background Tasks
FastAPI provides a BackgroundTasks
class to manage background tasks. You can define a function to run in the background and pass it to BackgroundTasks
.
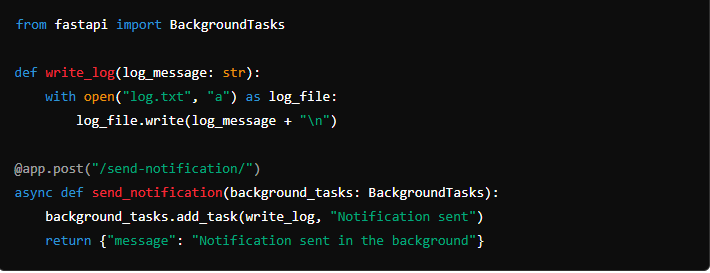
In this example, the write_log
function runs in the background after the send_notification
endpoint is called, allowing the endpoint to return a response immediately.
Chaining Background Tasks
You can also chain multiple background tasks together. FastAPI will execute them sequentially in the order they were added.
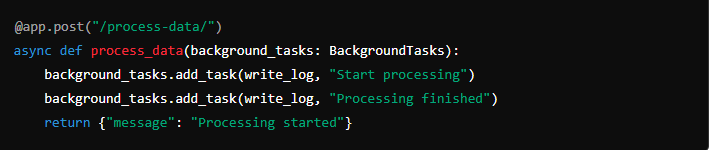
In this example, two log entries are written in sequence, ensuring that tasks are completed in order.
Using Background Tasks with Dependencies
Background tasks can also be used in conjunction with dependencies. This allows you to execute background tasks that rely on dependency injection.

In this example, the write_log
function logs a message that includes a dependency, demonstrating how background tasks can integrate with other FastAPI features.
By mastering these core concepts—path operations, request and response models, data validation, dependency injection, and background tasks—you can leverage FastAPI to build highly efficient and maintainable web applications. The flexibility and power of FastAPI’s core features make it a top choice for modern Python web development.
4. Advanced Features in FastAPI
FastAPI is a modern web framework for building APIs with Python, offering a range of advanced features that cater to complex application requirements. This section delves into these advanced features, including asynchronous support, middleware, custom request and response handling, form data and file uploads, error handling, security features, WebSockets, database integration, and more. By mastering these features, you’ll be equipped to build scalable, secure, and performant APIs.
Asynchronous Support
One of FastAPI’s most powerful features is its native support for asynchronous programming. Asynchronous code allows your application to handle multiple tasks simultaneously, making it more efficient, especially when dealing with I/O-bound operations like database queries or API calls.
Async and Await in FastAPI
FastAPI leverages Python’s async
and await
syntax to write asynchronous code. This is particularly useful when you have multiple I/O operations that can run concurrently, such as reading from a database, making external API requests, or processing files.
Here’s a simple example of how to use async
and await
in FastAPI:
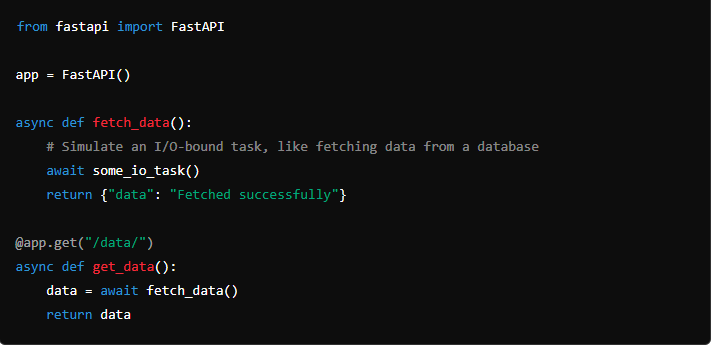
In this example, the fetch_data
function is an asynchronous function that performs an I/O-bound task. The await
keyword is used to pause the execution of get_data
until fetch_data
completes. This non-blocking behavior allows other tasks to be processed concurrently, improving the overall performance of your application.
Middleware
Middleware in FastAPI allows you to execute code before and after each request. It’s a powerful feature for handling cross-cutting concerns like logging, authentication, or modifying the request and response objects.
Creating Custom Middleware
To create custom middleware, you define a function that takes request
, call_next
, and response
as parameters. This function can modify the request before passing it to the next middleware or route handler, and it can also modify the response before sending it back to the client.
Here’s an example:
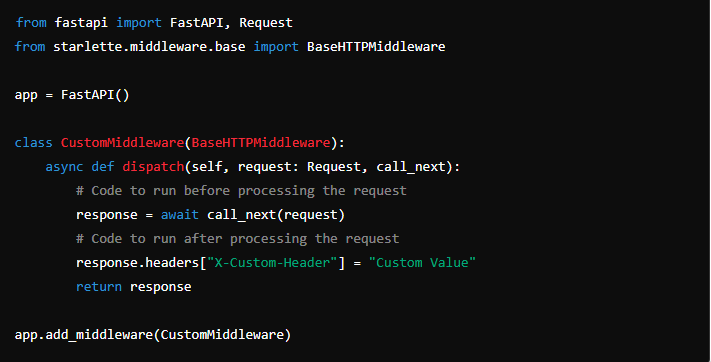
In this example, the CustomMiddleware
class intercepts all incoming requests and outgoing responses. You can add any logic you need in the dispatch
method, such as logging, modifying headers, or even blocking certain requests.
Built-in Middleware
FastAPI also provides built-in middleware for handling common tasks like CORS (Cross-Origin Resource Sharing), GZip compression, and more.
For example, to enable CORS in your application:
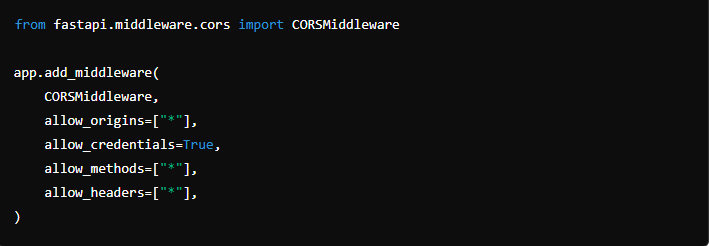
This enables CORS for all origins, allowing your API to be accessed from any domain.
Custom Request and Response Classes
FastAPI allows you to customize the request and response classes to suit your application’s needs. This is particularly useful when you need to handle complex data formats or implement custom serialization and deserialization logic.
Custom Request Classes
You can define custom request classes by inheriting from FastAPI’s Request
class. This allows you to add additional methods or properties to the request object.
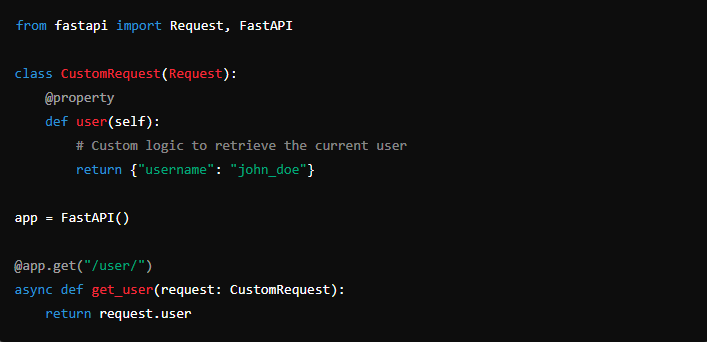
In this example, the CustomRequest
class adds a user
property to the request object, which can be accessed in your route handlers.
Custom Response Classes
Similarly, you can define custom response classes by inheriting from FastAPI’s Response
class. This allows you to customize how responses are formatted and sent back to the client.
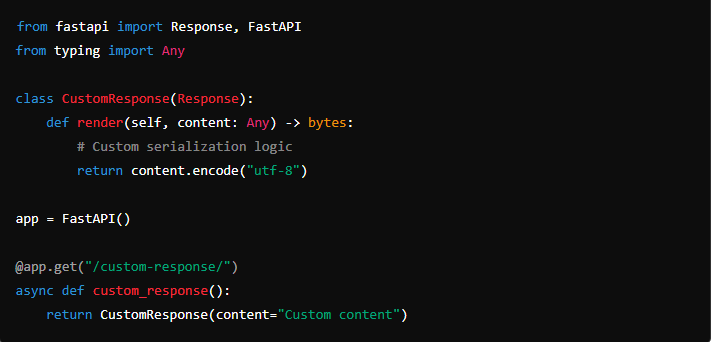
In this example, the CustomResponse
class overrides the render
method to customize how the response content is serialized.
Form Data and File Uploads
FastAPI provides robust support for handling form data and file uploads, making it ideal for building web applications that require file handling, such as image uploaders or document processors.
Handling Form Data
Form data is typically sent using the application/x-www-form-urlencoded
or multipart/form-data
media types. FastAPI makes it easy to handle these types of data using the Form
class.
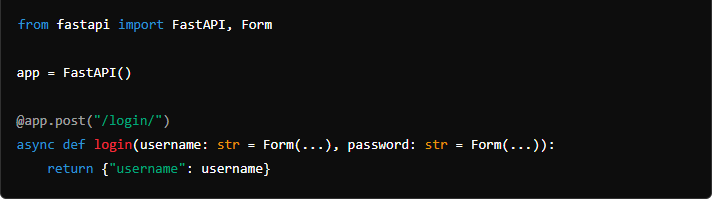
In this example, the Form
class is used to define form fields for the username
and password
parameters. FastAPI automatically parses the form data and populates these parameters.
Handling File Uploads
FastAPI also supports file uploads via the File
class, which is used to handle files sent with the multipart/form-data
media type.
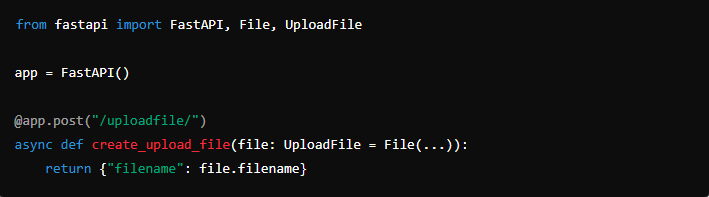
In this example, the UploadFile
class is used to handle the uploaded file. It provides methods to read the file content and access its metadata.
Saving Uploaded Files
To save an uploaded file to the server, you can use the UploadFile
class’s methods to read the file content and write it to a file on the server.
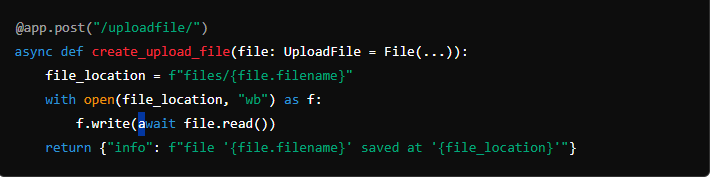
This example saves the uploaded file to the files/
directory on the server.
Handling Errors and Exceptions
FastAPI provides robust mechanisms for handling errors and exceptions, ensuring that your API behaves predictably even when things go wrong. This includes custom exception handlers, validation errors, and more.
Automatic Validation Errors
FastAPI automatically validates incoming requests based on your Pydantic models and type annotations. If the validation fails, FastAPI returns a 422 Unprocessable Entity response with details about the error.
For example:
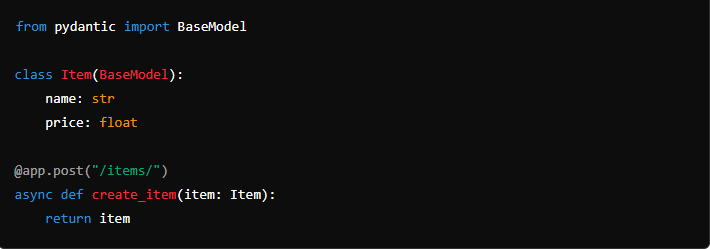
If a client sends a request with invalid data, FastAPI will automatically return an error response:
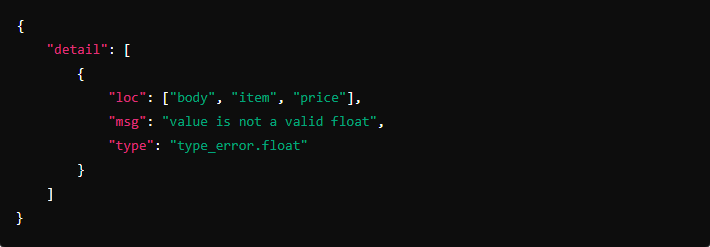
Custom Exception Handlers
You can define custom exception handlers to manage specific types of errors in your application. This is useful for handling custom exceptions or providing more user-friendly error messages.
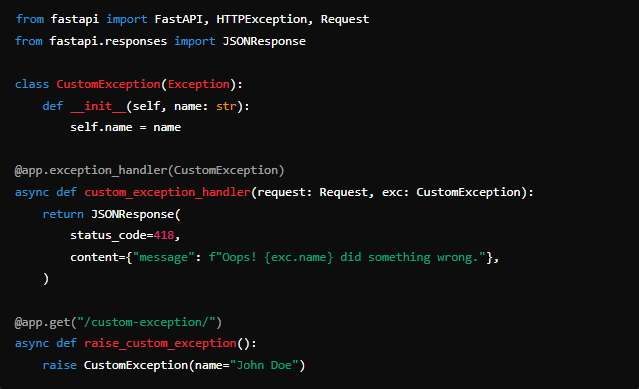
In this example, the CustomException
class defines a custom exception, and the custom_exception_handler
function handles it, returning a custom error message and status code.
Handling HTTP Exceptions
FastAPI also allows you to raise HTTP exceptions directly in your route handlers using the HTTPException
class. This is useful for handling common HTTP errors like 404 Not Found or 403 Forbidden.
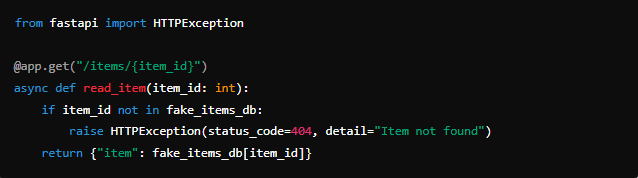
In this example, if the item_id
is not found in the database, FastAPI raises a 404 Not Found exception.
CORS (Cross-Origin Resource Sharing)
CORS is a security feature implemented by browsers to restrict web applications from making requests to a different domain than the one that served the web page. FastAPI provides built-in middleware to handle CORS.
Enabling CORS
To enable CORS in your FastAPI application, you can use the CORSMiddleware
class.
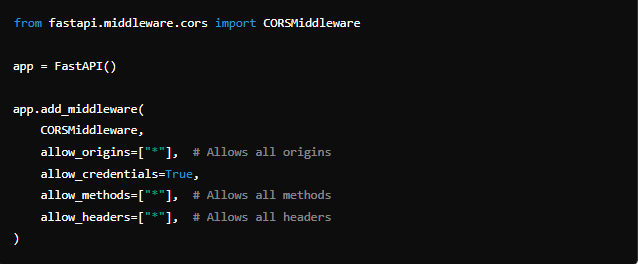
In this example, CORS is enabled for all origins, methods, and headers. This is useful during development but should be configured more restrictively in production.
Security Features
FastAPI provides robust security features out-of-the-box, including support for OAuth2, JWT authentication, and more. These features are essential for securing your APIs and ensuring that only authorized users can access them.
OAuth2 and JWT Authentication
OAuth2 is a widely used authorization framework that allows third-party applications to grant limited access to user accounts. FastAPI’s OAuth2 support is built-in and can be easily integrated into your application.
Using OAuth2 with Password and Bearer
The OAuth2PasswordBearer
class is used to handle OAuth2 with password and bearer token authentication. This is a common approach where the user provides a username and password to obtain a token, which is then used for subsequent requests.
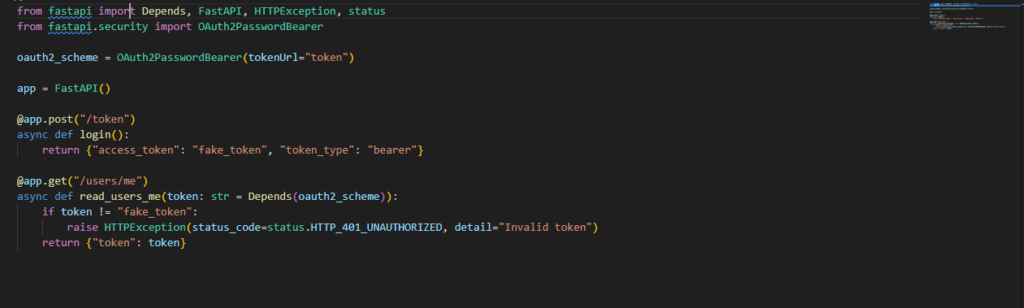
In this example, the oauth2_scheme
dependency extracts the token from the request header, and the read_users_me
endpoint verifies the token before granting access.
Rate Limiting and Throttling
Rate limiting and throttling are crucial for preventing abuse and ensuring fair use of your API. FastAPI doesn’t provide built-in rate limiting, but you can implement it using middleware or third-party libraries like slowapi
or fastapi-limiter
.
Using SlowAPI for Rate Limiting
SlowAPI
is a popular library for adding rate limiting to FastAPI applications. It integrates seamlessly with FastAPI, allowing you to define rate limits per endpoint or globally.
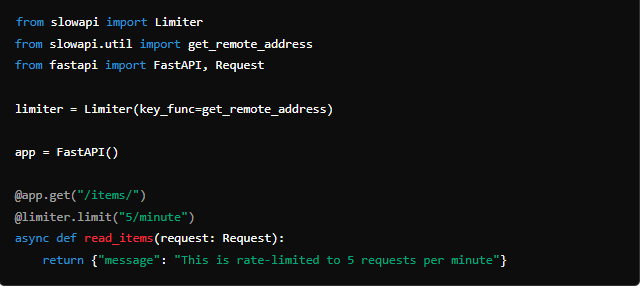
In this example, the read_items
endpoint is rate-limited to 5 requests per minute per IP address.
WebSockets in FastAPI
WebSockets are a protocol for full-duplex communication channels over a single TCP connection. FastAPI provides first-class support for WebSockets, making it easy to implement real-time features like chat applications, live notifications, and more.
Creating a WebSocket Endpoint
To create a WebSocket endpoint in FastAPI, use the WebSocket
class and the websocket_route
decorator.
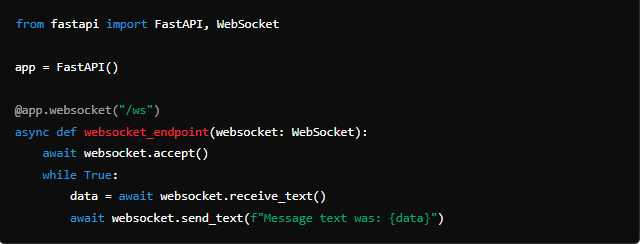
In this example, the WebSocket server accepts connections from clients, receives text messages, and sends responses back to the clients.
Handling Multiple Clients
FastAPI also allows you to handle multiple WebSocket connections simultaneously, which is useful for building chat applications or other real-time services.
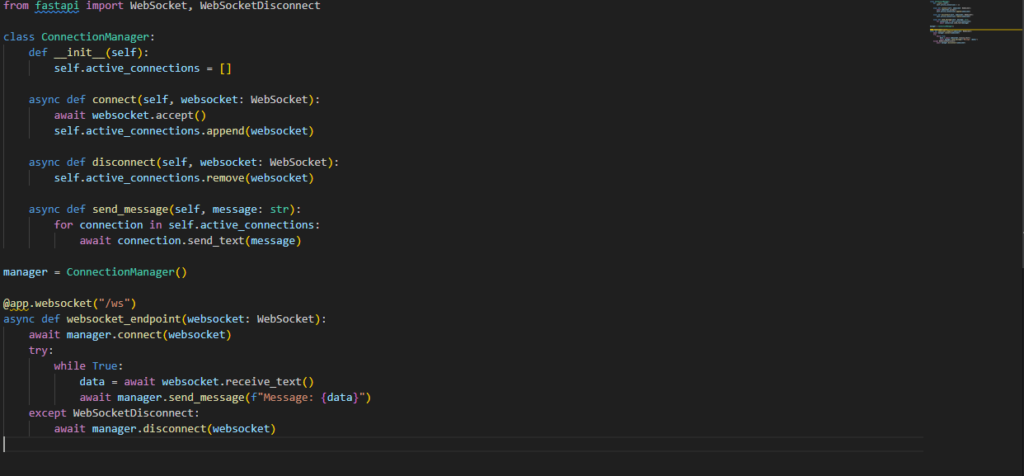
In this example, the ConnectionManager
class manages multiple WebSocket connections, allowing the server to broadcast messages to all connected clients.
Database Integration
FastAPI supports integration with various databases through SQLAlchemy, Tortoise ORM, and other ORMs. This flexibility allows you to choose the best database solution for your application’s needs.
Using SQLAlchemy
SQLAlchemy is a powerful and popular ORM (Object-Relational Mapping) library for Python. It allows you to interact with your database using Python classes and objects instead of raw SQL queries.
Setting Up SQLAlchemy with FastAPI
To set up SQLAlchemy with FastAPI, you’ll need to define your database models, create a database session, and connect it to your FastAPI application.
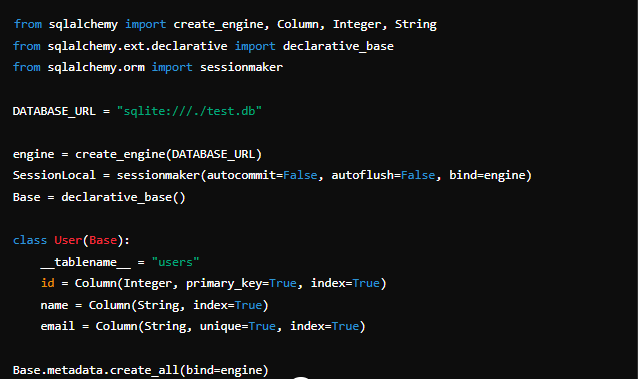
In this example, the User
class defines a database model for the users
table. The Base.metadata.create_all
function creates the table in the database.
Creating a Database Dependency
You can create a database dependency to inject a database session into your route handlers.
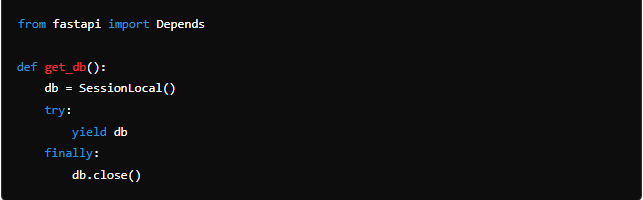
This get_db
function returns a database session that can be used in your route handlers.
CRUD Operations with SQLAlchemy
You can perform CRUD (Create, Read, Update, Delete) operations using the SQLAlchemy session.
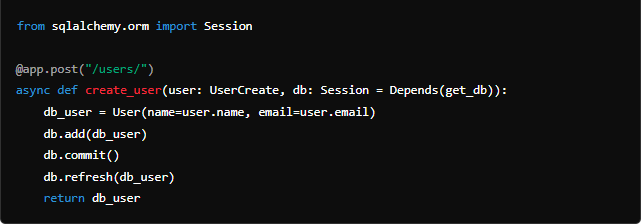
In this example, the create_user
endpoint adds a new user to the database using SQLAlchemy.
Using Tortoise ORM
Tortoise ORM is another popular ORM for Python, offering an asynchronous API that integrates well with FastAPI’s async capabilities.
Setting Up Tortoise ORM with FastAPI
To set up Tortoise ORM with FastAPI, you need to define your models and connect to the database.
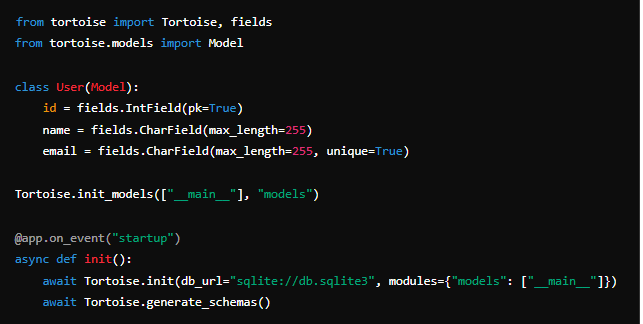
In this example, the User
class defines a model for the users
table. The Tortoise.init_models
function initializes the models, and the Tortoise.generate_schemas
function creates the database schema.
CRUD Operations with Tortoise ORM
Tortoise ORM allows you to perform CRUD operations using its async API.

In this example, the create_user
endpoint adds a new user to the database using Tortoise ORM.
API Versioning
API versioning is essential for maintaining backward compatibility as your API evolves. FastAPI provides several strategies for implementing versioning, allowing you to manage different versions of your API.
Path-Based Versioning
Path-based versioning involves including the version number in the URL path. This is the simplest and most common approach to versioning.
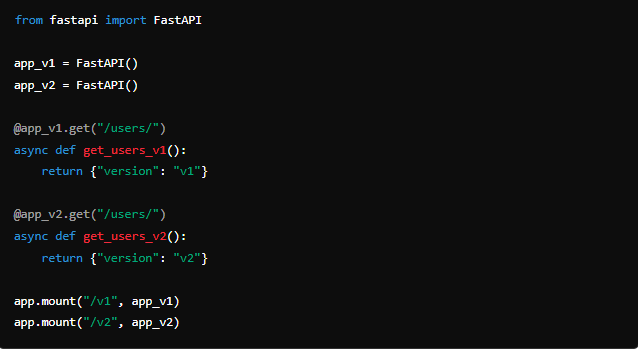
In this example, version 1 and version 2 of the get_users
endpoint are served under /v1/users/
and /v2/users/
, respectively.
Header-Based Versioning
Header-based versioning involves specifying the version number in the request headers. This approach is more flexible but requires clients to include the appropriate header.
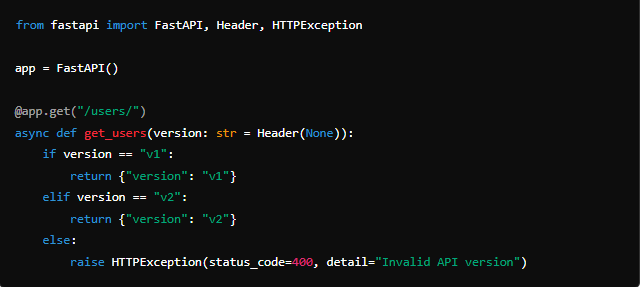
In this example, the version is specified in the X-API-Version
header, and the appropriate version of the get_users
endpoint is returned.
Query Parameter-Based Versioning
Query parameter-based versioning involves specifying the version number as a query parameter in the URL. This approach is similar to path-based versioning but keeps the versioning information out of the URL path.
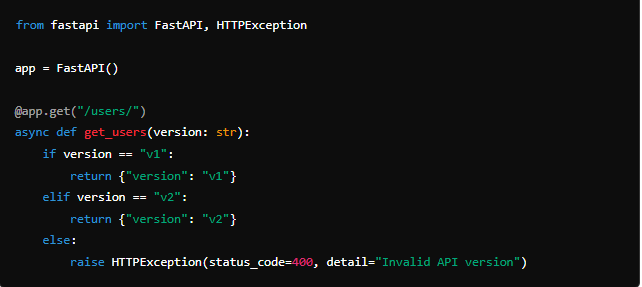
In this example, the version is specified as a query parameter, and the appropriate version of the get_users
endpoint is returned.
Combining Multiple Versioning Strategies
You can combine multiple versioning strategies to provide more flexibility for clients. For example, you can support both path-based and header-based versioning.
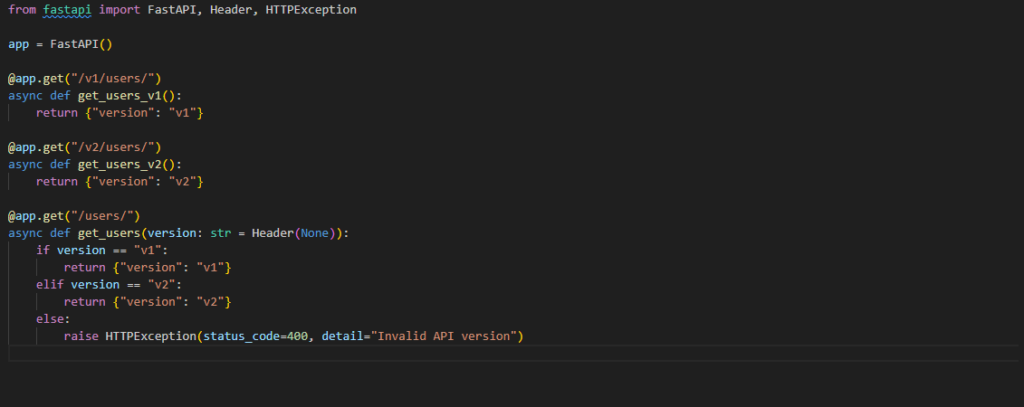
In this example, clients can choose between path-based versioning and header-based versioning based on their preferences.
FastAPI’s advanced features empower developers to build highly efficient and scalable applications. Whether you’re implementing async support, handling complex security requirements, or managing multiple API versions, FastAPI provides the tools you need to succeed. Understanding and leveraging these advanced features will allow you to create robust, maintainable, and future-proof applications that can grow with your needs.
5. Testing in FastAPI
Testing is a crucial aspect of developing robust and reliable web applications. FastAPI, with its asynchronous capabilities and built-in support for type validation, offers a seamless testing experience. This section will delve into the various testing strategies you can employ in FastAPI, covering unit testing, pytest integration, integration testing, testing asynchronous endpoints, and using TestClient.
Unit Testing in FastAPI
Unit testing is the process of testing individual components or functions in isolation. The primary goal is to ensure that each function or method behaves as expected under various conditions. In the context of FastAPI, unit testing often involves testing route handlers, services, or any other business logic.
Writing Unit Tests in FastAPI
To write unit tests in FastAPI, you should focus on isolating the function under test. This usually means avoiding dependencies on databases, external APIs, or other services. Instead, you can use mocking techniques to simulate these dependencies.
Example: Testing a Route Handler
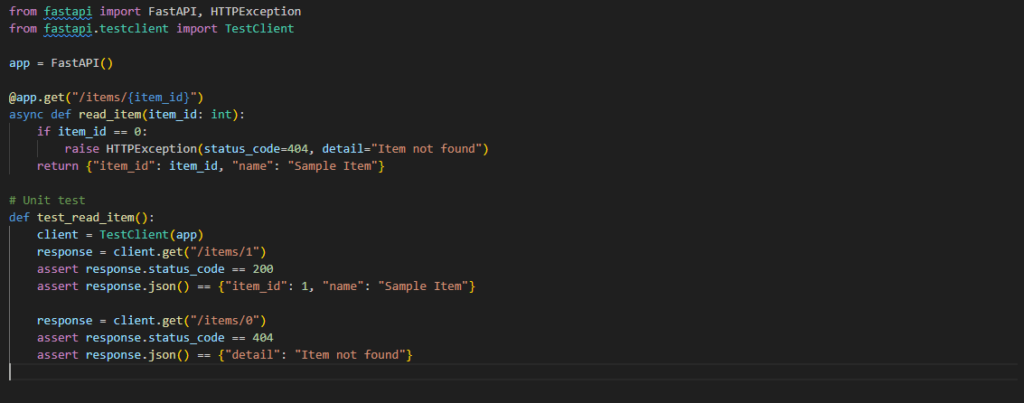
Mocking Dependencies
In real-world applications, your route handlers might depend on services like databases. In such cases, you can use mocking to isolate the unit being tested.
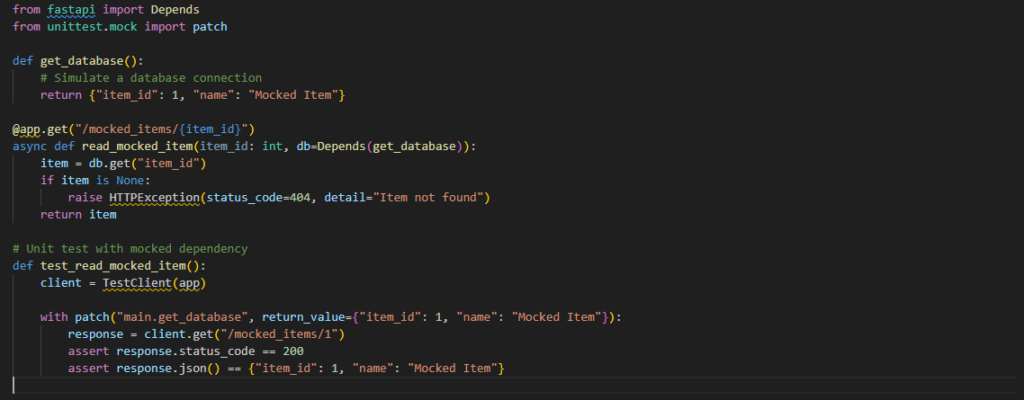
In this example, patch
is used to replace the get_database
function with a mocked version that returns a predefined item. This allows you to test the read_mocked_item
endpoint without requiring a real database connection.
Pytest with FastAPI
Pytest is a popular testing framework in Python that offers powerful features such as fixtures, parameterized testing, and plugins. FastAPI is fully compatible with pytest, making it an excellent choice for testing FastAPI applications.
Setting Up Pytest
To get started with pytest, you need to install it using pip install pytest.
Next, you can create a test file, typically named test_<module_name>.py
, and start writing your tests.
Example: Testing with Pytest
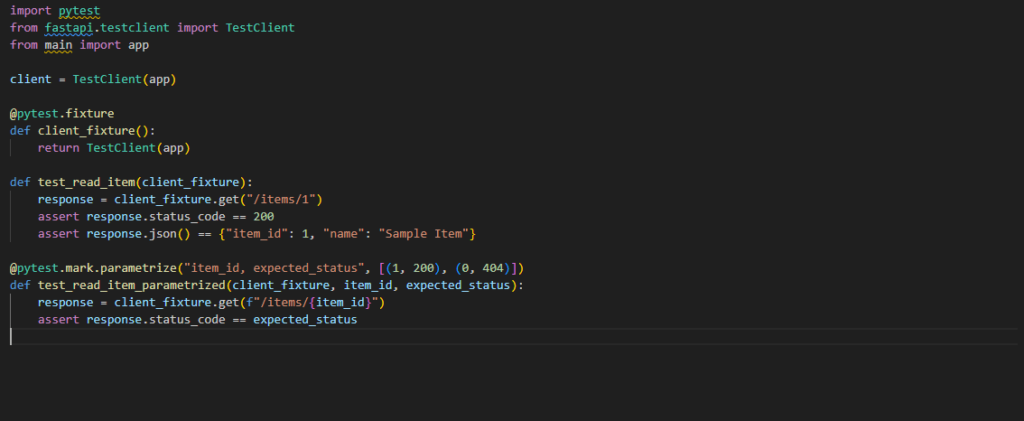
Using Fixtures
Fixtures in pytest are used to set up some context or state before the test runs. In the example above, the client_fixture
fixture initializes a TestClient
instance that can be reused across tests.
Parameterized Testing
Pytest allows you to run the same test with different parameters using @pytest.mark.parametrize
. This is useful when you want to test the same function with various inputs and expected outputs.
Integration Testing in FastAPI
Integration testing focuses on testing the interactions between different components or services in your application. In FastAPI, this might involve testing your application with a real database or interacting with external APIs.
Example: Integration Test with a Database
For integration testing, you often need a test database that mimics your production environment. FastAPI can be easily integrated with SQLAlchemy or other ORMs for this purpose.
Example: Testing with SQLAlchemy
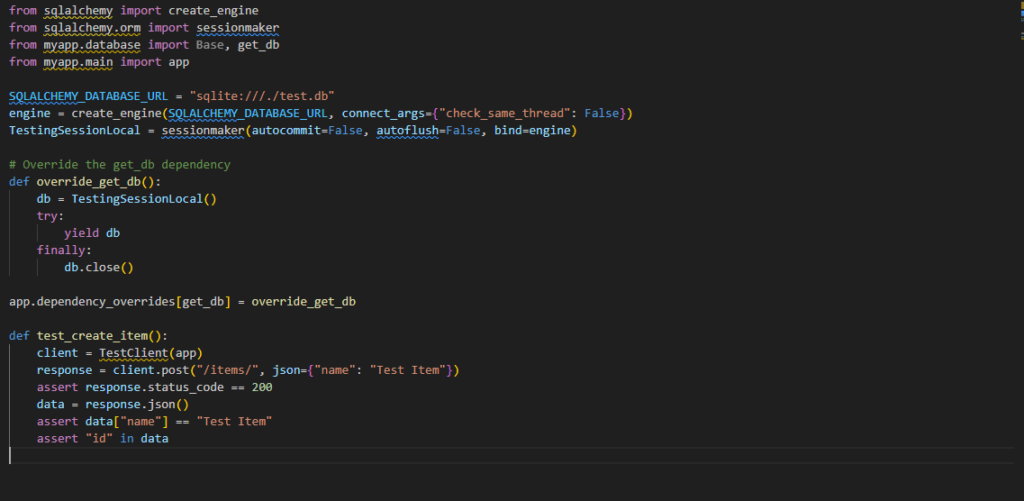
In this example, we create a test database using SQLite and override the get_db
dependency to use this test database. The test then verifies that an item can be created and retrieved from the database.
Testing with External APIs
If your application interacts with external APIs, you might want to mock these interactions during testing. Libraries like responses
or requests-mock
can be used to simulate external API responses.
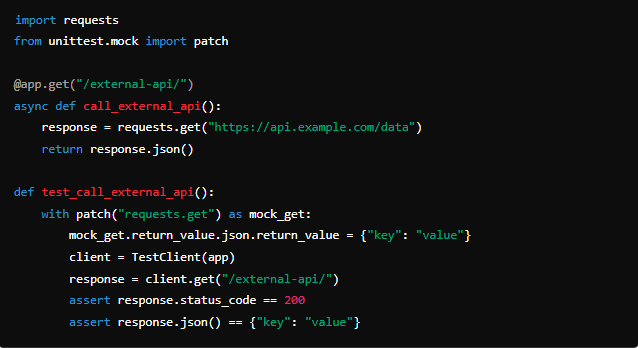
In this test, patch
is used to mock the requests.get
call to an external API, allowing you to simulate the API’s response without making a real HTTP request.
Testing Async Endpoints in FastAPI
FastAPI’s asynchronous capabilities allow you to handle a large number of requests efficiently. However, testing asynchronous endpoints requires a slightly different approach compared to synchronous ones.
Async Test Functions
When testing asynchronous endpoints, your test functions themselves need to be asynchronous. You can use the asyncio
library to run these tests.
Example: Testing an Async Endpoint
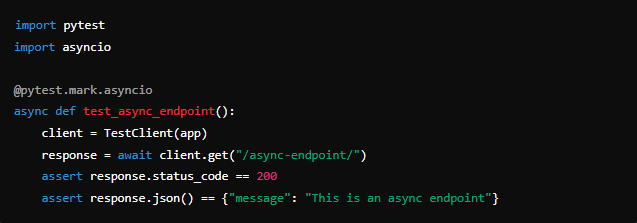
In this example, the @pytest.mark.asyncio
decorator is used to mark the test function as asynchronous. The test client can then be used to make asynchronous requests to the FastAPI app.
Using TestClient with Async Endpoints
The TestClient
in FastAPI is designed to handle both synchronous and asynchronous endpoints seamlessly. However, when dealing with complex asynchronous logic, you may need to leverage pytest-asyncio
or other async test frameworks.
Example: Advanced Async Testing
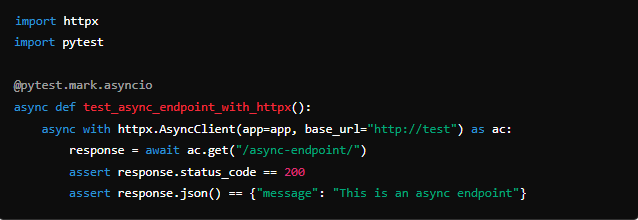
Here, httpx.AsyncClient
is used to make async requests to the FastAPI app. This approach can be particularly useful for more complex testing scenarios where you need finer control over async behavior.
Using TestClient in FastAPI
TestClient is a crucial tool in FastAPI testing. It allows you to send HTTP requests to your application as if it were running, but without actually starting a web server. This makes it ideal for both unit and integration testing.
Basic Usage of TestClient
TestClient is used to simulate HTTP requests to your FastAPI application. It can handle all HTTP methods like GET, POST, PUT, DELETE, etc.
Example: Basic TestClient Usage
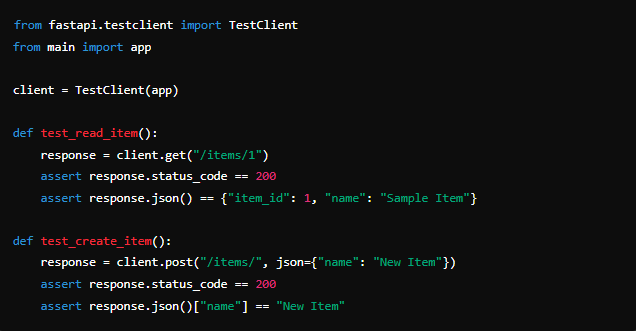
In this example, TestClient
is used to send GET and POST requests to the FastAPI app. The json
argument allows you to send JSON data with the request, and assert
statements are used to verify the response.
Advanced Usage with Custom Headers and Authentication
TestClient also supports sending custom headers, cookies, and handling authentication. This is particularly useful when your application requires JWT tokens or other authentication mechanisms.
Example: Test with Custom Headers
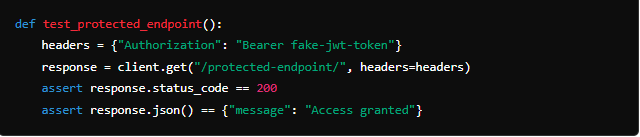
In this test, custom headers are passed to the TestClient
to simulate an authenticated request to a protected endpoint.
Testing Streaming Responses
FastAPI supports streaming responses, and you can use TestClient
to test these as well.
Example: Testing a Streaming Response
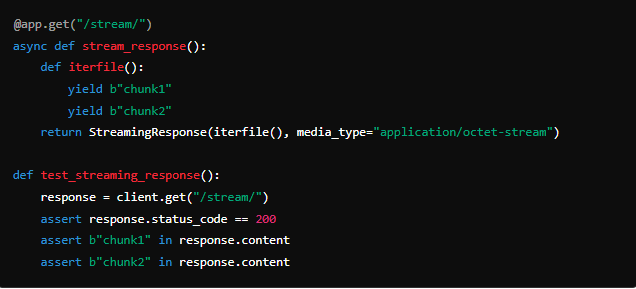
In this example, a streaming response is returned from the FastAPI endpoint, and TestClient
is used to verify the streamed content.
FastAPI’s testing capabilities, combined with tools like pytest
and TestClient
, provide a robust environment for ensuring your application is reliable and bug-free. Whether you’re writing unit tests, integration tests, or testing asynchronous endpoints, FastAPI’s design makes it straightforward to implement comprehensive testing strategies.
6. Documentation and API Schema
Automatic Interactive API Documentation
FastAPI’s automatic interactive API documentation is one of its standout features, making it incredibly easy for developers to explore and test their APIs directly from the browser. This feature is built on top of OpenAPI (formerly known as Swagger), which is a specification for building APIs that defines a standard, language-agnostic interface to RESTful APIs.
FastAPI automatically generates interactive API documentation based on the OpenAPI schema generated from your API code. This means that as you define your API endpoints, FastAPI will analyze the request and response models, including data types and validation rules, to create a corresponding OpenAPI schema. The automatic documentation is hosted directly within your application and can be accessed by simply navigating to specific URLs.
For instance, if you run your FastAPI application locally on http://127.0.0.1:8000
, you can access the interactive documentation at:
- Swagger UI:
http://127.0.0.1:8000/docs
- ReDoc:
http://127.0.0.1:8000/redoc
These UIs allow you to explore your API’s endpoints, view the expected inputs and outputs, and even test your endpoints by sending requests directly from the browser.
Swagger UI
Swagger UI is the default documentation interface provided by FastAPI. It offers a user-friendly, interactive interface for developers to explore and interact with their API.
- Exploring Endpoints: Swagger UI displays all the available endpoints in your API, along with the HTTP methods (GET, POST, PUT, DELETE, etc.) that can be used with each endpoint. Each endpoint is expandable, allowing developers to see details like request parameters, response formats, and status codes.
- Testing Endpoints: One of the most powerful features of Swagger UI is the ability to test your API endpoints directly from the interface. Each endpoint includes a “Try it out” button that allows you to send requests and view responses without leaving the documentation. This feature is particularly useful during development, as it allows you to quickly validate your API’s behavior.
- Detailed Response Information: Swagger UI provides detailed information about the responses your API returns. This includes not only the response data but also the status codes and any potential error messages. This helps developers understand how their API will behave in different scenarios.
- Customization: While Swagger UI is powerful out of the box, FastAPI allows you to customize its appearance and behavior. You can modify the title, description, version, and other metadata of your API, which will be reflected in the Swagger UI. Additionally, you can add custom tags to group related endpoints, making your documentation more organized.
ReDoc
ReDoc is an alternative documentation interface provided by FastAPI. It’s known for its clean, three-column layout and is particularly well-suited for large APIs with extensive documentation.
- Three-Column Layout: ReDoc organizes your API documentation into three columns: a navigation bar on the left, a content area in the center, and a detailed description pane on the right. This layout makes it easy to navigate through large APIs and provides a clear overview of the available endpoints.
- Collapsible Sections: ReDoc allows you to collapse and expand sections of your API documentation, making it easier to focus on specific parts of your API. This is especially useful for APIs with many endpoints, as it prevents the documentation from becoming overwhelming.
- Deep Linking: ReDoc supports deep linking, which means you can share direct links to specific sections or endpoints in your API documentation. This is useful for collaboration, as you can point others to the exact part of the documentation they need to see.
- Customizability: Similar to Swagger UI, ReDoc is highly customizable. You can modify the appearance and structure of the documentation to match your project’s branding or specific needs. For example, you can add custom logos, change the color scheme, and modify the layout to better fit your use case.
Customizing the OpenAPI Schema
FastAPI’s automatic generation of OpenAPI schemas is a major convenience, but there are times when you might want to customize this schema to better fit your needs. Customization options include modifying the API’s title, description, version, and more detailed aspects such as the structure of specific endpoints or models.
- Custom Titles and Descriptions: FastAPI allows you to easily customize the title, description, and version of your API directly in your code. This information is displayed prominently in the generated documentation and can be used to provide context and information about the API.
- Custom Path Operation IDs: By default, FastAPI generates operation IDs (unique identifiers for each endpoint) based on the function names of your view functions. However, you can customize these IDs to follow specific naming conventions or to make them more descriptive.
- Custom Tags: Tags in FastAPI are used to group related endpoints together. For example, you might group all user-related endpoints under a “Users” tag. These tags are reflected in the API documentation, making it easier to navigate and understand.
- Extending the OpenAPI Schema: In some cases, you might need to extend or modify the automatically generated OpenAPI schema. FastAPI allows you to do this by modifying the schema directly in your code. For example, you can add custom fields to your models, define additional security schemes, or modify the structure of specific endpoints.
Documenting Dependencies and Security
FastAPI’s dependency injection system is one of its core features, allowing you to define reusable components that can be injected into your endpoints. However, it’s equally important to document these dependencies and any security mechanisms you’ve implemented.
- Documenting Dependencies: Dependencies in FastAPI are typically used for tasks like database access, authentication, or data validation. These dependencies are automatically documented in the API schema, making it clear to developers what each endpoint requires. For example, if an endpoint requires an authenticated user, this requirement will be reflected in the documentation.
- Security Schemes: FastAPI supports multiple security schemes, such as OAuth2, JWT, and API keys. These schemes can be documented in your API schema, providing detailed information on how clients should authenticate their requests. FastAPI’s automatic documentation will include information on the security requirements for each endpoint, making it clear which endpoints are protected and how to access them.
- Customizing Security Documentation: While FastAPI automatically documents security requirements, you can customize this documentation to provide additional context or instructions. For example, you can add descriptions of different user roles, explain how to obtain API keys, or provide links to external authentication providers.
Versioning Documentation
As your API evolves, it’s important to version your documentation to ensure that users can access the correct information for the version of the API they are using. FastAPI provides several options for versioning your API and its documentation.
- Path-Based Versioning: One common approach to versioning in FastAPI is to include the version number in the path of your endpoints. For example, you might have
/v1/users
and/v2/users
for different versions of your user-related endpoints. This approach allows you to maintain separate documentation for each version of your API. - Custom Versioning Schemes: FastAPI also allows you to define custom versioning schemes, such as using query parameters or headers to specify the API version. This approach can be more flexible but requires careful documentation to ensure that users understand how to access different versions of the API.
- Maintaining Multiple Versions of Documentation: When you have multiple versions of your API, it’s important to maintain separate documentation for each version. FastAPI makes this easier by allowing you to define different OpenAPI schemas for different versions of your API. You can then serve different versions of the documentation at different URLs, such as
/v1/docs
and/v2/docs
. - Deprecation Notices: As you update your API, you may need to deprecate older versions or specific endpoints. FastAPI allows you to include deprecation notices in your documentation, informing users of upcoming changes and encouraging them to migrate to newer versions.
FastAPI’s robust documentation and API schema features provide developers with powerful tools for building and maintaining APIs. The automatic generation of interactive documentation through Swagger UI and ReDoc, combined with extensive customization options, makes it easy to create clear and comprehensive documentation. Additionally, FastAPI’s support for documenting dependencies, security, and versioning ensures that your API remains well-documented and accessible as it evolves.
7. Deploying FastAPI Applications
Deployment Strategies
Deploying FastAPI applications requires careful consideration of various factors to ensure optimal performance, scalability, and reliability. Depending on the use case, deployment strategies can vary from simple setups for small-scale applications to complex, multi-layered architectures for enterprise-grade systems.
- Local Deployment: For development and testing purposes, deploying FastAPI locally is the simplest approach. This involves running the FastAPI application directly on your development machine using
uvicorn
, the recommended ASGI server for FastAPI. While this is not suitable for production, it provides a quick way to test changes and iterate on the application. - Server Deployment: For production, you’ll typically deploy FastAPI on a server. This can be a virtual machine (VM) or a physical server where you install and configure all necessary components, including the FastAPI application, the web server (e.g., Nginx), and the application server (e.g., Gunicorn). Server deployment offers more control over the environment but requires manual management and maintenance.
- Containerization: Deploying FastAPI in containers using Docker has become increasingly popular. Containers encapsulate the application and its dependencies, ensuring consistency across different environments. Dockerization simplifies deployment and scaling, especially in cloud environments, where orchestration tools like Kubernetes can be used to manage containers at scale.
- Serverless Deployment: For certain use cases, deploying FastAPI as a serverless function can be an attractive option. Platforms like AWS Lambda, Google Cloud Functions, and Azure Functions allow you to deploy FastAPI in a serverless environment, where the cloud provider handles scaling and resource management. This approach reduces operational overhead but may introduce latency and cold start issues.
Dockerization
Dockerization involves packaging your FastAPI application into a Docker container, making it portable and easy to deploy across different environments. Docker ensures that the application runs consistently, regardless of where it is deployed.
Creating a Dockerfile: The first step in Dockerizing a FastAPI application is to create a Dockerfile
. This file contains a set of instructions for building a Docker image, including the base image to use, the dependencies to install, and the commands to run the application. A typical Dockerfile
for a FastAPI application might look like this:
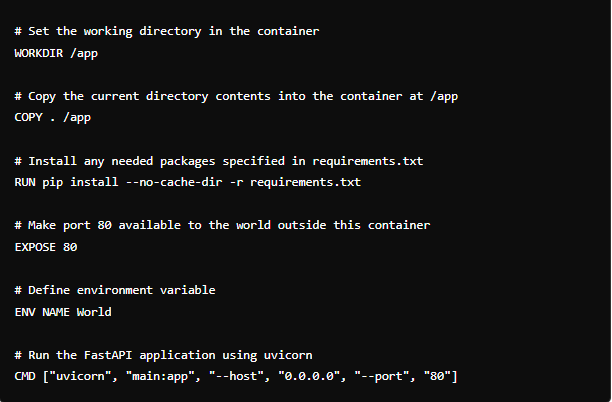
Building the Docker Image: Once the Dockerfile
is created, you can build the Docker image using the following command:

This command creates a Docker image with the tag my-fastapi-app
, which can be run as a container.
Running the Docker Container: After building the image, you can run the FastAPI application in a container using:

- This command runs the container in detached mode (
-d
), mapping port 80 of the host to port 80 of the container. - Optimizing Docker Images: To reduce the size of Docker images and improve performance, you can use multi-stage builds, which allow you to separate the build environment from the runtime environment. Additionally, using a slim version of the base image and minimizing the number of layers in the Dockerfile can further optimize the image.
Using Gunicorn with FastAPI
While uvicorn
is the recommended ASGI server for FastAPI, it is often used in conjunction with Gunicorn, a WSGI server, to handle production workloads. Gunicorn acts as a process manager that can spawn multiple worker processes, each running an instance of uvicorn
, thus improving the application’s ability to handle concurrent requests.
- Installing Gunicorn: You can install Gunicorn along with
uvicorn
using pip: pip install gunicorn uvicorn - Configuring Gunicorn: To run your FastAPI application with Gunicorn, you can use the following command: gunicorn -w 4 -k uvicorn.workers.UvicornWorker main:app
-w 4
specifies the number of worker processes to run.-k uvicorn.workers.UvicornWorker
tells Gunicorn to useuvicorn
as the worker class.- This setup allows Gunicorn to manage multiple
uvicorn
workers, providing better load balancing and fault tolerance.
- Optimizing Gunicorn Configuration: Tuning Gunicorn’s configuration is crucial for achieving optimal performance. This includes adjusting the number of workers based on the server’s CPU cores, setting appropriate timeouts to prevent hanging requests, and configuring logging for better observability.
Deployment on Cloud Platforms
Deploying FastAPI on cloud platforms offers scalability, reliability, and ease of management. Popular cloud providers like AWS, Google Cloud, and Azure offer various services that can be used to deploy and manage FastAPI applications.
- AWS (Amazon Web Services): AWS provides several options for deploying FastAPI applications, including Elastic Beanstalk, EC2, and Lambda.
- Elastic Beanstalk: AWS Elastic Beanstalk simplifies the deployment process by automatically handling the deployment, scaling, and monitoring of your application. You can deploy a FastAPI application on Elastic Beanstalk by uploading your Docker image or code bundle, and Elastic Beanstalk will manage the infrastructure for you.
- EC2: For more control over the environment, you can deploy FastAPI on an EC2 instance. This approach involves provisioning an EC2 instance, installing the necessary dependencies (e.g., Python, Gunicorn, Nginx), and deploying the FastAPI application manually or using automation tools like Ansible or Terraform.
- AWS Lambda: For serverless deployments, AWS Lambda can be used to run FastAPI applications. By using a framework like AWS Chalice or Zappa, you can deploy your FastAPI application as a Lambda function, with API Gateway handling the routing of HTTP requests.
- Google Cloud: Google Cloud offers several services for deploying FastAPI applications, including App Engine, Compute Engine, and Cloud Run.
- App Engine: Google App Engine is a fully managed platform that automatically handles scaling and load balancing. You can deploy a FastAPI application to App Engine by defining your application configuration in an
app.yaml
file and deploying using thegcloud
command. - Compute Engine: Similar to AWS EC2, Google Compute Engine provides VMs where you can deploy your FastAPI application. This approach offers more flexibility and control but requires manual management of the infrastructure.
- Cloud Run: Google Cloud Run is a serverless container platform that allows you to deploy FastAPI applications in Docker containers. Cloud Run automatically scales your application based on traffic, making it ideal for handling varying workloads.
- App Engine: Google App Engine is a fully managed platform that automatically handles scaling and load balancing. You can deploy a FastAPI application to App Engine by defining your application configuration in an
- Azure: Microsoft Azure provides several options for deploying FastAPI applications, including Azure App Service, Azure Functions, and Azure Kubernetes Service (AKS).
- Azure App Service: Azure App Service is a fully managed platform for deploying web applications. You can deploy a FastAPI application to Azure App Service by using the Azure CLI or the Azure portal, with support for both Docker containers and traditional code deployments.
- Azure Functions: For serverless deployments, Azure Functions allows you to run FastAPI applications with automatic scaling. By packaging your FastAPI application as a function app, you can deploy it using the Azure CLI or Visual Studio Code.
- Azure Kubernetes Service (AKS): For containerized deployments, Azure Kubernetes Service (AKS) provides a managed Kubernetes environment where you can deploy and manage FastAPI applications at scale. AKS simplifies the deployment and scaling of containerized applications, with support for advanced features like service mesh and monitoring.
CI/CD Pipelines for FastAPI
Continuous Integration (CI) and Continuous Deployment (CD) pipelines are essential for automating the deployment process, ensuring that your FastAPI application is consistently tested, built, and deployed with minimal manual intervention.
- CI/CD with GitHub Actions: GitHub Actions provides a powerful and flexible platform for building CI/CD pipelines directly in your GitHub repository. You can define workflows that automate the testing, building, and deployment of your FastAPI application using YAML configuration files.
- Testing: A typical CI pipeline starts with running tests to ensure that your FastAPI application is functioning correctly. You can use pytest to run unit and integration tests, with results automatically reported back to GitHub.
- Building Docker Images: After passing the tests, the pipeline can build a Docker image of your FastAPI application. This step involves defining a Dockerfile and using GitHub Actions to build the image and push it to a Docker registry like Docker Hub or GitHub Container Registry.
- Deployment: The final step in the CI/CD pipeline is deploying the Docker image to your chosen environment. This could involve deploying to a cloud platform like AWS, Google Cloud, or Azure, or to an on-premises server. GitHub Actions can automate this process using custom scripts or integrations with cloud provider services.
- CI/CD with GitLab CI/CD: GitLab CI/CD is another popular platform for building CI/CD pipelines. Similar to GitHub Actions, GitLab CI/CD allows you to define pipelines in YAML files, with support for stages like testing, building, and deploying.
- Pipeline Configuration: In GitLab CI/CD, you can define your pipeline in a
.gitlab-ci.yml
file. This file specifies the stages of the pipeline, the jobs to run in each stage, and the environments where the application should be deployed. - Environments and Deployments: GitLab CI/CD supports multiple environments, allowing you to deploy your FastAPI application to different stages like development, staging, and production. You can also use GitLab’s Kubernetes integration to deploy containerized applications to a Kubernetes cluster.
- Pipeline Configuration: In GitLab CI/CD, you can define your pipeline in a
- CI/CD with Jenkins: Jenkins is a widely used CI/CD tool that provides extensive flexibility and customization options. You can set up a Jenkins pipeline to automate the testing, building, and deployment of your FastAPI application.
- Pipeline as Code: Jenkins supports defining pipelines as code using Jenkinsfiles. A Jenkinsfile allows you to script the stages and steps of your CI/CD pipeline, with support for parallel execution, conditional steps, and notifications.
- Integration with Docker and Kubernetes: Jenkins integrates well with Docker and Kubernetes, making it a suitable choice for deploying containerized FastAPI applications. You can configure Jenkins to build Docker images, push them to a registry, and deploy them to a Kubernetes cluster.
Monitoring and Logging
Monitoring and logging are critical components of maintaining a FastAPI application in production. These practices help you track the application’s performance, detect issues early, and gain insights into how the application is being used.
- Monitoring Tools: There are several monitoring tools that you can integrate with FastAPI to track the application’s health and performance.
- Prometheus and Grafana: Prometheus is a popular open-source monitoring tool that collects metrics from your FastAPI application and stores them in a time-series database. You can then visualize these metrics using Grafana, which provides a powerful and customizable dashboard interface.
- New Relic: New Relic is a commercial application performance monitoring (APM) tool that provides deep insights into your FastAPI application’s performance, including response times, error rates, and throughput. New Relic also offers advanced features like distributed tracing and anomaly detection.
- Datadog: Datadog is another popular APM tool that provides real-time monitoring and analytics for your FastAPI application. Datadog integrates with various services and platforms, offering features like log management, synthetic monitoring, and network performance monitoring.
- Logging: Logging is essential for debugging and troubleshooting issues in your FastAPI application. By recording key events and errors, you can gain insights into what is happening within the application and identify the root cause of problems.
- Logging Configuration: FastAPI uses Python’s built-in logging module, which can be configured to log messages at different levels (e.g., DEBUG, INFO, WARNING, ERROR, CRITICAL). You can configure logging to output to the console, files, or external logging services.
- Structured Logging: Structured logging involves logging messages in a structured format (e.g., JSON), making it easier to parse and analyze logs. Tools like Logstash and Fluentd can be used to collect and process structured logs, while services like Elasticsearch and Splunk can store and index logs for searching and analysis.
- Centralized Logging: In distributed systems, centralized logging is crucial for aggregating logs from multiple sources and making them accessible from a single location. Services like AWS CloudWatch, Google Cloud Logging, and Azure Monitor provide centralized logging solutions that integrate with FastAPI applications.
7. Scaling FastAPI Applications
Scaling FastAPI applications involves increasing the application’s capacity to handle more requests and users. Scaling can be achieved through horizontal or vertical scaling, depending on the application’s requirements and infrastructure.
- Horizontal Scaling: Horizontal scaling involves adding more instances of the FastAPI application to handle increased traffic. This approach is generally preferred for cloud-native applications, as it allows for more flexible and resilient scaling.
- Load Balancing: When horizontally scaling a FastAPI application, a load balancer is used to distribute incoming requests across multiple instances. This ensures that no single instance is overwhelmed with traffic, improving the application’s overall performance and availability.
- Auto-Scaling: Cloud platforms like AWS, Google Cloud, and Azure offer auto-scaling services that automatically adjust the number of instances based on traffic and resource usage. Auto-scaling helps ensure that your FastAPI application can handle sudden spikes in traffic without manual intervention.
- Stateless Design: To effectively scale horizontally, your FastAPI application should be designed to be stateless. This means that each instance of the application should be able to handle any request independently, without relying on shared state. Techniques like using external databases or caching systems (e.g., Redis) can help achieve statelessness.
- Vertical Scaling: Vertical scaling involves increasing the resources (e.g., CPU, memory) of a single instance to handle more traffic. This approach is simpler to implement but has limitations, as there is a maximum limit to how much you can scale a single instance.
- Optimizing Resource Usage: When scaling vertically, it’s important to optimize the resource usage of your FastAPI application. This includes tuning the application’s memory and CPU usage, optimizing database queries, and reducing the overhead of external dependencies.
- Limits of Vertical Scaling: While vertical scaling can provide immediate benefits, it is generally less scalable in the long term compared to horizontal scaling. As traffic continues to grow, you may eventually need to transition to a horizontally scaled architecture to meet demand.
Deploying FastAPI applications involves a series of strategic decisions, from choosing the right deployment strategy to implementing effective monitoring, logging, and scaling practices. Whether deploying on cloud platforms, using containerization, or implementing CI/CD pipelines, each aspect plays a critical role in ensuring that your FastAPI application is robust, scalable, and maintainable in a production environment. By understanding these concepts in depth, you can optimize your FastAPI deployments to meet the specific needs of your project and organization.
8. Performance Optimization: Introduction
Performance optimization is a critical aspect of building and maintaining a robust FastAPI application. As web applications grow in complexity and scale, ensuring they perform efficiently under varying loads becomes paramount. Performance optimization involves identifying and addressing bottlenecks, improving response times, and maximizing resource utilization to deliver a seamless user experience.
In the context of FastAPI, performance optimization can be approached from multiple angles—benchmarking to establish performance baselines, optimizing database queries to reduce latency, implementing effective caching strategies, leveraging concurrency and parallelism for better task management, and conducting load testing to assess scalability. Each of these areas plays a vital role in enhancing the overall performance of your FastAPI application, ensuring it can handle real-world demands efficiently.
This section will delve into the key strategies and best practices for optimizing FastAPI’s performance. By understanding and applying these techniques, you can significantly improve your application’s speed, reliability, and capacity to handle increased traffic, making it more resilient and user-friendly.
Benchmarking FastAPI
Introduction to Benchmarking: Benchmarking is the process of measuring the performance of an application by running a set of standardized tests. In the context of FastAPI, benchmarking involves assessing various aspects such as response time, throughput, and resource utilization under different conditions. The goal is to understand how well FastAPI performs under load and identify potential bottlenecks.
Tools for Benchmarking:
- Locust: A popular load-testing tool that simulates multiple users accessing the API. It allows you to define user behavior and see how the API performs under stress.
- Apache Benchmark (ab): A command-line tool for measuring the performance of your web server. It’s useful for simple, quick benchmarks.
- JMeter: A comprehensive tool that allows for complex load testing and performance measurement, including distributed testing capabilities.
- Artillery: A modern, powerful, and easy-to-use load-testing tool for testing the performance of APIs.
Setting Up Benchmarks:
- Define the performance metrics you want to measure, such as response time, requests per second, CPU usage, and memory usage.
- Establish baseline performance by running benchmarks under minimal load.
- Gradually increase the load to simulate real-world usage and identify how the system behaves as traffic increases.
- Record the results, paying close attention to metrics like latency, throughput, and error rates.
Interpreting Benchmark Results:
- Latency: Low latency indicates quick response times, which is crucial for API performance. Aim for consistent latency across different loads.
- Throughput: This is the number of requests your FastAPI application can handle per second. Higher throughput is generally better, but it must be balanced with acceptable latency.
- Resource Utilization: Monitor CPU and memory usage to ensure your application is not overusing resources, which can lead to degradation in performance.
Common Benchmarking Pitfalls:
- Not simulating realistic workloads: Ensure your benchmarks mimic actual usage scenarios, including different types of requests and user behaviors.
- Ignoring cold starts: FastAPI’s performance may vary during initial requests after deployment, known as a cold start. Include these in your benchmarks.
- Overlooking network conditions: Consider network latency and bandwidth, especially if your API will be accessed over the internet.
Optimizing Database Queries
Introduction to Database Query Optimization: Database queries are often the most significant performance bottleneck in web applications. Optimizing these queries can drastically improve the performance of your FastAPI application. This involves writing efficient SQL queries, reducing unnecessary database hits, and leveraging database indexing.
Key Strategies for Optimizing Database Queries:
- Indexing: Properly indexing your database tables can speed up query execution. Identify frequently used columns in
WHERE
,JOIN
, andORDER BY
clauses and add indexes accordingly. - Query Profiling: Use tools like
EXPLAIN
in SQL to analyze query performance and identify slow queries. This helps you understand how the database executes your query and where it might be optimized. - Reducing Query Complexity: Avoid complex queries that involve multiple joins or subqueries. Simplify where possible or consider breaking complex queries into smaller, more manageable ones.
- Caching Database Results: For frequently accessed data that doesn’t change often, consider caching the results of database queries to reduce the load on the database.
- Avoiding N+1 Query Problem: When fetching related data, ensure you’re not executing multiple queries where one would suffice. Use efficient ORM methods or raw SQL to avoid the N+1 problem.
- Pagination and Limiting Results: When dealing with large datasets, use pagination to limit the number of records returned in a single query. This reduces load time and resource consumption.
ORM (Object-Relational Mapping) Optimization:
- Lazy vs. Eager Loading: In FastAPI, using SQLAlchemy or Tortoise ORM, decide between lazy and eager loading based on your use case. Lazy loading delays loading related data until it’s accessed, while eager loading fetches everything upfront.
- Bulk Operations: For inserting or updating large datasets, use bulk operations instead of individual inserts or updates. This reduces the number of database round-trips.
- Session Management: Ensure efficient session management in your ORM. Keep sessions short-lived and avoid keeping them open longer than necessary to prevent locking issues.
Database Connection Pooling:
- Connection Pooling: Use connection pooling to manage database connections efficiently. Tools like SQLAlchemy’s connection pool can help manage a pool of reusable connections, reducing the overhead of creating and closing connections for each request.
- Adjusting Pool Size: Optimize the pool size based on your application’s concurrency requirements. Too few connections can lead to bottlenecks, while too many can overwhelm the database server.
Caching Strategies
Introduction to Caching: Caching is the process of storing frequently accessed data in a fast storage layer (cache) so that future requests can be served quicker without hitting the database or recomputing results. This significantly reduces latency and improves the throughput of your FastAPI application.
Types of Caching:
- In-Memory Caching: Storing data in memory using tools like Redis or Memcached. This is ideal for data that needs to be accessed quickly and changes frequently.
- HTTP Caching: Leveraging HTTP headers like
Cache-Control
andETag
to cache responses at the client or CDN level, reducing the load on your server. - Database Query Caching: Caching the results of expensive database queries to avoid repeated execution.
Implementing Caching in FastAPI:
- FastAPI Middleware: Use middleware to intercept requests and responses for caching purposes. Custom middleware can be developed to handle complex caching logic.
- Third-Party Libraries: Integrate libraries like
aiocache
for asynchronous caching orcachetools
for simple in-memory caching. - Caching with Redis: Redis is a popular choice for caching in FastAPI applications. It supports various data structures and allows for complex caching scenarios like time-based expiration and invalidation.
Cache Invalidation:
- Time-Based Invalidation: Set a time-to-live (TTL) for cached items to ensure stale data is automatically removed.
- Event-Driven Invalidation: Invalidate cache based on specific events, such as data updates or user actions, ensuring the cache always returns fresh data.
- Manual Invalidation: Provide mechanisms to manually invalidate cache entries when necessary, such as through admin interfaces or API endpoints.
Caching Best Practices:
- Cache Granularity: Determine the granularity of caching, whether it’s caching entire responses, specific sections, or database query results.
- Avoiding Cache Staleness: Implement strategies to detect and prevent serving stale data from the cache.
- Monitoring Cache Performance: Regularly monitor cache hit/miss ratios and adjust your caching strategy accordingly to maximize efficiency.
Concurrency and Parallelism
Concurrency vs. Parallelism:
- Concurrency involves handling multiple tasks simultaneously but not necessarily executing them at the same time. It’s about efficiently managing tasks that might be waiting for I/O operations.
- Parallelism involves executing multiple tasks at the same time, utilizing multiple CPU cores for true parallel execution.
Concurrency in FastAPI:
- Asynchronous I/O: FastAPI is built on top of Starlette, which uses asynchronous I/O operations powered by Python’s
async
andawait
keywords. This allows FastAPI to handle a large number of concurrent connections efficiently. - Asynchronous Tasks: Use asynchronous tasks for I/O-bound operations like database queries, network requests, or file handling to keep the main thread free to handle other requests.
- Task Queues: For long-running tasks, consider using task queues like Celery or
backgroundtasks
in FastAPI, which allows tasks to be processed in the background without blocking the main event loop.
Parallelism in FastAPI:
- Process Pooling: For CPU-bound tasks that require significant computation, use process pooling with Python’s
multiprocessing
library. This allows tasks to be distributed across multiple CPU cores. - Thread Pooling: For tasks that involve blocking I/O operations, thread pooling can be used to improve performance. However, threading in Python can be limited by the Global Interpreter Lock (GIL).
Optimizing Concurrency in FastAPI:
- Efficient Use of
async
/await
: Ensure that I/O-bound operations are marked withasync
andawait
to free up the event loop. Avoid using blocking calls within asynchronous functions. - Handling High Concurrency: Adjust FastAPI’s server settings, such as the number of worker processes and threads, based on your application’s concurrency requirements.
- Balancing Load: Use load balancers in front of your FastAPI application to distribute incoming requests evenly across multiple instances or servers.
Load Testing
Introduction to Load Testing: Load testing involves simulating real-world traffic to understand how your FastAPI application performs under different loads. The goal is to identify performance bottlenecks, assess scalability, and ensure the application can handle expected traffic.
Tools for Load Testing:
- Locust: A user-friendly load testing tool that allows you to simulate millions of users making requests to your FastAPI application.
- Artillery: A modern load-testing toolkit suitable for testing the performance of APIs and services at scale.
- JMeter: A comprehensive tool for load testing, allowing you to simulate various types of loads and analyze the results.
Designing Load Tests:
- Realistic Scenarios: Design load tests that mimic real-world usage patterns, including peak traffic periods and different types of requests (e.g., GET, POST, PUT).
- Ramp-Up Period: Gradually increase the load on your application during the test to identify at what point performance starts to degrade.
- Steady-State Testing: Maintain a constant load for an extended period to assess how the application performs under sustained traffic.
Analyzing Load Test Results:
- Throughput and Latency: Measure the number of requests your application can handle per second and the response time for each request. High throughput with low latency indicates good performance.
- Error Rates: Monitor the number of errors or failed requests during load testing. A spike in error rates could indicate that your application is struggling under the load.
- Resource Utilization: Track CPU, memory, and network usage to ensure your application is using resources efficiently. High resource usage with declining performance may require optimization.
Improving Load Handling:
- Horizontal Scaling: Deploy additional instances of your FastAPI application to distribute the load. This can be achieved through container orchestration platforms like Kubernetes.
- Vertical Scaling: Increase the resources (CPU, memory) allocated to your application server to handle more load. This is useful if your application is limited by resource constraints.
- Circuit Breakers: Implement circuit breakers to prevent system overloads. Circuit breakers monitor the health of the application and stop accepting requests when a failure threshold is reached.
- Rate Limiting: Implement rate limiting to control the number of requests a user or client can make in a given period. This helps prevent abuse and ensures fair usage of resources.
Best Practices in FastAPI Development
Building a FastAPI application involves more than just writing code that works; it requires a thoughtful approach to ensure the code is organized, secure, maintainable, and scalable. This section explores the best practices to follow when developing FastAPI applications, covering everything from project structure to security measures, input validation, API design, and code maintenance.
Code Organization and Project Structure
Importance of Proper Code Organization: A well-organized codebase is crucial for maintainability, scalability, and collaboration. As your FastAPI application grows, keeping the codebase organized helps in understanding, debugging, and extending the application. It also makes it easier for new developers to onboard and contribute effectively.
Recommended Project Structure: While FastAPI doesn’t enforce a specific project structure, adopting a consistent and logical structure is beneficial. Here’s a common structure for FastAPI projects:
project_name/
│
├── app/
│ ├── __init__.py # Initialize the application package
│ ├── main.py # Entry point for the FastAPI application
│ ├── config.py # Configuration settings
│ ├── models/ # Database models
│ │ ├── __init__.py
│ │ ├── user.py
│ │ └── item.py
│ ├── routers/ # Routers for different API endpoints
│ │ ├── __init__.py
│ │ ├── user.py
│ │ └── item.py
│ ├── schemas/ # Pydantic models for request/response validation
│ │ ├── __init__.py
│ │ ├── user.py
│ │ └── item.py
│ ├── services/ # Business logic and service layers
│ │ ├── __init__.py
│ │ ├── user_service.py
│ │ └── item_service.py
│ ├── dependencies.py # Dependency injection
│ ├── exceptions.py # Custom exception handlers
│ └── utils.py # Utility functions
│
├── tests/ # Test cases
│ ├── __init__.py
│ ├── test_user.py
│ └── test_item.py
│
├── .env # Environment variables
├── requirements.txt # Python dependencies
├── Dockerfile # Docker configuration
└── README.md # Project documentation
Explanation of Key Components:
main.py
: The entry point for the application where the FastAPI instance is created and routes are included.config.py
: Holds configuration settings like database URLs, secret keys, and environment-specific configurations.models/
: Contains SQLAlchemy models or other ORM models representing database tables.routers/
: Organized by functionality, each router file contains related API endpoints.schemas/
: Contains Pydantic models used for input and output validation, ensuring data integrity.services/
: The business logic layer, separating complex operations from the endpoints, making the codebase cleaner and easier to test.dependencies.py
: Centralizes dependency injection, promoting code reuse and simplifying testing.exceptions.py
: Defines custom exception handlers to provide meaningful error messages and responses.
Benefits of a Modular Structure:
- Separation of Concerns: Each module is responsible for a specific part of the application, making it easier to understand and modify.
- Reusability: Code organized into services, utilities, and schemas can be reused across different parts of the application.
- Testability: A modular structure makes it easier to isolate and test individual components.
Security Best Practices
Securing FastAPI Applications: Security is a critical concern in web development, and FastAPI provides several features to help you build secure applications. Following security best practices protects your application from common vulnerabilities and ensures the integrity and confidentiality of user data.
Key Security Measures:
- Use HTTPS: Always use HTTPS to encrypt data in transit, preventing man-in-the-middle attacks. Tools like Let’s Encrypt can help you obtain and manage SSL certificates for your FastAPI application.
- Environment Variables for Sensitive Information: Store sensitive information like database credentials, API keys, and secret tokens in environment variables. This keeps them out of the codebase and reduces the risk of exposure.
- Security Headers: Implement HTTP security headers like
Content-Security-Policy
,X-Content-Type-Options
,Strict-Transport-Security
, andX-Frame-Options
to protect against various web vulnerabilities. - OAuth2 and JWT for Authentication: Use OAuth2 and JWT (JSON Web Tokens) for secure user authentication and authorization. FastAPI provides built-in support for these methods, enabling you to implement secure authentication mechanisms.
- Rate Limiting: Implement rate limiting to protect your API from brute-force attacks and abuse. This limits the number of requests a client can make in a given period.
Database Security:
- SQL Injection Prevention: Always use parameterized queries to prevent SQL injection attacks. ORMs like SQLAlchemy provide mechanisms to safely construct SQL queries, mitigating this risk.
- Database Encryption: Use database-level encryption to protect sensitive data at rest. This ensures that even if the database is compromised, the data remains secure.
API Security:
- Input Validation: Validate all incoming data to prevent malicious inputs from compromising your application. Use Pydantic models to enforce data validation rules.
- Cross-Origin Resource Sharing (CORS): Properly configure CORS to control which domains can access your API. Misconfigured CORS policies can expose your API to security risks.
Input Validation
Importance of Input Validation: Input validation ensures that the data received by your application is safe, correct, and expected. It’s a fundamental security practice that helps prevent various types of attacks, including injection attacks, buffer overflows, and malformed data exploits.
Using Pydantic for Validation: FastAPI leverages Pydantic to validate incoming data, making it easy to enforce data integrity. Pydantic models allow you to define the structure, types, and constraints of the data your API expects.
Key Validation Techniques:
- Type Validation: Ensure that the data types match what is expected. For example, an integer field should not accept a string value.
- Range Validation: Use Pydantic’s
constr
,conint
,conlist
, and other constraints to enforce limits on the size, length, and range of data. - Format Validation: Validate that fields like email addresses, URLs, and phone numbers conform to the correct formats using regular expressions or built-in validators.
- Custom Validators: Implement custom validators in Pydantic models to enforce complex validation rules, such as checking that a date is not in the past.
Error Handling in Validation:
- Descriptive Error Messages: Ensure that validation errors provide clear and descriptive messages, helping users understand what went wrong and how to fix it.
- Global Validation: Implement global validation logic for common data patterns (e.g., IDs, usernames) to maintain consistency across the application.
Avoiding Common Security Pitfalls
Common Security Pitfalls in FastAPI: Despite best efforts, developers often encounter common security pitfalls that can compromise the security of a FastAPI application. Being aware of these pitfalls and how to avoid them is crucial.
SQL Injection:
- Cause: Occurs when user input is improperly sanitized, allowing attackers to inject malicious SQL code.
- Prevention: Always use parameterized queries or ORM methods that safely handle user input. Avoid concatenating user input directly into SQL queries.
Cross-Site Scripting (XSS):
- Cause: Occurs when an attacker injects malicious scripts into web pages viewed by other users.
- Prevention: Sanitize user input that is rendered in the browser. Use template engines that escape HTML by default and implement content security policies.
Cross-Site Request Forgery (CSRF):
- Cause: Occurs when an attacker tricks a user into performing actions on a web application where they are authenticated.
- Prevention: Implement CSRF tokens in forms and require them for state-changing operations. Use session-based authentication methods that include CSRF protection.
Insecure Deserialization:
- Cause: Occurs when untrusted data is used to instantiate objects, potentially leading to arbitrary code execution.
- Prevention: Avoid deserializing data from untrusted sources. Use libraries that enforce strict validation on serialized data formats.
Sensitive Data Exposure:
- Cause: Occurs when sensitive data such as passwords, credit card information, or personal identifiers are exposed to unauthorized users.
- Prevention: Encrypt sensitive data at rest and in transit. Use environment variables to manage sensitive configurations and audit access to sensitive endpoints.
API Design Best Practices
Use of HTTP Status Codes:
- Success Codes: Use
200 OK
for successful GET requests,201 Created
for successful POST requests that create resources, and204 No Content
for successful DELETE requests. - Client Error Codes: Use
400 Bad Request
for invalid input,401 Unauthorized
for authentication failures,403 Forbidden
for permission issues, and404 Not Found
when a resource doesn’t exist. - Server Error Codes: Use
500 Internal Server Error
for unexpected server issues,502 Bad Gateway
when the server is acting as a gateway and cannot receive a response, and503 Service Unavailable
for temporary outages.
Versioning:
- API Versioning: Implement versioning in your API to manage breaking changes. This can be done using URL path versioning (
/v1/users
) or header versioning. Versioning ensures backward compatibility and smooth transitions for clients when updates occur.
Consistent Naming Conventions:
- Endpoint Naming: Use clear and consistent naming conventions for endpoints. Stick to plural nouns (
/users
,/items
) and avoid using verbs in endpoint names, as HTTP methods already define actions. - Field Naming: Follow consistent conventions for field names across all resources. For example, use
created_at
andupdated_at
for timestamps, andid
for resource identifiers.
Pagination:
- Handling Large Datasets: Implement pagination for endpoints that return large datasets. Use query parameters like
?page=1&size=20
to control the number of results returned and provide links to next and previous pages in the response. - Cursor-Based Pagination: Consider using cursor-based pagination for more efficient data retrieval, especially in high-throughput systems. This method is more scalable for APIs with large datasets.
Error Handling:
- Unified Error Responses: Standardize error responses across your API, providing a consistent structure that includes an error code, a message, and possibly a link to documentation.
- Descriptive Errors: Ensure that error messages are clear and informative, helping developers understand what went wrong and how to resolve the issue.
Documentation:
- Auto-Generated Documentation: Leverage FastAPI’s ability to auto-generate interactive API documentation with Swagger UI or ReDoc. This feature is invaluable for developers and users to explore the API and understand how to interact with it.
- Custom Documentation: Supplement auto-generated docs with custom documentation for complex use cases, examples, and best practices. This helps users implement your API effectively.
Version Control and Documentation
Importance of Version Control: Version control is essential for tracking changes, collaborating with other developers, and maintaining a history of the codebase. It allows you to manage different versions of your application, roll back to previous states if necessary, and experiment with new features without affecting the main codebase.
Best Practices in Version Control:
- Use Git: Git is the most widely used version control system, and tools like GitHub, GitLab, or Bitbucket provide platforms for hosting and collaborating on repositories.
- Branching Strategy: Adopt a branching strategy like Git Flow, where you have a
main
branch for production, adevelop
branch for staging, and feature branches for individual features or bug fixes. This strategy helps in organizing work and managing releases. - Commit Messages: Write clear and concise commit messages that describe the changes made. Follow a consistent format, such as including the issue number (
#123
), a short description, and any relevant details.
Pull Requests and Code Reviews:
- Pull Requests (PRs): Use pull requests to propose changes to the codebase. PRs allow team members to review, discuss, and approve changes before they are merged.
- Code Reviews: Conduct thorough code reviews to catch bugs, ensure code quality, and share knowledge among team members. Encourage constructive feedback and focus on both functionality and style.
Documentation:
- Internal Documentation: Maintain internal documentation for developers, including setup guides, architecture diagrams, coding standards, and troubleshooting tips. This documentation is essential for onboarding new developers and ensuring consistency across the team.
- API Documentation: Ensure that your API documentation is comprehensive and up-to-date. Use tools like Sphinx or MkDocs for generating static documentation from your codebase.
- Versioned Documentation: If your API has multiple versions, maintain separate documentation for each version. This helps users who are still on older versions while guiding them toward upgrading.
Using Linters and Formatters
Code Quality Tools: Maintaining high code quality is essential for ensuring that your application is reliable, maintainable, and free from common errors. Linters and formatters are tools that help enforce coding standards and catch issues early in the development process.
Linters:
- What is a Linter? A linter is a tool that analyzes your code for potential errors, stylistic issues, and deviations from coding standards. It helps maintain consistency and avoid common mistakes.
- Popular Linters for Python: Use
flake8
,pylint
, ormypy
to enforce coding standards and catch errors in your FastAPI project. Each of these tools can be configured to match your team’s coding style and requirements. - Continuous Integration: Integrate linters into your CI/CD pipeline to automatically check code quality whenever changes are pushed. This ensures that code quality is consistently maintained across the project.
Formatters:
- What is a Formatter? A formatter automatically reformats your code to adhere to a consistent style. This reduces the need for manual formatting and helps maintain readability.
- Black: Use
Black
, a popular Python formatter, to automatically format your FastAPI codebase. Black enforces a consistent style, making the codebase more readable and easier to work with. - Prettier: For projects that involve frontend code or JSON files, consider using
Prettier
, which formats code in multiple languages, ensuring consistency across your entire project.
Benefits of Using Linters and Formatters:
- Consistency: Enforcing a consistent style across the codebase makes it easier to read and maintain, especially in large teams.
- Error Prevention: Linters catch potential issues before they become bugs, reducing the likelihood of runtime errors.
- Code Reviews: Clean and consistently formatted code makes code reviews faster and more focused on logic and functionality rather than style issues.
Maintaining Clean and Readable Code
Importance of Code Readability: Clean and readable code is essential for maintainability, collaboration, and reducing technical debt. Readable code is easier to understand, modify, and debug, which is crucial as the project evolves over time.
Best Practices for Clean Code:
- Follow the Zen of Python: Adhere to Python’s guiding principles, as outlined in the Zen of Python (PEP 20). Principles like “Simple is better than complex” and “Readability counts” should guide your coding practices.
- Meaningful Names: Use descriptive and meaningful names for variables, functions, classes, and modules. Avoid single-letter names except in cases like loop counters, and prefer names that clearly convey the purpose of the code.
- Modularization: Break down large functions and classes into smaller, modular components. Each function should have a single responsibility, making it easier to test and maintain.
- Avoid Magic Numbers: Replace magic numbers (hard-coded values) with named constants. This makes the code more understandable and easier to update.
- Commenting and Documentation: Write comments where necessary to explain complex logic, but avoid over-commenting. Use docstrings to document the purpose and usage of functions and classes.
Refactoring:
- Continuous Refactoring: Regularly refactor code to improve its structure, readability, and efficiency. Refactoring should be an ongoing process, not a one-time task.
- Test-Driven Development (TDD): Adopt TDD to ensure that your code is thoroughly tested and that refactoring does not introduce new bugs. TDD encourages writing tests before writing the implementation, leading to better code design.
Avoiding Code Smells:
- Code Smells: Be aware of common code smells like duplicated code, long methods, and large classes. These are indicators of potential problems that should be addressed through refactoring.
- Eliminate Dead Code: Regularly remove unused code, functions, and imports. Dead code increases the complexity of the codebase and can lead to confusion and errors.
Conclusion
Summary of Key Points
FastAPI has emerged as a powerful, high-performance framework for building modern web applications and APIs. Its design philosophy emphasizes speed, efficiency, and ease of use, making it a compelling choice for developers. Key points discussed include:
- Performance and Asynchronous Capabilities: FastAPI’s asynchronous support and performance optimizations set it apart from other frameworks. Its ability to handle high loads and concurrent requests efficiently makes it ideal for scalable applications.
- Automatic Documentation: The integration with OpenAPI and automatic generation of interactive API documentation through Swagger UI and ReDoc simplifies development and improves usability.
- Type Safety and Validation: FastAPI leverages Python’s type hints and Pydantic for robust data validation and type safety, enhancing code reliability and reducing runtime errors.
- Rich Ecosystem: The framework benefits from a rich ecosystem of libraries and tools that integrate seamlessly, extending its functionality and making it versatile for various use cases.
- Community and Support: FastAPI’s active community contributes to its growth, providing valuable resources, support, and contributions that drive ongoing improvements.
- Future Prospects: The framework’s roadmap and emerging trends suggest continued evolution with enhanced features, better integration with modern technologies, and a growing role within the Python ecosystem.
Why Choose FastAPI for Your Next Project?
Choosing FastAPI for your next project offers several advantages:
- Exceptional Performance: With its high speed and efficient handling of asynchronous operations, FastAPI is well-suited for performance-critical applications.
- Developer Productivity: FastAPI’s automatic documentation, type checking, and clear API design improve developer productivity and reduce the time needed for development and maintenance.
- Scalability: The framework’s support for asynchronous operations and integration with modern tools makes it a strong choice for scalable and high-load applications.
- Ease of Use: The simplicity and ease of use, combined with comprehensive documentation and community support, make FastAPI accessible for both new and experienced developers.
- Future-Proofing: FastAPI’s alignment with modern Python features and its active development ensure that it will continue to be a relevant and powerful tool for future projects.
Final Thoughts
FastAPI represents a significant advancement in web development frameworks, bringing together performance, ease of use, and modern programming practices. Its adoption is likely to grow as more developers and organizations recognize its benefits. Whether you are building RESTful APIs, microservices, or real-time applications, FastAPI offers a robust and efficient solution that aligns well with contemporary development needs. Embracing FastAPI can lead to faster development cycles, better performance, and a more enjoyable coding experience.
References
Official Documentation
- FastAPI Official Documentation: The primary source for comprehensive information about FastAPI, including installation, usage, and advanced features.
Books, Articles, and Tutorials
- Books:
- “FastAPI Documentation” by Tiangolo: Offers a detailed guide on using FastAPI and its features.
- Tutorials:
- Real Python’s FastAPI Tutorial: A comprehensive tutorial on building APIs with FastAPI.
- FastAPI Crash Course by Traversy Media: A video tutorial providing a quick start with FastAPI.